LED Progress Bar
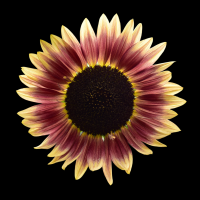
I am making an LED progress where the user holds a button down and every second they hold it an additional bar lights up. If they let go anywhere in that sequence before getting to the end, they all turn off. I immediately went to stacking if statements. Turns out there's a limit of 8 nested items! How do I do this more efficiently?
if ina[RedButton] == 1 outa[7..13] := %1000000 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1100000 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1110000 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1111000 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1111100 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1111110 waitcnt(clkfreq + cnt) if ina[RedButton] == 1 outa[7..13] := %1111111 RedFlag := 1 else outa[7..13] := %0000000 else outa[7..13] := %0000000 else outa[7..13] := %0000000 else outa[7..13] := %0000000 else outa[7..13] := %0000000 else outa[7..13] := %0000000
Comments
Reference: Propeller Manual v1.2 · Page 59
https://www.parallax.com/sites/default/files/downloads/P8X32A-Web-PropellerManual-v1.2.pdf#page59
eg:
while ina[RedButton] == 1
https://www.parallax.com/sites/default/files/downloads/P8X32A-Web-PropellerManual-v1.2.pdf#page188
You have to define "RedButton".
I haven't tested this.
Edit: Set LED pins as outputs. More RedFlag code.
SHL and SHR are assembly commands. DOH I'm off track now.
Hint: Don't hard-code pin numbers into your listing except into a CONstant definition that you can easily change later.
Update: Working code attached.
Make sure you set "BUTTON_PIN" to the pin you are using.
I switched the value of "FIRST_LED" temporarily to 16 and tested the code on a QuickStart board. It seemed to work as expected.
Cleaned out the debug code:
Assume button on P0 active low, leds on P7..P13 active high
This version inits the count to the same as the first led and clamps at the last led.
Couldn't resist.....some propbasic
Dave
" : DEMO 7 BEGIN 0 PIN@ IF DROP 7 $3F80 OUTCLR ELSE 13 MIN DUP HIGH 1+ THEN 1 s AGAIN ;"
It even looks so ....light on the keyboard. Efficient.
Or maybe my brain is just playing tricks on me, hard to tell
Yes doggiedoc, that video is exactly what I'm looking to do.
The idx variable increments each time around the loop, only up to 8, due to the <#, and always returns to zero if the button is up, due to the muliplication.
The outputs are set to the decoded value of idx, minus 1. Read |< idx as "idx to the power of 2".
You can flip the order by outa[lolight..hilight] instead of outa[hilight..lolight].
Excellent. The first thing I ever learned from Tracy Allen, I think it was in 2008, was scaling values. I learned to move the decimal point to the right to eliminate the decimal point, do integer calculations and then move the point back to the left.