SimpleIDE Code Examples For The DS3231 RTC (Final Example Added 04/07/2020)

Hello Everyone
For those of you who have been following along, I have recently posted several project examples for the DS1302 RTC, which can be found here (forums.parallax.com/discussion/171402/simpleide-code-examples-for-the-ds1302-module-new-sample-posted-04-05-2020).
Instead of the DS1302 RTC, in this thread, I will be providing several project examples for the DS3231 RTC. The DS3231 RTC is supposedly a much more accurate timepiece.
Anyhow, I will basically be reusing the majority of the code from the previous thread, simply swapping out several of the functions to the I2C protocol.
Here is the code for the first example and I will be attaching a zipped project file below:
For those of you who have been following along, I have recently posted several project examples for the DS1302 RTC, which can be found here (forums.parallax.com/discussion/171402/simpleide-code-examples-for-the-ds1302-module-new-sample-posted-04-05-2020).
Instead of the DS1302 RTC, in this thread, I will be providing several project examples for the DS3231 RTC. The DS3231 RTC is supposedly a much more accurate timepiece.
Anyhow, I will basically be reusing the majority of the code from the previous thread, simply swapping out several of the functions to the I2C protocol.
Here is the code for the first example and I will be attaching a zipped project file below:
// Set_The_DS3231.c #include "simpletools.h" ////////////////////////////////////////////////////////////////// // OVERVIEW // This code is intended to set your DS3231 time and date according // the time it is complied and run on your PC, and should be run // seperately from your other firmware. ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// // Step #1 // Before running this code, set your PC time clock to the highest // accuracy that you can possibly achieve. ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// // Step #2 - One time setting // Define the I/0 pin going from your Propeller chip to the DS3231 // SDA pin. #define DS3231_SDA 14 ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// // Step #3 - One time setting // Define the I/0 pin going from your Propeller chip to the DS3231 // SCL pin. #define DS3231_SCL 15 ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// // Step #4 - Adjust as necessary // Adjust for the difference between compile time and runtime. // This is the number of seconds between the compilation time and // the amount of time that it takes to write the date and time to // the DS3231 registers. #define OFFSET_SECONDS 4 ////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////// // Step #5 - Run with terminal // If your DS3231 is inaccurate compared to your PC's time clock, // then repeat Step #4 and Step #5 until the DS3231 time and your // PC's time clock are synchronized. ////////////////////////////////////////////////////////////////// int second; int minute; int hour; int weekday; int month; int date; int year; int second_bcd; int minute_bcd; int hour_bcd; int weekday_bcd; int month_bcd; int date_bcd; int year_bcd; i2c *pBus; // Convert integer to binary coded decimal int DecimalToBCD(int Decimal) { return (((Decimal / 10) << 4) | (Decimal % 10)); } // Get the weekday... 0 = Sunday, 1 = Monday, etc. int GetTheWeekday() { int Weekday; int Month; int Date; int Year; Month = month; Date = date; Year = year; Weekday = ((Date += (Month < 3 ? Year-- :(Year - 2))), (23 * Month / 9 + Date + 4 + Year / 4 - Year / 100 + Year / 400)) % 7; return Weekday; } void Set_DS3231_Time() { i2c_start(pBus); i2c_writeByte(pBus, 0xd0); i2c_writeByte(pBus, 0x00); i2c_writeByte(pBus, second_bcd); i2c_writeByte(pBus, minute_bcd); i2c_writeByte(pBus, hour_bcd); i2c_writeByte(pBus, weekday_bcd); i2c_writeByte(pBus, date_bcd); i2c_writeByte(pBus, month_bcd); i2c_writeByte(pBus, year_bcd); i2c_stop(pBus); } void Get_DS3231_Time() { i2c_start(pBus); i2c_writeByte(pBus, 0xd0); i2c_writeByte(pBus, 0); i2c_start(pBus); i2c_writeByte(pBus, 0xd0 | 1); second = i2c_readByte(pBus, 0); minute = i2c_readByte(pBus, 0); hour = i2c_readByte(pBus, 0); weekday = i2c_readByte(pBus, 0); date = i2c_readByte(pBus, 0); month = i2c_readByte(pBus, 0); year = i2c_readByte(pBus, 1); i2c_stop(pBus); } int main() { const char *pCharacterPointer; char chMonth[4]; char chDate[3]; char chYear[5]; char chWeekday[2]; char chHour[3]; char chMinute[3]; char chSecond[3]; int previous_second; i2c_open(pBus, DS3231_SCL, DS3231_SDA, 0); if(strstr(__DATE__, "Jan") != NULL) { month = 1; } else if(strstr(__DATE__, "Feb") != NULL) { month = 2; } else if(strstr(__DATE__, "Mar") != NULL) { month = 3; } else if(strstr(__DATE__, "Apr") != NULL) { month = 4; } else if(strstr(__DATE__, "May") != NULL) { month = 5; } else if(strstr(__DATE__, "Jun") != NULL) { month = 6; } else if(strstr(__DATE__, "Jul") != NULL) { month = 7; } else if(strstr(__DATE__, "Aug") != NULL) { month = 8; } else if(strstr(__DATE__, "Sep") != NULL) { month = 9; } else if(strstr(__DATE__, "Oct") != NULL) { month = 10; } else if(strstr(__DATE__, "Nov") != NULL) { month = 11; } else if(strstr(__DATE__, "Dec") != NULL) { month = 12; } pCharacterPointer = strstr(__DATE__, " "); if(pCharacterPointer != NULL) { pCharacterPointer += 2; strncpy(chDate, pCharacterPointer, 1); chDate[1] = '\0'; pCharacterPointer += 2; } else { pCharacterPointer = strstr(__DATE__, " "); if(pCharacterPointer != NULL) { pCharacterPointer += 1; strncpy(chDate, pCharacterPointer, 2); chDate[2] = '\0'; pCharacterPointer += 3; } } sprint(chMonth, "%d", month); strncpy(chYear, pCharacterPointer, 4); chYear[4] = '\0'; strncpy(chHour, __TIME__, 2); chHour[2] = '\0'; pCharacterPointer = strstr(__TIME__, ":"); pCharacterPointer += 1; strncpy(chMinute, pCharacterPointer, 2); chMinute[2] = '\0'; pCharacterPointer += 3; strncpy(chSecond, pCharacterPointer, 2); chSecond[2] = '\0'; month = atoi(chMonth); date = atoi(chDate); year = atoi(chYear); hour = atoi(chHour); minute = atoi(chMinute); second = atoi(chSecond); weekday = GetTheWeekday(); sprint(chWeekday, "%d", weekday); second = second + OFFSET_SECONDS; year = year - 2000; weekday = weekday + 1; print("Compile Date: "); print("%s/", chMonth); print("%s/", chDate); print("%s\n", chYear); print("Compile Time: "); print("%s:", chHour); print("%s:", chMinute); print("%s\n\n", chSecond); second_bcd = DecimalToBCD(second); minute_bcd = DecimalToBCD(minute); hour_bcd = DecimalToBCD(hour); weekday_bcd = DecimalToBCD(weekday); month_bcd = DecimalToBCD(month); date_bcd = DecimalToBCD(date); year_bcd = DecimalToBCD(year); Set_DS3231_Time(); previous_second = 0; while(1) { Get_DS3231_Time(); if(second != previous_second) { print("DS3231 Time: "); if(weekday == 1) { print("Sunday"); } else if(weekday == 2) { print("Monday"); } else if(weekday == 3) { print("Tuesday"); } else if(weekday == 4) { print("Wednesday"); } else if(weekday == 5) { print("Thursday"); } else if(weekday == 6) { print("Friday"); } else if(weekday == 7) { print("Saturday"); } print(" "); putHexLen(month,2); print("/"); putHexLen(date,2); print("/"); putHexLen(year,2); print(" "); putHexLen(hour,2); print(":"); putHexLen(minute,2); print(":"); putHexLen(second,2); print("\n"); } previous_second = second; } }
zip
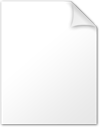
801K
Comments
To be honest, it doesn't matter much to me at this moment, because I am only interested in timestamps. However, there may come such a time when it will be important to me.
As for everyone else, here is the code for the second example. I will also be attaching a zipped project file below.
DUH
Anyhow, here is the code for the last example and I have attached a zipped project file below.