A question about qsin
I am studding Spin2 on a Prop2
Would anyone mind breaking this down into an explanation of what is going on or point me to a documentation of qsin
I get the Level, Y and X but not the 256, 128 or shift left.
Y := (qsin(level, x++, 256 ) + 128 ) << 8
Comments
Spin2 documentation is available here:
-- https://docs.google.com/document/d/16qVkmA6Co5fUNKJHF6pBfGfDupuRwDtf-wyieh_fbqw/
If you have a P2, why not create a simple test program to examine the output? Do things incrementally. I started here:
From 0 to 127 the output values were positive. 128 was zero. from 128 to 255 the output values were negative. This shows that QSIN returns a signed value. At position 63 (corresponding to ~90 degrees) the output was equal to the length (first parameter).
Sadly, Parallax hasn't had the same staff or resources to create the excellent docs we had with the P1, so it's up to us to experiment. Do share results; I'm sure others will appreciate it.
Yes indeed I do have some P2 but what is this
term.fstr2(@"%3d %4d \r", x, y)
Maybe this code makes it more clear:
Andy
Actually Andy that helped a load because I was losing time trying to understand serial formatting using term.fstr2 suggested by Jon, your debug line made all the difference. I should look up more of this debug command because it appears more closer to the hardware.
Indeed, DEBUG is built into the compiler. For formatted output I use my jm_fullduplexerial.spin2 which is included in the library files if you downloaded and installed Propeller Tool.
Appreciate your input Jon, however, I am really against using third party libs if possible; or one never learns how to do stuff. I am more of creating working and complete snippets of code then later sticking them together in my own messy style.
Here's the code for 8-Bit version, trying to figure now how to do a true 16-Bit version but I do not think this is really possible given the DAC registers are just 8-Bit; I do have an idea:
`
CON
_clkfreq = 300_000_000
MAX_LEN = 64
BUF_SIZE = 32
VAR
long i,Radius, Angle, S1,S2,loops
PUB Main( )
Pinlow(8)
Pinlow(9)
radius :=127
repeat loops from 0 to 10000
repeat angle from 0 to 359 'step 15
S1 := qsin(radius, angle, 360)
S2 := qcos(radius, angle, 360)
S1:=S1+128
S2:=S2+128
wrpin(8, p_dac_124r_3v + (S1 & $FF) <<8)
wrpin(9, p_dac_124r_3v + (S2 & $FF) <<8)
'debug(sdec(S1),sdec(S2),sdec(angle))
'waitus(10)
`
And the result:
The DACs themselves are 8 bit, but there's a smart mode that does dithering to get almost-16bit precision (almost because it clips at $FF00): https://p2docs.github.io/pin.html#p-dac-dither-rnd
Meaning that it would be 65536 - 255 = 65281 ?
Yes.
I found this, on a youtube video linked at the bottom
Its supposed to be 16bit but it does not appear to be working as it should, of course I could have made a typo.
The output pin somehow gets activated, goes from zero to 3.3V but without steps . For testing I use a real scope on the P2 pin.
Any ideas chaps?
`
CON
_clkfreq = 300_000_000
_pin = 8
PUB go( ) | i
pinstart(_pin, p_dac_124r_3v | p_oe | p_dac_dither_pwm, 256, 0)
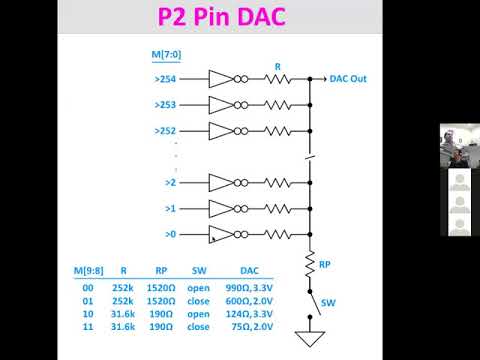
repeat
wypin(_pin, $7f00 + (i & $3ff))
waitms(20)
i++
`
This code produces a 16bit sine wave at pin 8 with about 10ms periode
Andy
BTW: use 3 backticks before and after code, not only one
A more general version in this thread:
https://forums.parallax.com/discussion/comment/1496551/#Comment_1496551
Lovely Cheers, that does prove that it works and it will get me progressing.
Re: the Backticks, I always selected them from the dropdown, its a bug on the website ?.
If you do it with the dropdown, you need to select the whole code first.
One Backtick is inserted if you select a part of a single line.