Assembly Language across different microcontrollers question.
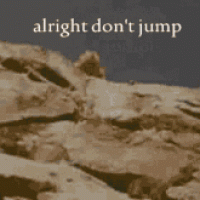
Is assembly language different that depends on the microcontroller you are using?
The reason I ask is because when I compile this assembly code, to run on my sx28 using the sx-key program, I get compile errors galore.
I am slowly TRYING to convert it using the sx-key manual, and the daubach manual. But its quite confusing. Do I even need to convert the program?
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Meh. Nothing here, move along.
The reason I ask is because when I compile this assembly code, to run on my sx28 using the sx-key program, I get compile errors galore.
I am slowly TRYING to convert it using the sx-key manual, and the daubach manual. But its quite confusing. Do I even need to convert the program?
$MOD751 ; ************************************************** ;* * ; * HDM3224 Application Note V1.0 * ;* * ; ************************************************** ; The processor clock speed is 16MHz. ; Cycle time is .750mS. ; Demo software to display a bonsai ; tree bitmap image and 4 lines of ; text on a 320 x 240 LCD. org 00h ljmp start ;program start org 100h ; Initialize the 32241 ; Text page 0000h 04afh ; Graphics page 04b0h 2a2fh start: mov r1,#40h ;system set lcall comm32 mov dptr,#msg1 ;ss param lcall data32 mov r1,#44h ;scroll lcall comm32 mov dptr,#msg2 ;scroll param lcall data32 mov r1,#5dh ;csr form lcall comm32 mov dptr,#msg3 ;csr param lcall data32 mov r1,#4ch ;csrdir lcall comm32 mov r1,#5ah ;hdot scr lcall comm32 mov dptr,#msg18 ;hdot param lcall data32 mov r1,#5bh ;overlay lcall comm32 mov dptr,#msg4 ;ovrly param lcall data32 mov r1,#59h ;disp on/off lcall comm32 mov dptr,#msg5 ;disp param lcall data32 ; clear the text page lcall clrtext ; display bitmap mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg6 lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg12 lcall data32 ; display text mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg7 lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg14 lcall data32 mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg8 lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg15 lcall data32 mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg9 lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg16 lcall data32 mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg10 lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg17 lcall data32 sjmp $ ;stop ;************************************************* ;SUBROUTINES ; comm32 sends the byte in R1 to the ; 32241 display as a command comm32: setb RA.2 ;a0=1=command comm321: mov a,r1 ;get data byte mov RB,a clr RA.0 ;CS the display clr RA.1 ;strobe setb RA.1 setb RA.0 ret ; write32 sends the byte in R1 to the ; 32241 display as a data byte. write32: clr RA.2 ;a0=0=data sjmp comm321 ; data32 sends the message pointed to ; by the DPTR to the 32241 display. data32: clr a ;get the byte movc a,@a+dptr cjne a,#0a1h,data321;done? ret data321: mov r1,a lcall write32 ;send it inc dptr sjmp data32 ;next byte ; Clear text RAM on the 3224 clrtext: mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg13 ;cursor param lcall data32 mov r1,#42h ;mwrite lcall comm32 mov dptr,#msg11 ;all spaces lcall data32 mov r1,#46h ;set cursor lcall comm32 mov dptr,#msg6 lcall data32 ret ;************************************************ ; TABLES AND DATA ; Initialization parameters for 3224. msg1: db 30h,87h,07h,27h ;system set db 39h,0efh,28h,0h,0a1h msg2: db 0,0,0efh,0b0h ;scroll db 04h,0efh,0,0 db 0,0,0a1h msg3: db 04h,86h,0a1h ;csr form msg4: db 01h,0a1h ;overlay param msg5: db 16h,0a1h ;disp on/off msg6: db 0b0h,04h,0a1h ;set cursor to ;graphics page msg7: db 31h,2h,0a1h ;set cursor ;text page ;1st line msg8: db 59h,2,0a1h ;2nd line msg9: db 81h,2,0a1h ;3rd line msg10: db 0a9h,2,0a1h ;4th line ; 1200 spaces for text page clear ; The following table is not listed ; here, except for the first 8 bytes, ; but consists of 1200 bytes ; all of which are 20h msg11: db ' ' db 01ah msg18: db 0,01ah ;hscr param ; 320x240 bonsai tree graphic ; The following table is not listed ; here. It consists of 9600 bytes ; which constitute a full screen ; bit map image of a bonsai tree. ; You may add a few bytes before the ; 01ah termination byte for testing ; puposes or include a complete ; bitmap image msg12: db 01ah msg13: db 0,0,01ah ;set cursor ;to text page msg14: db 'HANTRONIX' db 0a1h msg15: db 'Crystal Clear and' db 0a1h msg16: db 'Visibly Superior' db 0a1h msg17: db 'LCD Modules' db 0a1h end
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Meh. Nothing here, move along.
Comments
It tends to be completely different in terms of detailed specifics such as syntax and opcode names. It tends to be completely the same in terms of having the same types of core instructions and operations. To put it a little better, it's sort of like asking if a book written in Spanish can be read by someone who only speaks English. They're both languages, they have words, grammar, etc, share a (mostly) common alphabet, and so on. However, you wouldn't expect someone who only speaks English to read a book written in Spanish, right? Same thing for assembly languages.
That's why folks use compiled languages for lots of things. The compiler is the translator that takes a common language (C, BASIC, Fortran, etc) and converts it to the actual assembly language that a particular chip speaks. Long story short? You'll never get assembly code from a completely different chip (in your case an 8051 variant) to assemble on another chip directly. You can cross convert it, but it's a lot of work and you'll need a combination of good comments in the original source (so you know what it's trying to accomplish) coupled with a good understanding of the source assembly language to perform the conversion.
Thanks, PeterM
You really have to understand ther fundamental differences in both Assembly languages and both pieces of hardware.
You apparently have code for another device written in a compiler developed for that device. Sometimes you may just have a portion of code without proper initialization and no where to go.
Now you have to learn SX Asm and SX hardware to translate it into anything useful. PIC and Senix don't mix, but code can be shifted over if you learn both. Memory is different between the two.
This is why high level languages [noparse][[/noparse]such as Basic and C] were developed.
If you really want to learn both, it is a good exercise.
If not, try to just sort out a Flow Diagram to help you code in SX Asm or SX/B.
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
"When all think alike, no one is thinking very much.' - Walter Lippmann (1889-1974)
······································································ Warm regards,····· G. Herzog [noparse][[/noparse]·黃鶴 ]·in Taiwan