Is there a simple trick to take the signed 32bit count
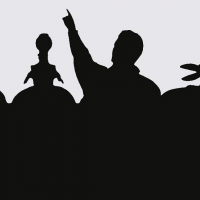
and extend it to signed 64bit?
I can think of a few really clunky ways to achieve this but I wouldn't be surprised to learn that there is a standard (simple) technique.
Craig
and extend it to signed 64bit?
I can think of a few really clunky ways to achieve this but I wouldn't be surprised to learn that there is a standard (simple) technique.
Craig
Comments
Which count do you mean? If you're referring to the standard system clock counter, the full 64 bit value may be read via _getcnthl():
@ersmith
Oops, my bad. Forgot to mention that I was referring to the quadrature counters
Craig
Ah. Well, if you have a signed 32 bit value and want to know what the upper word of the full 64 bit value would be, you'd just shift the lower value right by 31, like:
Or you could convert to a 64 bit longint, like:
but the latter is of very limited use right now, since 64 bit integers (longint) aren't officially supported and don't fully work.
What's the ultimate goal here?
Keeping track of a motor encoder @ 1.6M counts/sec (a rotary axis, like a spindle)
It would be nice to keep track of its actual position beyond the 32bit rollover.
Craig
Well, for totally basic arithmetic you could use a longint to keep track of things -- I think add, sub, and shifts, at least, will work on longints, and probably comparisons will as well. If you want to do some more complicated work you will probably have to split the longint up into a 32 bit high, low pair, but that's easy enough to do:
I think(tm) most of the obvious 64bit issues are gone now (literal parsing aside)
Sort of thing that I had in mind except for the longint. I mean, I have seen it in the manual but the wording kinda gave me the impression of "this is it but it doesn't work yet"
This is soooo cool, many thanks
Craig
Hmm, does Hex$ know about the longint?
Might help if I wasn't still on 5.9.6
Craig
print hex$(68719476735)
Only displays the lower 32 bits.
Craig
hex$() takes a uinteger parameter, so it only works on 32 bits. Someday we'll have to create a hexl$() for longints.
Not a problem. 64 bit counter working beautifully but I didn't know it until I split it to "lo" and "hi"....bit of a head-scratcher for a minute
Craig
Edit: Got some strange looks in the office when I used voice to ask Google and they heard the reply: "range of a 64 bit integer".
@ersmith
Bit of a quirk?
`dim as longint sixty4A,sixty4B
dim as uinteger hi, lo
sixty4A = &hFFFFFFFE
lo = &hCD2FA200
sixty4B = sixty4A<<32
sixty4B = sixty4B + lo
hi = sixty4B>>32
lo = sixty4B
print "method 1"
print hex$(hi)
print hex$(lo)
sixty4B =&hFFFFFFFECD2FA200
hi = sixty4B>>32
lo = sixty4B
print
print
print "method 2"
print hex$(hi)
print hex$(lo)
`
That's your literal getting truncated to 32 bit: https://github.com/totalspectrum/spin2cpp/issues/215
Thanks Ada. It's no biggie. I was only preloading for testing
Craig
Yep. Longints aren't completely ready for prime time yet, although we've made some good progress on them.
Once longints arrive, I betcha you/Ada/both could knock out double-precision floats before dinner time, right? 😜
Based on my tests.......looking really good now that I understand how to handle what we have
At full speed, this thing would need to run for ~170,000 years before rollover. What the heck, that's well out of warranty anyway
Craig
I go along with @"Heater." 's boss (or colleague):
"If you can't solve the problem with integers, you don't understand the problem"
I just eliminated a bunch of floats. Lost nothing but gained significant execution speed.
But then, my stuff is pretty simplistic
I’ve heard that quote in different variations from several sources. And faster would definitely be better!
Rather than hijack this thread, I’ll open a new topic in a bit where I can better explain what I’m up to and solicit some guidance.
ADDIT: The new thread is here: https://forums.parallax.com/discussion/174704/integer-calcs-instead-of-floating-point/p1?new=1
Unfortunately, no
. There are a whole load of library functions like sin(), cos(), log(), etc. that will need to be modified to support 64 bits.
Okay. So… maybe by supper then? 🤣