Test code on a Coordinate Measuring Machine (CMM)
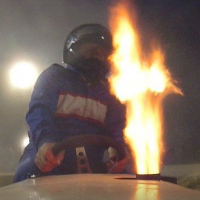
Hi,
I have a obsolete Romer CMM, all the encoders are operational but the main controller is dead. There are 6 joints on this arm, but to keep it simple to start with I'm only using the 3 major ones (waist,shoulder and elbow). This code reads the encoders just fine but the the conversion to degree's is glitchy, any thoughts on how to properly convert to degree's?
Thanks' in advanced.
Comments
I think you meant to do this:
pub count_to_degrees(count) : degrees return (count * 360_0 / ENC_RES1)
Note the underscore whre you have a decimal point. This will return the degrees in 0.1-degree units. I tested with this loop:
repeat c from 0 to ENC_RES1 step 50 d := count_to_degrees(c) term.fstr2(@"%5d %5.1f\r", c, d) waitms(10)
Here's the output on PST.
If it's helpful to your project, you can get an extra digit of resolution without any headaches.
Cool, That works great now. Thank You!
now I have to covert from degrees to radians then to XYZ, is there any trig objects ?
Now I'm trying to go to radians and I get the same glitchy behavior that I got with my first code.
PUB count_to_radians(count) : radians
return (count * 2_00000 * pi / ENC_RES1)
Thanks'
The problem you're having -- in both cases -- is using floats in your expressions. Floats are only lightly supported in Spin2, require special operators, and have to be translated back to integers, anyway
Here's the fix for radians which gives you three decimal places of resolution (you can get up to four if you think that will be useful).
con ENC_RES1 = 24000 pub main() | c, d, r setup() wait_for_terminal(true, 250) repeat c from 0 to ENC_RES1 step 50 d := count_to_degrees(c) r := count_to_radians(c) term.fstr3(@"%5d %6.2f %5.3f\r", c, d, r) waitms(10) repeat pub count_to_degrees(count) : degrees return (count * 360_00 / ENC_RES1) pub count_to_radians(count) : radians return (count * 6_283 / ENC_RES1)
Something you can do in Spin2 is return multiple values from a function. You could do this:
pub convert_count(count) : degrees, radians degrees := count * 360_00 / ENC_RES1 radians := count * 6_283 / ENC_RES1
If you want both values:
You can use an underscore to ignore a return value. For example, if you only wanted radians you could do this:
Super,
Thank You!
Ok,
I'm kicking it up to the next level, this code should (might) calculate the position of the tip of the arm, but again not seeing results that are not accurate.
Thanks'
Under Propeller Tool there is a problem with NAN. I also noticed that your fixed-point constants that are mismatched.
This:
Z_OFFSET = 209_55 X_OFFSET = -609_0 Y_OFFSET = 609_0
Should probably be this:
Z_OFFSET = 209_55 X_OFFSET = -609_00 Y_OFFSET = 609_00
I have programming friends that make fun of me because I'm so fastidious about code formatting, but this is why: sloppy formatting is an insidious creator of bugs.
You're also sending integers into methods that probably require floating point values; you may need to convert from integer to floating point first.
Create a method to solve the problem at hand. Send it KNOWN values that you have worked with on a calculator. Once the method works as intended, plug it into your application. In my world I avoid putting functional code into main(); I use main() to call pre-tested methods. That tends to keep main() smaller easier to follow.
LOL, so funny to read about my nemesis here: Romer is/was a joint venture between Homer Eaton (Carlsbad CA) and some French guy named Rohm(sp).
Homer accused me of copying his system that he ripped-off from Rolls-Royce. His version (at-the-time) utilized a non-contact IR-laser probe which was a PITA to work with. Mine was visible laser.
His original company, Eaton Leonard, came after me with some nasty, underhanded tactics. I put them out of business
and I loved every minute of it
Craig
@Brian_B
Not measuring tube formations by chance?
Craig
Edit: Point being that I have all the source-code that I will happily provide. Caveat: QuickBASIC kicked C butt once again (probe-scanning is MASM, however) so you'll need to work with a "toy language"

Edit2: Oh wait...SIX axis? Not familiar with this animal...they were always 5-axis
Craig,
I want to use it for mostly measuring small engine and tractor parts. I do have the original controller board (system 6) and it seems to be alive, but I don't know how it's communicating. It would be cool to see the code even if it's for a 5 axis.
Thanks'
Probably gonna be some pretty awful coding in there
Hardly a clue about programming at the time.
Craig
Cool! I'll take a look at it when i get a chance, Thanks'
This is pretty exciting stuff actually. I'd love to see a 6-axis CMM based on the Prop. Calibration is a bit of a nightmare but it's amazing what these portable systems can do.
Slightly different topic but the Prop would also make a nice alternative controller for
https://www.anninrobotics.com/
Craig
Yes the AR4 would be perfect with the p2 (I have one). It's going to take me a little bit to get up to speed on the p2, but it looks to like robotic control is in it's DNA.
Heck, you lucky so-and-so. Where do you live, I'll be right there
The fact that this arm can be 3d printed would fit well with Parallax's educational portfolio.
However, although Annin finally closed the position loops, the P2 version needs to run BLMs. There is no way (to my knowledge) of softening-up those stepper motors, meaning the arm cannot comply and nor can it be a "cobot".
This is a proper robot. Those wheelie things are autonomous vehicles.
I'm still working on this, but I'm really having a hard time finding any good examples of working with floating point in Spin.