Random numbers
Is it possible to generate a random number within limited parameters, such as a number from 1 to 20? Or 20-40? Whatever?
Is it possible to have the uC pause, or better yet, sleep, for 30 minutes? Seems to me if the cmd has to be in ms I would have to tell it to Sleep 60000 to get 1 minute of sleep, or Pause 60000. Finally, combining the 2 concepts, can I get it to pause a random amount of time from 20-30 minutes? Could I quantize it so that it's either 21, 22, 23. . . 30 minutes but not anything in between?
Here's my proposed solution to the puzzle, but I'm betting both that this wouldn't work and that even if it would there's a much easier way. I only found the MIN and MAX commands after I did this--would they help? Could I use them to directly generate a random number from 20 to 30? Lastly, how can I get it to sleep for 20-30 minutes instead of 20-30 milliseconds?
Post Edited (Mongojerry_2) : 3/11/2005 8:15:42 AM GMT
Is it possible to have the uC pause, or better yet, sleep, for 30 minutes? Seems to me if the cmd has to be in ms I would have to tell it to Sleep 60000 to get 1 minute of sleep, or Pause 60000. Finally, combining the 2 concepts, can I get it to pause a random amount of time from 20-30 minutes? Could I quantize it so that it's either 21, 22, 23. . . 30 minutes but not anything in between?
Here's my proposed solution to the puzzle, but I'm betting both that this wouldn't work and that even if it would there's a much easier way. I only found the MIN and MAX commands after I did this--would they help? Could I use them to directly generate a random number from 20 to 30? Lastly, how can I get it to sleep for 20-30 minutes instead of 20-30 milliseconds?
Post Edited (Mongojerry_2) : 3/11/2005 8:15:42 AM GMT
bs2
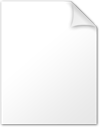
805B
Comments
Yes, you can create a limited range. Remember that the RANDOM function spits out values from a 65536-element (0 - 65535) list, so dividing the output into your range is an easy way to go. Let's use your 20 - 30 suggestion:
· RANDOM randVal
· delay = randVal / 6553 + 20
For longer sleep periods you'll need to use a loop.
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA
As to sleeping for 20 minutes I need it to sleep for 65,535 milliseconds about 20 times. Seems to me for what I want to do, building on your code above:
For X =1 to delay
· Sleep 65535
Next
Does delay have to be an integer for this to work?
·
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA
Go_To_Sleep:
· FOR restTime = 1 TO delay
··· SLEEP 60
· NEXT
· RETURN
The units for sleep are in seconds, so SLEEP 60 will go into low-power mode for approximately one minute (see SLEEP in the help file for details).·
Another thing to keep in mind is that the RANDOM function is actually pseudo-random, so to get real randomness you'll want to tumble the seed until you actually need the random value.· You can do this in your main loop:
Main:
· ' main loop code
· IF (Some_Condition) THEN
··· GOSUB Go_To_Sleep
· ENDIF
· RANDOM delay
· GOTO Main
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA
I·see-- I thought sleep was in milliseconds.
I understand about tumbling the random number, but my interest in this program is for it to go to sleep for 20-30 minutes when the program starts. My solution was to tumble the seed value one time:
Pause_routine:
··RandVal·= 11000
··For X = 1 to 2
··· ·Random RandVal
··Next
· Delay = RandVal / 6553 + 20
· For X = 1 to Delay
··· Sleep 60
· Next
Will that work?
Post Edited (Mongojerry_2) : 3/11/2005 6:58:39 PM GMT
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA
InitialRandom_Routine: 'To get the first random #
RandVal = 11000
FOR X = 1 TO 5
RANDOM RandVal
NEXT
Waiting_routine: 'To sleep 20-30mins
RANDOM RandVal
Duration = RandVal / 6553 + 20 '20-30
FOR X = 1 TO Duration
SLEEP 60
NEXT
RANDOM produces a list of random-looking list values that don't repeat until after 65536 iterations of the function. As the function is mathmatically based, the same seed (11000 in your program) will ALWAYS produce the same result. The only way to get true randomness is to insert some external event -- like a human pressing a button, etc.
You could, I suppose, use a really low-quality capacitor and read it with the POT command; the low quality cap *might* give you a different value when you read it. If you use a photocell for the R component of the POT circuit, the light falling on it when read will affect the reading.
On RANDOM not being truely random ... my boss (the guy who invented the BASIC Stamp) wrote a really clever Simon game that takes advantage of RANDOM's behavior
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA
RandVal = 11000
FOR X = 1 TO 5
RANDOM RandVal
NEXT
That uses random seed values. By the time this goes to the next line, RandVal is no longer based on the 11000 seed value. That's where I'm confused.
Assuming this works I have another question. Should I want to use the button for another purpose later in the program do I have to clear its workspace to zero before doing that?
InitialRandom_Routine:················· 'To get the first random #
··· RandVal = 11000
··· DO
····· RANDOM RandVal
····· BUTTON 0, 1, 255, 255, Btn, 1, Waiting_Routine
··· LOOP
Waiting_routine:······················· 'To sleep 20-30mins
· RANDOM RandVal
· Duration = RandVal / 6553 + 20
· FOR X = 1 TO Duration
··· SLEEP 60
· NEXT
Reset:
· DO
··· RANDOM delay
··LOOP UNTIL (StartBtn = Pressed)
Use the PIN definition for StartBtn and define a CONstant for Pressed (1 for active-high [noparse][[/noparse]pin pulled down], 0 for active-low [noparse][[/noparse]pin pulled up])
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Jon Williams
Applications Engineer, Parallax
Dallas, TX· USA