I got the blues

Blues wireless has been out for a while now and it offers a convenient solution for sending and receiving data from the cloud. AWS and AZURE have solutions but it requires a complicated process.
Back about 20 years ago IBM came out with MQ Series for moving data between systems. This solution guaranteed delivery of a message from one system to another no matter what the protocol. Routes were setup between the systems and a message send on one system would then be routed to the other system even if the message was broken up and sent by different methods. The system would reassemble the message and present it in order to the end system.
Since then it has become open source and is now called MQTT. This is what AWS and Azure use to send messages to and from the cloud. MQTT is available for a number of processor and one could write it for the P1/P2. In addition security and encryption needs to be added so that messages cannot be intercepted.
Now comes Blues wireless that has several products to allow you to send messages to the cloud and have them routed to where you need them. The Cellular Notecard and WiFi Notecard have built in STM processors that take care of moving the message from your device to the cloud.
The communication between your Micro controller is simple I2c or serial. You just need to build a JSON packet with the message you want to send and the Notecard takes care of moving it to the cloud. Once it reaches the cloud it can be forwarded to AWS, Azure, or a website that you have setup.
The Notecards require a carrier board to mount the Notecard and provides connection between your micro controller and the Notecard. The Notecard has a special connector that you could build your own interface too.
The nice thing about the Cellular Notecard is that it comes with services that doesn’t expire for 10 years. No more signing up for a plain and paying a monthly fee to send data to the cloud.
The Cellular Notecard comes with 500Meg of data so when you use up that data you have to buy more at a cost of about $5 per 150Meg. In addition there is a cloud fee for the number of transactions that you use.
In addition when you’re not using the cloud the unit uses very little power and only wakes up when you want to send or receive a message.
They have some quick start tutorial to help you get started.
Within a few minutes I was up and running and was able to send and receive a message from the cloud.
sample data sent:
{"temp":35.5,"humid":56.23}
sample data received:
{ "time": 1675878573, "body": { "key1": "val1" } }
Mike
Comments
My next task was to interface it to the P1/P2.
My initial thought was to use the QWIIC connector on the board but that proved to be a no go. My other devices that use a QWIIC connector power the device and you just had to write a I2C driver to interface to the device. Well, the Carrier board does not allow powering the device that way and so I abandoned that idea and proceeded to use the serial interface instead.
This involved wiring up a cable to the RX and TX pins on the carrier board as shown in the picture above.
Writing a driver proved to be a little challenging in that serial data is not like I2C where you send a request and get a response. Serial you send a request and then have to wait for a response that you don't know what you're going to get.
I had to write a background task to receive the data asynchronously and while I was at it I had it also send the data as well.
Now I need to somehow know when I got all the data and not just some of it and have to put it back together somehow. It turns out that when the data is ready it is terminated with a carriage return and line feed. So, I just had to wait for that value and signal the process that a full packet of data was ready to be received.
Dealing with JSON is another thing in that it is wordy and requires quote marks all over the place. Luckly, I have a JSON library that I put together before and just had to make a few changes to it to make it work.
For example this is the return data for the Notecard:
Parsing the "ver_major", "ver_minor", and "ver_patch" was simple enough using the JSON library functions.
Test program:
I will be putting up my library on my github page. Mostly it's a full duplex serial driver.
Mike
Hello!
I've seen the thing. Nice! However Sparkfun does sell a breakout board for connection other devices that use I2C protocols but isn't wired with their unique connectors. Try this https://sparkfun.com/products/14495 It is their adapter, they call it "SparkFun Qwiic Adapter" and it would work to connect the P2 or even the P1 to the products that the company sells who uses those methods.
Two mascots.
@"Buck Rogers"
Yes, I made the mistake of buying the Adafruits STEMMA cable instead of Sparkfuns QWIIC cable. The Adafruits cable is far too stiff and the part that it's connected to flys all around. Sparkfuns cables are a little more flexible and I just picked up a couple of those.
I tend to like the Silcon wire from Adafruits Hookup Wire.
I picked up a couple of these cables QWIIC cable and just buy a 4 pin header like this Harwin. That makes it a simple plug and play.
I have several other devices that I'm playing with that use the QWIIC connector.
Mike
Looks like the unit will be good for another week. I'm only down 200mv since I started logging.
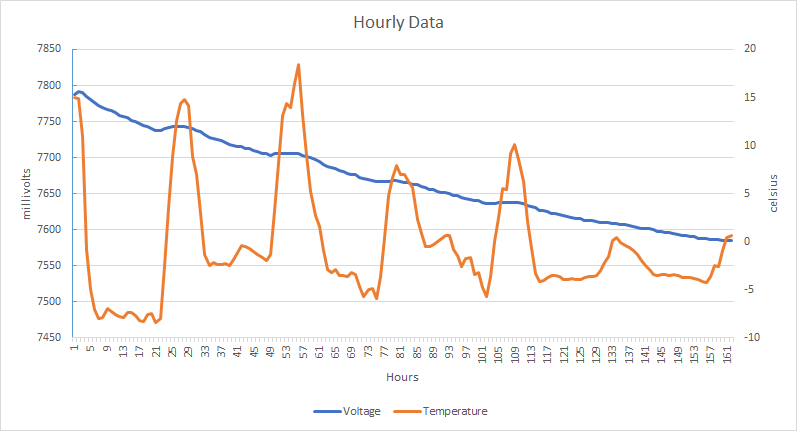
Looking at the chart is looks like the battery voltage dropped as the temperature dropped and the battery did better when it started to get warmer.
Mike