PIR Motion Sensor with Piezo Buzzer and LEDs
I am trying to program a PIR motion sensor that will sense motion and turn on a piezo buzzer and LED light that are all connected on a Parallax Propeller. The code is written in C and used on Simple IDE. The code works to where the motion is sensed and turns on the buzzer and LED but I can't turn it off unless I unplug the circuit. I was thinking of adding a push button that will turn it off and reset it to the previous state. Any advice?
txt
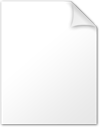
948B
Comments
/** * @brief Description of Project Here * @author * @date Month Day, Year * @version 1.0 * */ #include "simpletools.h" #include "stdbool.h" #define LED 2 #define BUZZER 4 #define PIR 5 // ------ Global Variables and Objects ------ int pir; bool volatile alert; // ------ Function Declarations ------ void led2(void *); // ------ Main Program ------ int main() { alert = false; cog_run(led2, 128); // Wait 20 to 30 seconds for PIR to calibrate or"warm up" print("Wait about 5 seconds for PIR to calibrate..........."); print("\r"); pause(5000); term_cmd(CLS); // Once the PIR detects movement, it will send a HIGH or a value of 1 out from its OUTPUT pin while (1) { pir = input(PIR); if (pir == 1) { alert = true; print("Intruder Alert!"); print("\r"); } else { if (alert) { print("All Clear!"); print("\r"); alert = false; } } pause(1000); term_cmd(CLS); } } // ------ Functions ------ void led2(void *par) { while (1) { if (alert) { freqout(BUZZER, 500, 1000); high(LED); pause(500); low(LED); } else { freqout(BUZZER, 0, 1000); } pause(500); } }
It compiles but I don't have your setup created to try it out.
Mike
Since the code that "iseries" provided was closer to what I think you want, I added the appropriate changes to it to implement a push-button to reset(turn-off) an active alarm. The code below compiles, but I haven't tested it on any hardware. Take a look at it and give it a try. Let us know how it goes...
/** * @brief Description of Project Here * @author * @date Month Day, Year * @version 1.0 * */ #include "simpletools.h" #include "stdbool.h" #define LED 2 #define BUZZER 4 #define PIR 5 #define PUSHBUTTON 8 // ------ Global Variables and Objects ------ int pir; bool volatile alert; bool volatile button_pressed; // ------ Function Declarations ------ void led2(void *); // ------ Main Program ------ int main() { alert = false; button_pressed = false; cog_run(led2, 128); // Wait 20 to 30 seconds for PIR to calibrate or"warm up" print("Wait about 5 seconds for PIR to calibrate..........."); print("\r"); pause(5000); term_cmd(CLS); // Once the PIR detects movement, it will send a HIGH or a value of 1 out from its OUTPUT pin while (true) { pir = input(PIR); if (pir == 1) { alert = true; print("Intruder Alert!"); print("\r"); } else { if (button_pressed) { print("All Clear!"); print("\r"); button_pressed = false; } } pause(1000); term_cmd(CLS); } } // ------ Functions ------ void led2(void *par) { while (1) { if (alert) { freqout(BUZZER, 500, 1000); high(LED); pause(500); low(LED); pause(500); if ( input(PUSHBUTTON) == 1 ) { button_pressed = true; alert = false; freqout(BUZZER, 0, 1000); } } else { freqout(BUZZER, 0, 1000); low(LED); } pause(100); } }
Just remove the freqout(BUZZER, 0, 1000);
Mike
Glad to hear it worked...