Panel 64 Cube and More...

Anyone make a cube yet using the P2. I have been toying with it for a while and couldn't come up with a decent cube design.
After looking at a simple design I was able to put one together. Here is a picture of the guts:
I 3D printed a bottom frame, side frames, and a top frame. The side frames are held in place with M3x6 screws using brass inserts. The top frame uses magnets to hold it in place.
Those little screws are hard to see, and I needed a very small screwdriver to get them in and out.
The guts are made up of a P2 plug and play with a Parallax WiFi module for programming and a IN260 power monitor just to keep track of the power along with a 5 volt 3 amp regulator. This is all powered with two 18650 batteries.
Here is a video of it running a maze program.
Panel 64 Maze Video
Mike
Comments
Awesome video, great job. Are you using Stephen Moraco's HUB75 drivers or did you roll your own? I would like to get a copy of the STL file(s) for your panel mounts, if you are willing to share...
@"Francis Bauer"
Everything written from scratch as I code in C and not spin. I wrote a panel driver program which was simple enough to do since I was only dealing with the Parallax 64 panels and only 7 colors.
Attached are the stl files for the base, sides, and top. 2mm x 2.5mm magnets.
Mike
Thank you, Mike
Back again, This time I turned my panel64 cube into a dice.
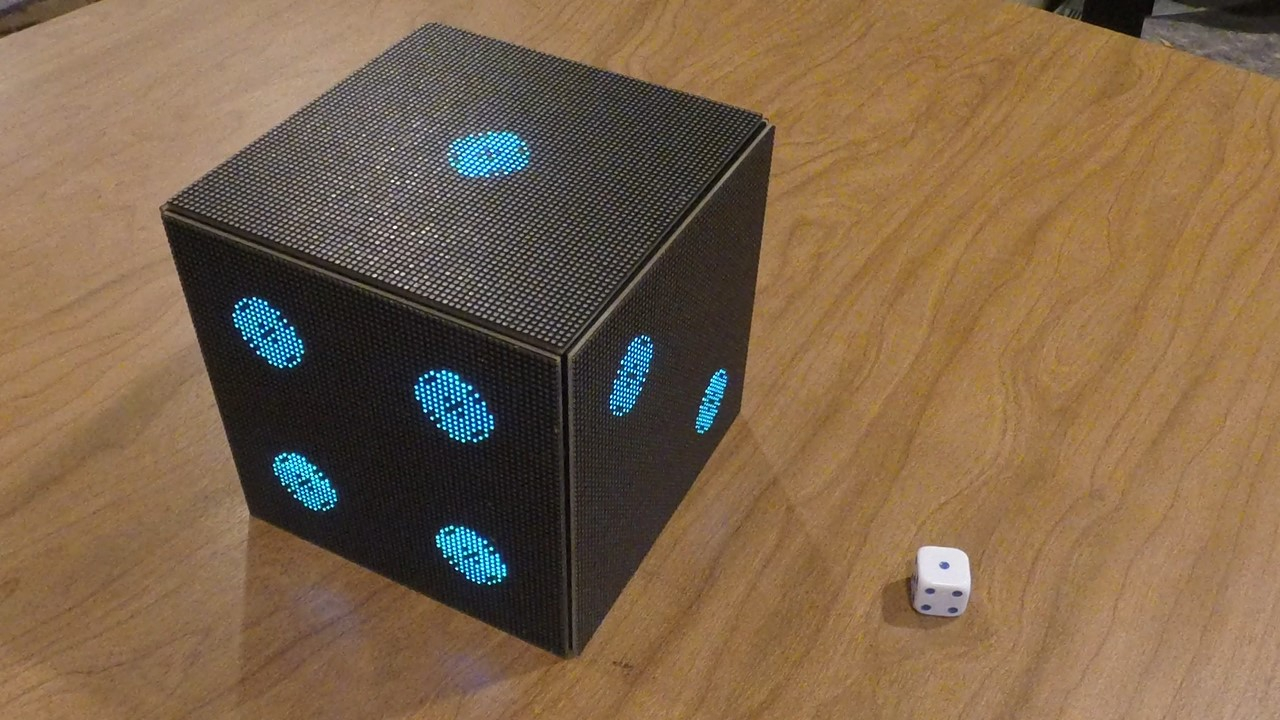
To make the dice work I needed to divide the dice into sections so that I could draw a circle for each value.
Each panel has 64 led's so have of that would give me the center which would be 32. And half of that would give me 16.
Now I have 9 regions to play with: (16,16), (32, 16), (48, 16), (16, 32), (32, 32), (48, 32), (16, 48), (32, 48), (48, 48).
To draw each face I created an array of binary data that represents each dice value. 256 128 64 32 16 8 4 2 1.
So the number 1 would be in hex: 010 ---> 000010000. This represents the center region on the face (32,32).
Now I need another array so that I place the correct faces on each side based on the up face.
When the dice is rolled it returns the up face and I draw each of the corresponding faces.
Here is a video of the dice being rolled and the cube changing as the value from the dice is returned.
Die being rolled.
Mike
These panels are cool. Nice project!
For a moment I thought you were rolling the whole LED cube when I read this. LOL. I also learned that Bluetooth dice are thing these days.
It's a very neat looking final result. Well done. Any issues with overheating under load? Not the dice application, but in general when lots of LEDs are lit and the cube is fully closed up.
@rogloh ,
Power not an issue. The cube fully powered uses less than 1 amp. With all the LED's lit white it uses just over 1 amp.
Not a cheap experiment at just over $300.
Mike
Super tidy, Mike. Congrats.
Do you open it up to recharge the batteries?
@Tubular ,
Correct, the top is held on with magnets.
Mike
Back Again, This time were talking to the cube or is that talking to the Hand?
Well, were not actually talking to the cube but to a MAX78000 feather board that has been programmed to understand 15 key words.
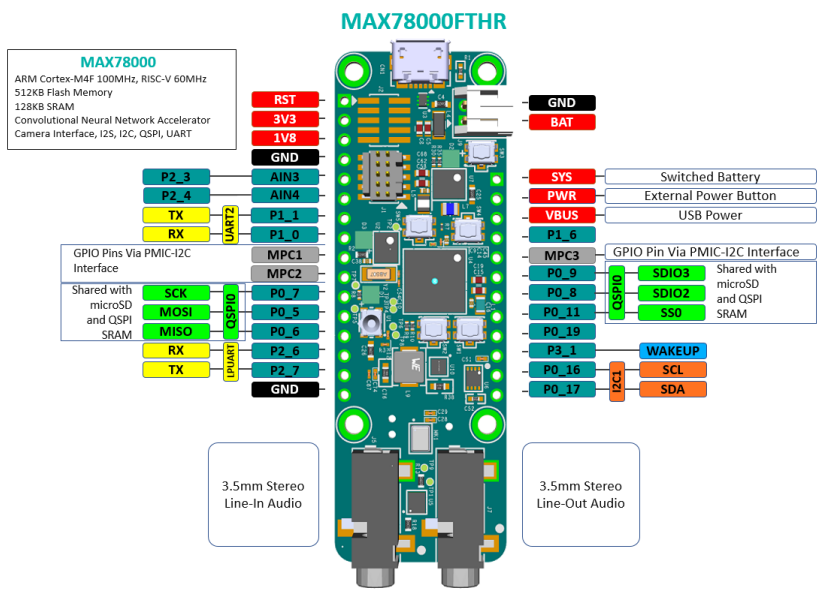
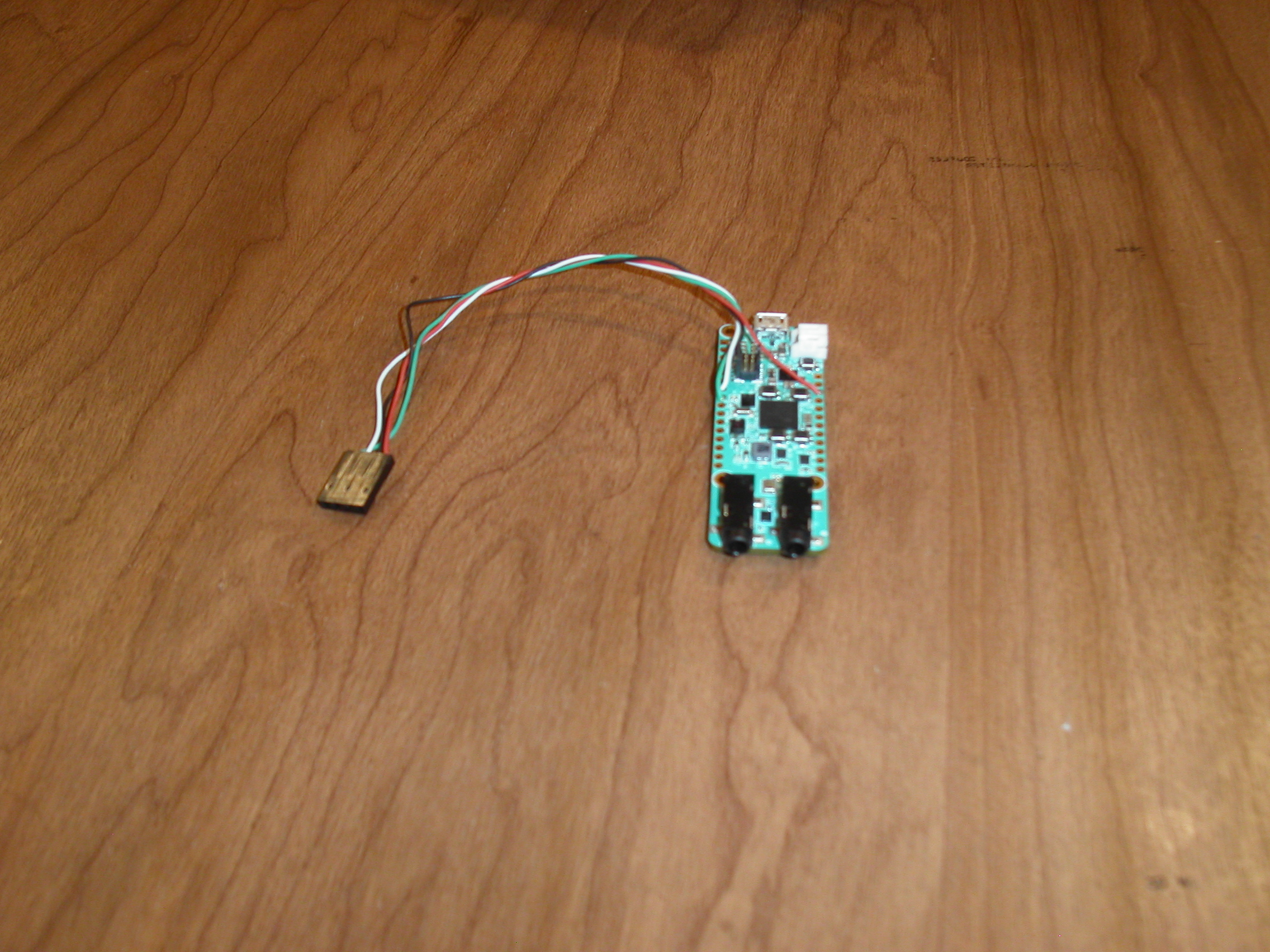
The MAX78000 feather board is programmed to output in JSON format over a serial connection to the P2 which will turn those words into cube values.
Here is a video of the Panel64 in action:
MAX78000 and Panel64
Here is the code to make those values show up from the P2:
You can see there is not much there. The font that I used was from the P1 so it was easy to find the character I wanted to display.
To fill most of the panel space I doubled the size of the characters which is simple enough to do.
Enjoy,
Mike