First tries
I tried Thonny and Mu, but ended up using Python Microbit.org/v/3 on the Chrome browser. It takes some getting used to, in terms of of how to use it. It does have the capability to turn your script code into a usable .hex file.
I like that it has the microbit graphic in the upper right hand corner. While working with terse temperature code, I noticed I was getting two different values when the program is run. The graphic in the upper right hand corner seems to be closer in accuracy than what is showing on the actual microbit.
I guess I will have to set up a small logger to see what is actually being captured by the program.
So far so good, next I might have to see how the BT works, not sure I like the pairing you have to go through.
Ray
Comments
Below is the start of my temperature code. Now I have to add some code for streaming some values to file.
Ray
[deleted]
In the program below I added open file code. The program runs without errors, but I cannot figure out where that open file is at. I checked the Downloads folder, it is not there. Anybody know where the program puts the file?
Ray
The file will be stored in microbit flash, this is nice for small files and programs but is not practical for larger data. The workaround is to add external storage or write the data to the PC. Bluetooth is a little hard to achieve with the microbit so maybe you might want to explore other communication protocols. For storage the Adafruit SPI flash SD card is a pretty good deal there are breakouts with 3 different memory capacity if I recall, plus this takes you into SPI with the microbit which is really quite easy and fun to use.
I am away from home until the weekend but I have some reasonable resources for creating, saving and displaying csv files some of this requires a Python GUI on a PC but is not needed if that is not the way you want to go. When I get home if you are still working on creating csv files I'll post a little info. in that respect.
A quick note on file access Google Python "with open", this is a feature that will open and close a file in a more fail safe way than the method you are currently using.
P.S. I'm sure this should work with the microbit but not 100 % certain, try it and see.
After doing some reading, I got the impression that the file was being created/opened in the microbit flash. So, I guess one way to get the file/data out of microbit is to use the uart command, with an attached, lets say RPi. This is sort of becoming a little clunky, but work with what you got.
I was thinking about having more than one microbit, in different locations collecting temperature data and having it stored in a csv file where I could do some data analysis using pandas. To bad the RPi doesn't have a corresponding radio transceiver.
Ray
This is working without errors, opens a log file , writes to it, then prints what was written to it. Just wanted to verify that something was working as expected. Now, is there some way of doing a dir of the flash file system, might want to see what is in the flash.
Ray
I found this example code, it is little more useful, this creates a my_data.htm on the microbit drive. When you open that file up it gives you different options that can be used. One command is the download, which copies the my_data as a .csv file to your Downloads folder. I will have to play with this for awhile to see what I come with.
Ray
Use os for exploring flash file system
Another point, you did well using "with open" but by using that method you don't have to close the file so any "f.close()" can be removed from your script.
You can list the directory of the flash file system of a Micro:Bit in MicroPython with:
I use Thonny as it gives you lots of options in developing your code...
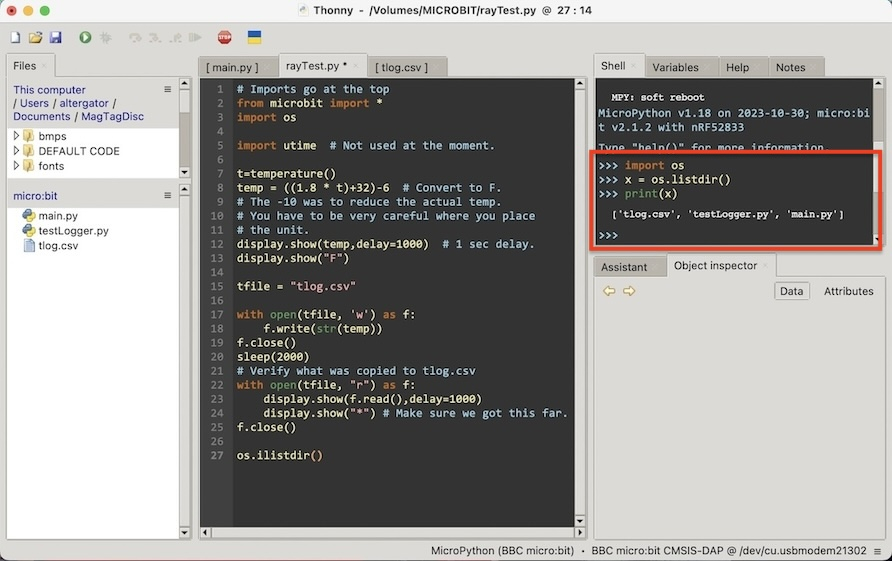
dgately
I tried working with Thony, no success. I installed the microbit 3 plugin, but Thony cannot find stuff. I am working in a Linux session. Does Thony know how to convert .py files to .hex files and run it on an attached micrbit?
Ray
Thonny is cross-platform.
Once MicroPython (an interpreter with interactive mode) is installed and running, you don't need to convert .py files to .hex
I used Thonny to install MicroPython onto an old micro:bit (Tools -> Options -> Interpreter).
I ran this line of code in the REPL:
Then I wrote a simple one-liner in the editor and told Thonny to run it. Success. Then I saved the the code as hello.py onto the micro:bit. Then I used @dgately's suggestion above and there's the file (in the micro:bit file system).
It seems you're making [incorrect] assumptions about how MicroPython interreacts with Thonny. It's the same as standard Python which I also use Thonny for -- the only difference is that when running MicroPython the interpreter is on an external device.
Check in the lower right-hand corner of the Thonny display to see which interpreter and port it's connected to. You can change this by clicking on it.
Once you get this going, you'll be able to use the REPL to test bits of code live, and then copy-and-paste that code into your program.
Once you get things sorted, you may want to bookmark this site:
-- https://microbit-micropython.readthedocs.io/en/v2-docs/
I was working with Thony some more, and at one point it was presenting something to do with replacing the microbit firmware with a Thony microbit firmware. I am probably going to hold off going any further with Thony, until Iget a better handle on things.
Ray
Well, the Thonny fork seems to work well -- I run native in Windows and even made it work in Ubuntu using a VM. If your goal is to be productive and free to experiment, I think Thonny Is the way to go.
Now I have to decide how far or how much time and money I want to invest in this new project. In order to make some really useful projects ,expansion would be the way to go. So far all that I have seen is expansion via a breadboard. But, definitely Thony will be the way to go, I might even switch my regular Python programming from Geany to Thony.
Ray
One thing to note about Thonny and Micro:Bit (or even Arduino RP2040's, Raspberry Pi Pico's & ESP32's) is that you can save your code on the board as "main.py" and it will run when the board is rebooted (or reset)...
When you use the "Save" menu on your source, Thonny gives you a choice to save the file on your PC or to the board. I save a copy of the file with a descriptive name on my computer (Mac) and then again as "main.py" on the Mirco:Bit.
dgately
I did. For the PC I'm usually writing small command line utilities and I like that there's a command line arguments box right on the screen (upper right).
@Rsadeika I want to correct something I said earlier about the "with open" method. Every time you use "with open" it will overwrite the existing file because microbit has no "append" mode so keeping a file open until all data has been written is probably what you want to do and is what you were doing to start with.
This is from the documentation
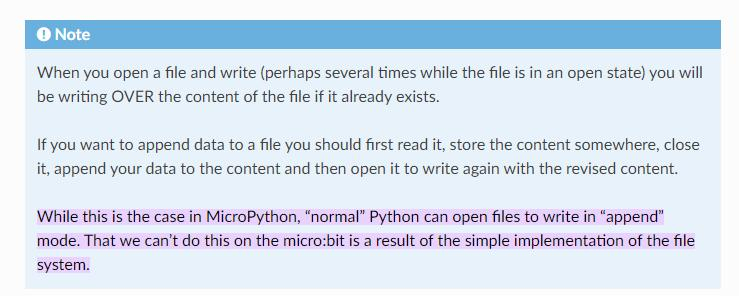
Sorry for the earlier misleading info