Status Lights to Indicate Activity of Cogs
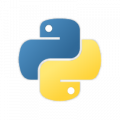
in Propeller 1
Hello, I am making a spin program to turn on and off the VGA LEDs on the PropBOE according to the active status of each cog.
Cog 0 starts up, Sets pins 0..7 as outs, then low, then turns on LED 0 to indicate that it is active, then generates two random numbers. Cog 0 then starts a cog according to random number a, then stops a cog according to random number b, also setting the corresponding LED low. As Each Cog is activated, it turns on an LED to indicate it's activity, then generates the random numbers to start and stop the other cogs.
What I need help with is the syntax of Coginit and the random operator, Thanks!
Cog 0 starts up, Sets pins 0..7 as outs, then low, then turns on LED 0 to indicate that it is active, then generates two random numbers. Cog 0 then starts a cog according to random number a, then stops a cog according to random number b, also setting the corresponding LED low. As Each Cog is activated, it turns on an LED to indicate it's activity, then generates the random numbers to start and stop the other cogs.
What I need help with is the syntax of Coginit and the random operator, Thanks!
Comments
Look in the Propeller Manual for the syntax of COGINIT and the random operation.
EDIT:
I see it now.
Only Cog 0 wil run the Private method Init.
You can use a "lock" to reserve access to a shared resource to fix this problem. There are examples in the Propeller Manual for the LOCKNEW, LOCKSET, LOCKCLR, and LOCKRET starting at pg 120.
EDIT:
I changed the Private method "Init" to a Public method, AND IT WORKED!!
All but the LEDs, that is.
What do I do about the LEDs only being on for a moment? I would like them to be on for the entire duration of the cog's active status.
OUTA[COGID] := 1 { Set corresponding OUTA bit to high }
DIRA[COGID] := 1 { and enable I/O pin output state }
Whenever the cog stops, whether the cog stops itself or it's stopped by another cog, the LED will turn off because a stopped cog zeroes all of its control registers including DIRA and OUTA.
Then you'd have:
You can use an array for MyID and make cogStack big enough for 8 cog stacks. Usually I'd allow 100 longs, but that's way more than you'd really need for one cog. Try 50 per cog with VAR LONG cogStack[400]
You can put the IF in a REPEAT loop with i going from 1 to 7. You'd have
MyID[ I ] := COGNEW(cogLoop,@cogStack+I*200)+1
You probably will want to put delays in the code for all but the initialization cog so the initialization cog can finish its work before one of the other cogs reuses the initialization cog before it's done.
CON ms = CLKFREQ / 1000
This gives the number of system clock cycles for a millisecond and adjusts it as needed when a program is recompiled with a different system clock speed. Then you can do:
WAITCNT(CNT+150*ms)
to get a 150ms pause. The total delay in clock cycles must be less than 2^31 or you'll run into problems with overflow since calculations are done with 32 bit numbers. Be careful with delays on the order of tens of microseconds or less. That's getting near the execution time for primitive Spin operations. Such situations probably should be handled using assembly language.
You can also define
CON us = CLKFREQ / 1000000
or
CON sec = CLKFREQ