Shortening redundant code
Hey guys.· I am going to be using the I2C command set alot for this project that i am working on.· Here is a snippet of what i wrote
I know that i should be able use one SendIt command that would have all the I2C stuff in it and just send the variables to it.· I can write it like this
i cant understand how to send the data to the variables and to the SendIt subroutine
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
It's Only A Stupid Question If You Have Not Googled It First!!
' ------------------------------------------------------------------------- ' Subroutine Code ' ------------------------------------------------------------------------- FontSequence0: I2CStart SDA 'Display First set of sequence I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $00 I2CSend SDA, $01 I2CSend SDA, $00 I2Csend SDA, $01 I2CStop SDA Pause ScrollSpeed I2CStart SDA 'Display Second set of sequence I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $01 I2CSend SDA, $00 I2CSend SDA, $01 I2Csend SDA, $00 I2CStop SDA Pause ScrollSpeed Return FontSequence1: I2CStart SDA I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $02 I2CSend SDA, $03 I2CSend SDA, $04 I2Csend SDA, $05 I2CStop SDA Pause ScrollSpeed I2CStart SDA I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $03 I2CSend SDA, $04 I2CSend SDA, $05 I2Csend SDA, $02 I2CStop SDA Pause ScrollSpeed I2CStart SDA 'Display Second set of sequence I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $04 I2CSend SDA, $05 I2CSend SDA, $02 I2Csend SDA, $03 I2CStop SDA Pause ScrollSpeed I2CStart SDA 'Display Second set of sequence I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $05 I2CSend SDA, $02 I2CSend SDA, $03 I2Csend SDA, $04 I2CStop SDA Pause ScrollSpeed Return
FontSequence2: I2CStart SDA I2CSend SDA, TopSlaveID I2CSend SDA, $60 I2CSend SDA, $20 I2CSend SDA, $0A I2CSend SDA, $06 I2Csend SDA, $20 I2CStop SDA
I know that i should be able use one SendIt command that would have all the I2C stuff in it and just send the variables to it.· I can write it like this
Wing Var Byte M0 Var Byte M1 Var Byte M2 Var Byte M3 Var Byte SendIt: I2Cstart SDA I2CSend SDA, Wing I2CSend SDA, M0 I2CSend SDA, M1 I2CSend SDA, M2 I2CSend SDA, M3 I2CStop SDA
i cant understand how to send the data to the variables and to the SendIt subroutine
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
It's Only A Stupid Question If You Have Not Googled It First!!
SXB
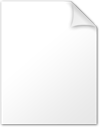
9K
Comments
As you figured out, your code is redundant (always sending four bytes) so you could create a subroutine like this:
Update_Display:
· temp1 = __PARAM1
· temp2 = __PARAM2
· temp3 = __PARAM3
· temp4 = __PARAM4
· I2CSTART SDA
· I2CSEND SDA, TopSlave
· I2CSEND SDA, $60
··I2CSEND SDA,·temp1
··I2CSEND SDA,·temp2
··I2CSEND SDA,·temp3
··I2CSEND SDA,·temp4
· I2CSTOP
· RETURN
Of course, you'd have to define this at the top of the program like this:
Update_Display··· SUB·····4
... and then -- here's the cool part --·you can call it from your program like this:
· Update_Display $00, $01, $00, $01
This will be easier to code, and save a lot of space as you're·expanding·fewer I2CSEND instructions.
·
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Post Edited (Jon Williams (Parallax)) : 2/1/2006 8:41:41 PM GMT
thanks, Brian
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
It's Only A Stupid Question If You Have Not Googled It First!!
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
I2Cstart SDA, TopSlave
Can the address to the i2c chip be on that line? It is not shown that way in the example
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
It's Only A Stupid Question If You Have Not Googled It First!!
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
·1+1=10
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Post Edited (Jon Williams (Parallax)) : 2/1/2006 3:42:10 PM GMT
I think what has you confused is that Jon wrote "I2CSTART SDA, TopSlave"
I believe he meant:
"I2CSTART SDA"
"I2CSEND TopSlaveID"
Bean.
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
"SX-Video·Module" Now available from Parallax for only $28.95
http://www.parallax.com/detail.asp?product_id=30012
"SX-Video OSD module" Now available from Parallax for only·$49.95
http://www.parallax.com/detail.asp?product_id=30015
Product web site: www.sxvm.com
"Ability may get you to the top, but it takes character to keep you there."
·
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax