Writing code is an art form

Especially when it's this colorful. JetBrains just released the CLion 2016.3 EAP (that's their way of saying "beta") which includes "semantic highlighting". That means CLion now renders the following code block in one of two ways, depending on your theme settings:
Baseline
Light theme
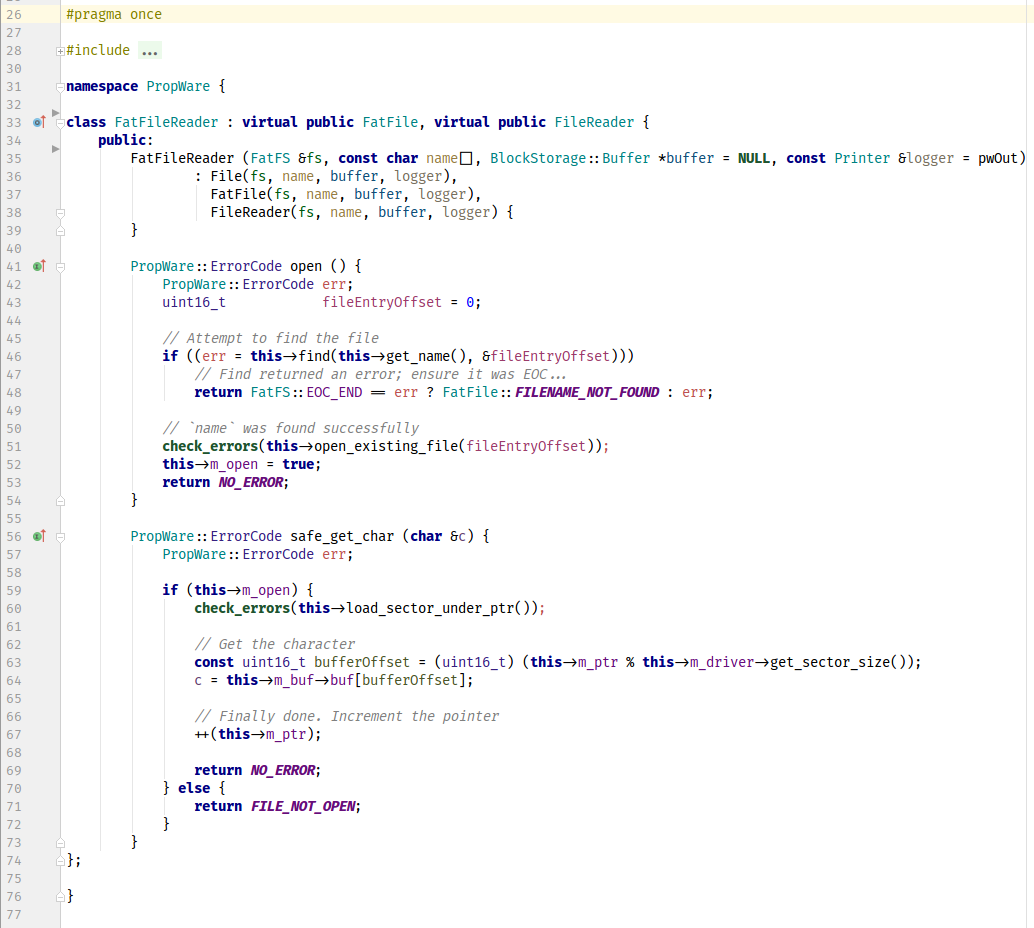
Dark theme
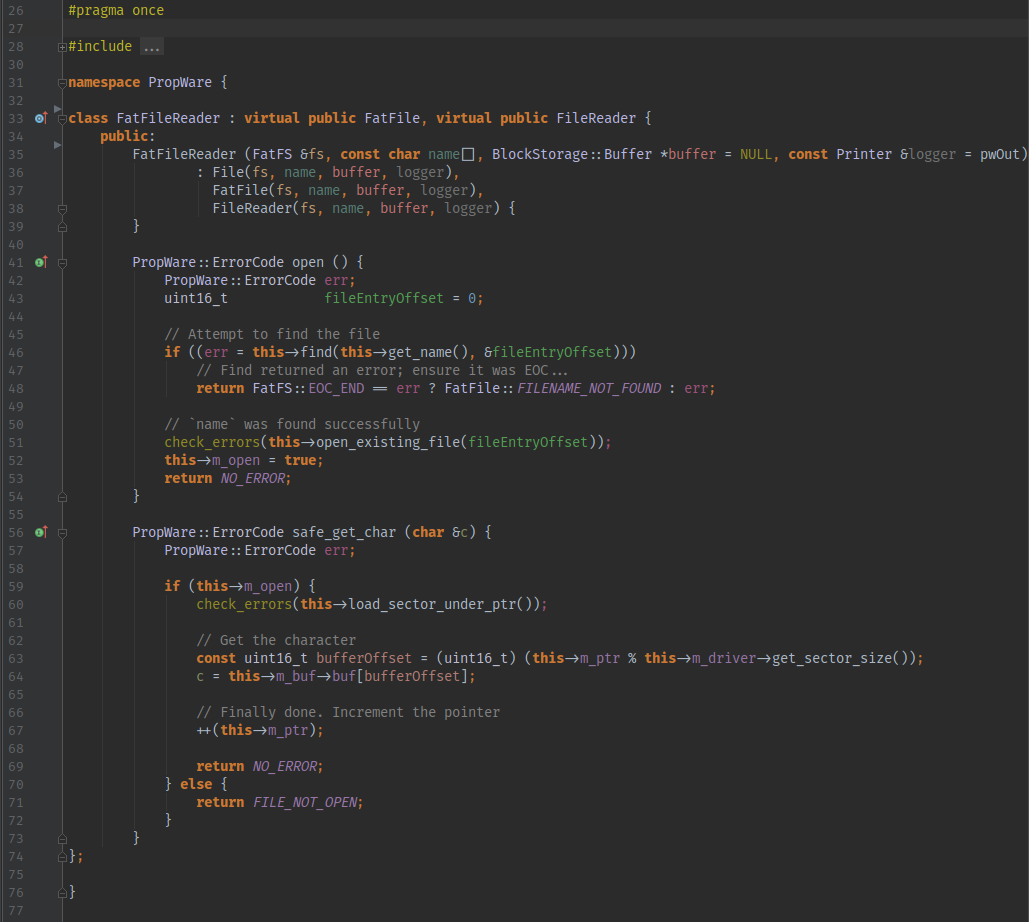
You'll notice that variables in a function scope each have their own unique color assigned to them. The variables "fs", "name", "buffer", and "logger" are each given their own unique syntax coloring in the constructor. This makes it even easier to spot their usage throughout the function. The same goes for the variables "c", "err", and "bufferOffset" in the safe_get_char function.
I imagine there are number of folks here that might think this is just too much color, and that it is distracting. I think I like it though!
Baseline
#pragma once #include <PropWare/filesystem/filereader.h> #include <PropWare/filesystem/fat/fatfile.h> namespace PropWare { class FatFileReader : virtual public FatFile, virtual public FileReader { public: FatFileReader (FatFS &fs, const char name[], BlockStorage::Buffer *buffer = NULL, const Printer &logger = pwOut) : File(fs, name, buffer, logger), FatFile(fs, name, buffer, logger), FileReader(fs, name, buffer, logger) { } PropWare::ErrorCode open () { PropWare::ErrorCode err; uint16_t fileEntryOffset = 0; // Attempt to find the file if ((err = this->find(this->get_name(), &fileEntryOffset))) // Find returned an error; ensure it was EOC... return FatFS::EOC_END == err ? FatFile::FILENAME_NOT_FOUND : err; // `name` was found successfully check_errors(this->open_existing_file(fileEntryOffset)); this->m_open = true; return NO_ERROR; } PropWare::ErrorCode safe_get_char (char &c) { PropWare::ErrorCode err; if (this->m_open) { check_errors(this->load_sector_under_ptr()); // Get the character const uint16_t bufferOffset = (uint16_t) (this->m_ptr % this->m_driver->get_sector_size()); c = this->m_buf->buf[bufferOffset]; // Finally done. Increment the pointer ++(this->m_ptr); return NO_ERROR; } else { return FILE_NOT_OPEN; } } }; }
Light theme
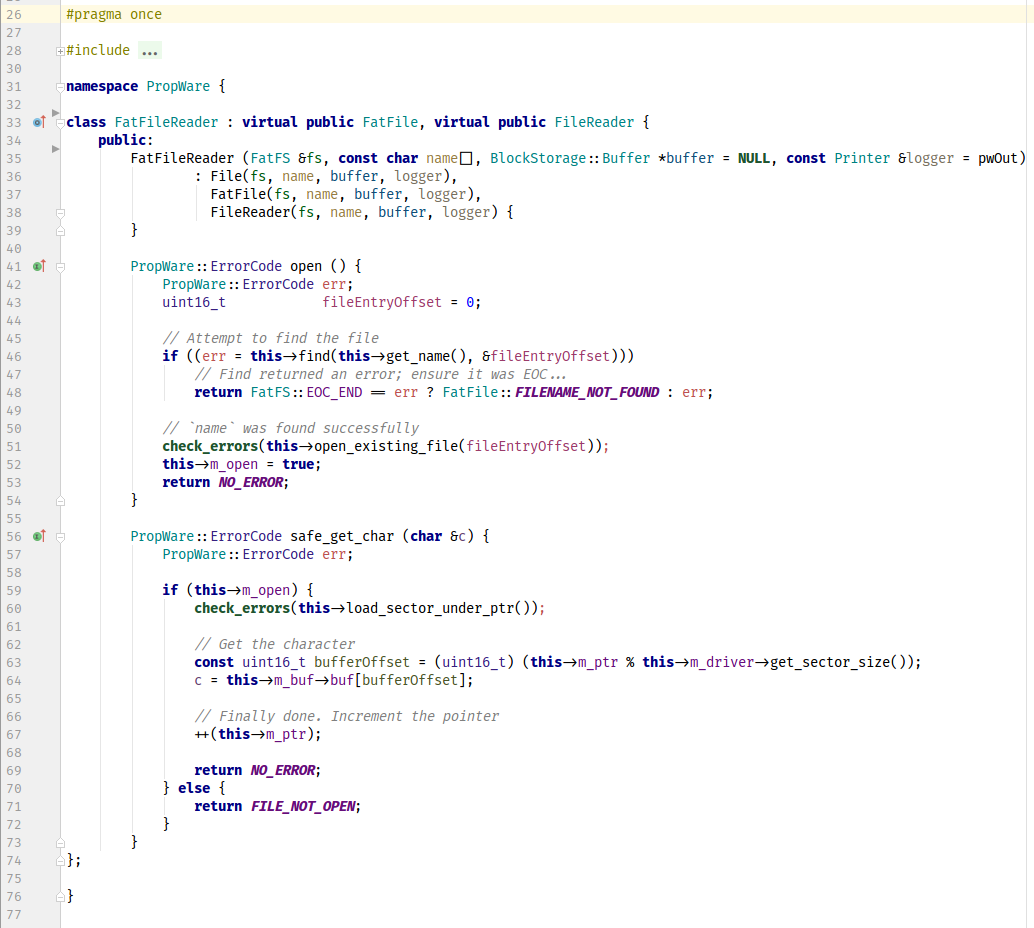
Dark theme
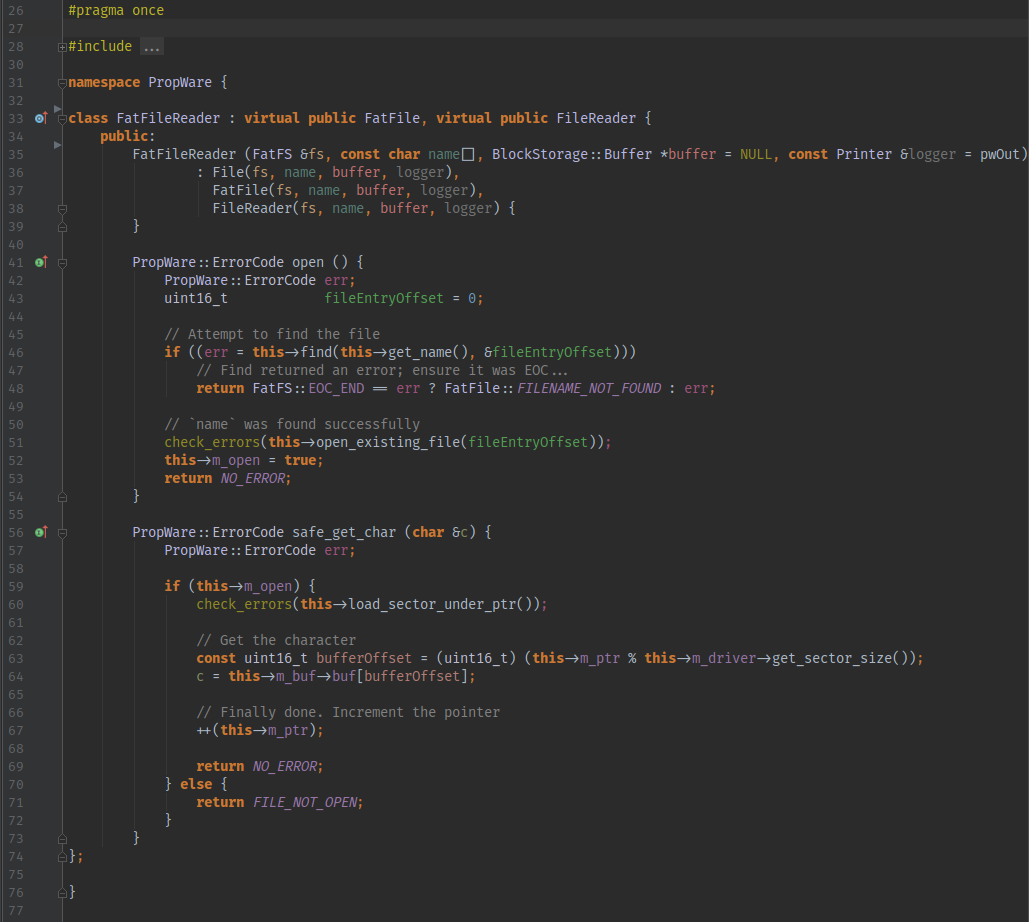
You'll notice that variables in a function scope each have their own unique color assigned to them. The variables "fs", "name", "buffer", and "logger" are each given their own unique syntax coloring in the constructor. This makes it even easier to spot their usage throughout the function. The same goes for the variables "c", "err", and "bufferOffset" in the safe_get_char function.
I imagine there are number of folks here that might think this is just too much color, and that it is distracting. I think I like it though!
Comments
JetBrains already responded to my question regarding this actually. Anastasia says:
https://blog.jetbrains.com/clion/2016/09/clion-opens-2016-3-eap/#comment-30724
http://forums.parallax.com/discussion/165006/bs2-haiku
Yes, that thread is quite inspiring
And, until an IDE comes around that has a Vi-style interface, I'll never be able to use an IDE again. The editing speed gain that editors like Vim and Kakoune give, with the concept of keystrokes as a text editing language, is just too significant to make the niceties of other editors that don't have these capabilities remotely worth it.
I consider that a feature
Have you seen JetBrain's IdeaVim? Heater would appreciate that it's also opensource https://plugins.jetbrains.com/plugin/164
https://github.com/JetBrains/ideavim
Perhaps we have had this discussion before, but, as far as I can tell, this JetBrains thing is closed source. I don't see any point in having an open source vi/vim mode for it.
It's really not going to help me when I ssh into some server and want to hack stuff.
Meanwhile, the open source MS Visual Studio Code IDE has a vim extension.
Get that, I'm using an MS product. And even liking it. That's a first.
We have, but this is the Internet, and the Internet is for por... repeating yourself.
The C/C++ parts of CLion are closed source, but the platform is open, including numerous language agnostic plugins (IdeaVim, Git, Subversion, etc), as well as the Java and Python plugins.
Depends what language you want to use for hacking said stuff. I know PyCharm lets you edit remote files directly in the IDE, without having to execute a SFTP/SCP command before/after to keep them in sync. Every time you hit CTRL + S or let the IDE autosave, it does the sync for you. Then you get all the features of an IDE with quick turnaround of remote editing. I would bet (but haven't confirmed) that it's also available in their HTML/CSS/JavaScript IDE called WebStorm.
That whole MS VS Code thing is pretty cool... I'll give you that
If it's actual code changes I want to do then that gets developed and tested locally. It ends up on our server via git. Using github or bit bucket.
Now, I don't mind whatever tool one uses to develop code. The only requirement is that the resulting code can be worked on by others, built and deployed, without any requirement for closed source tools.