"Graphical" solve of nonlinear differentialequation with the propeller
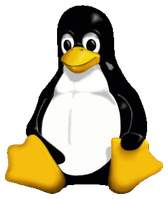
Hi,
if someone not interesst in math, skip it ;-))
I think about a alternative solution to do the numerical integration with integer by use the counter ( PSHA += FREQA) property.
Will try it, but now I have go to work .
best regards
Reinhard
if someone not interesst in math, skip it ;-))
/* Solve the Differential Equation x'(t) = r * x(t) * ( 1 - x(t) ) with Euler method and send x(t) as Analog out to P0 (RC lowpass recommended ) (P0 observed with an oszi) ------------------------------------------------------------------ */ #include <propeller.h> #include <math.h> #define P0 1<<0 #define CRTMODE (1<<28) + (1<<27) + 0 #define scale 16777216 // 2^32 / 256 typedef float (*Fct)(float x); void msleep(int t) { waitcnt((CLKFREQ/1000)*t+CNT); } float Log_EQ (float x) { float dt = 0.001; float r = 0.7; return x + dt * r * (1.0 - x); } void main () { int duty; Fct eulerstep; float x; int it; DIRA |= P0; // P0 = OUTPUT CTRA = CRTMODE; // set Counter Mode: DUTY SINGLE ENDED while(1) { x = 0.1; eulerstep = Log_EQ; for (it = 0; it <= 4000; it ++) { x = eulerstep(x); duty = (int)(x * 255); FRQA = (duty * scale); // set FRQA Register } FRQA = 0; msleep(100); } }
I think about a alternative solution to do the numerical integration with integer by use the counter ( PSHA += FREQA) property.
Will try it, but now I have go to work .
best regards
Reinhard
Comments
(It's great to see that someone is testing our floating point code, too. :-))
Regards,
Eric
here is the fixed point version (much smaller and faster)
used macros found on ARM website:
Propeller Version 1 on com7
Writing 588 bytes to Propeller RAM.
Verifying ... Upload OK!
/* no comment */ ;-)
Thank you for this tip, because I'm very lazy, I use it.
Reinhard
Your program looks very nice. It makes me wonder how much can be packed into a cog C program. It would be interesting to have a contest to see who could produce the largest or most interesting C program that fits in a cog.
Dave
Back to the thread, Reinhard, thank you for posting this! It is neat to see complex math being done on the Propeller. Complex being more than just algebra