Passing method pointer as parameter
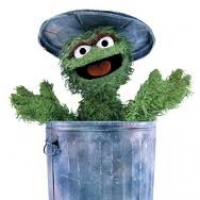
I'm wondering if there's an easy fix for what I'm trying to do. My Spin 2 program sends commands to a TFT display to draw objects. I have a method that draws a numeric entry keypad, waits for user entry, then returns to the previous screen. When entry is complete, I'd like the option to run a method specified by the caller. I can do this with a method pointer:
PUB DoNumericEntry(xPos, yPos, {lots more parameters}, MethodToRunWhenComplete) { numeric entry code } MethodToRunWhenComplete() {code to return to previous screen }
And it would be called like this:
DoNumericEntry(200, 50, {other parameter values}, @SomeMethod)
Generally this works fine. But the problem is that SomeMethod can't have any parameters of its own in order to be passed as part of DoNumericEntry. It would be nice if SomeMethod could have any number of parameters, but I don't know how to accomplish this. Clearly this doesn't work:
DoNumericEntry(200, 50, {other parameter values}, @SomeMethod(a, b, c))
My current workaround is to create an intermediate method that calls SomeMethod with global variables:
PUB SomeMethodWrapper() SomeMethod(global1, global2, global3)
And use that as the method pointer:
DoNumericEntry(200, 50, {other parameter values}, @SomeMethodWrapper)
Is there a better way to accomplish this? I should mention that everything is in the same cog.
Comments
I think you can pass a method pointer -- which is just a long -- but you'll have to use globals as you're doing. I've never really worked with method pointers so I did this simple test.
This might be a better way to go if you're method exists in another object.