Spin to PASM converter (still very early stages)
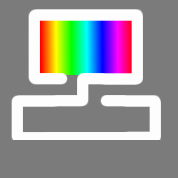
in Propeller 1
I've been doing some work on adding PASM output to spin2cpp, so it can be used to produce PASM directly (instead of having to produce C code and then use PropGCC to create PASM). The advantage would be much more readable output, and also it will serve to validate the idea of putting different back ends on spin2cpp. We've talked in the past about using the LLVM code generator when it becomes available.
The custom code generator will not produce code of the quality of, say, GCC or LLVM, but it does do some basic optimizations and the result is not too bad. I was able to actually build and run my first program completely with spin2cpp (no PropGCC needed). It's a blinking LED program, of course. The input source code (foo.spin):
It is still very early days, so I don't have a binary release yet -- ATM this is for fairly experienced programmers only. The code resides on the "spin2pasm" branch of https://github.com/totalspectrum/spin2cpp.
There's still a lot to do (e.g. the VAR section is ignored at the moment) but I was hoping for some kind of general feedback on what kinds of features would be useful in this:
(1) Should the output use only COG memory (so it can only hold very small amounts of data + code), or does it make sense to put the VAR data into HUB memory the way PropGCC does?
(2) Is LMM mode desirable? I presume so.
(3) How important is P2 support?
(4) Right now all locals are placed in COG memory rather than on a stack in HUB. This produces much more readable (and efficient) code, but it means that (a) recursive functions are not allowed, and (b) COG memory gets used up quickly.
I guess there are some interesting trade-offs between making the code readable and useful for learning versus making a production ready compiler. Any thoughts on which way this tool would be used?
Finally, once the front end (spin parser) and back end (code generator) are cleanly separated, then in theory other front ends like Basic, Pascal, etc., could be added. I don't know how useful this would actually be, since the back end is not going to optimize as well as GCC or LLVM. On the other hand I think it will be much lighter weight and easier to use, so perhaps it would still be useful to people?
Eric
The custom code generator will not produce code of the quality of, say, GCC or LLVM, but it does do some basic optimizations and the result is not too bad. I was able to actually build and run my first program completely with spin2cpp (no PropGCC needed). It's a blinking LED program, of course. The input source code (foo.spin):
CON _clkmode = xtal1+pll16x _clkfreq = 80_000_000 pin = 15 PUB start | pause pause := (_clkfreq/2) DIRA[pin] := 1 ' set as output OUTA[pin] := 1 ' turn it on repeat waitcnt(CNT + pause) !OUTA[pin]and the output (unmodified) produced by spin2cpp --asm foo.spin:
DAT org 0 foo_start mov foo_start_pause_, imm_40000000_ or DIRA, imm_32768_ or OUTA, imm_32768_ L_001_ mov foo_start_tmp001_, CNT add foo_start_tmp001_, foo_start_pause_ waitcnt foo_start_tmp001_, #0 xor OUTA, imm_32768_ jmp #L_001_ L_002_ foo_start_ret ret foo_start_pause_ long 0 foo_start_tmp001_ long 0 imm_32768_ long 32768 imm_40000000_ long 40000000This can then be compiled into foo.binary via "spin2cpp --binary --dat foo.pasm".
It is still very early days, so I don't have a binary release yet -- ATM this is for fairly experienced programmers only. The code resides on the "spin2pasm" branch of https://github.com/totalspectrum/spin2cpp.
There's still a lot to do (e.g. the VAR section is ignored at the moment) but I was hoping for some kind of general feedback on what kinds of features would be useful in this:
(1) Should the output use only COG memory (so it can only hold very small amounts of data + code), or does it make sense to put the VAR data into HUB memory the way PropGCC does?
(2) Is LMM mode desirable? I presume so.
(3) How important is P2 support?
(4) Right now all locals are placed in COG memory rather than on a stack in HUB. This produces much more readable (and efficient) code, but it means that (a) recursive functions are not allowed, and (b) COG memory gets used up quickly.
I guess there are some interesting trade-offs between making the code readable and useful for learning versus making a production ready compiler. Any thoughts on which way this tool would be used?
Finally, once the front end (spin parser) and back end (code generator) are cleanly separated, then in theory other front ends like Basic, Pascal, etc., could be added. I don't know how useful this would actually be, since the back end is not going to optimize as well as GCC or LLVM. On the other hand I think it will be much lighter weight and easier to use, so perhaps it would still be useful to people?
Eric
Comments
I like Eric's idea of creating a Spin-to-PASM compiler. This would allow Spin to execute much faster than the current method of using byte codes and an interpreter. There are a lot of trade-offs in how this is implemented. A truly compatible implementation would need to use the stack just like the interpreter does. It's not clear how much faster this would be than the interpreter version since it would need an LMM interpreter to run from Hub memory on the P1. However, it would avoid the need to decode byte codes.
Using registers instead of the stack will improve performance. I do wonder if spin2cpp is a better approach since the optimizer in the C compiler can be used to improve performance even further.
P2 support with hubexec would also be amazing.
I imagine that would be a lot of work though.
This looks great.
Can you add the Source as comments in the ASM ?
Do you create any sort of Debug file ?
A weakness in Spin2cpp is the final code is going to have so many steps, original source code step-debug is going to be very hard.
SDCC has an interesting approach and IIRC they embed the Source file path and line numbers in the ASM, so that simple debug can work-backwards to HLL lines.
Spin can always run as normal Spin, so I would focus on 'small' here to act as a bridge between Spin and PASM for new users.
ie they code in Spin, then pick the bits that need to be faster.
This could also be a great way to get rapid P2 Spin/PASM, which I would call very important.
At the moment it just produces PASM text, which can then be compiled into a raw binary. What sort of debug file were you thinking of? The PropGCC .elf format would be an obvious candidate, but I'm not sure how many people actually use gdb on the Propeller.
If you use the -g flag (for debug) then the C/C++ code output by spin2cpp contains references to the original .spin file. If you debug the compiled .elf with gdb you should see the original Spin source in gdb.
Of course that doesn't apply to the --asm variant ("spin2pasm"). For that we could output DWARF debug info and assemble with GAS. But for now I'd like to keep spin2pasm self contained (not requiring external programs).
Thanks for your suggestions!
I think you're right, David -- P2 hubexec shouldn't be too hard to support. I've tried to write the code with eventual P2 support in mind, but I don't have a working P2 setup yet so I've been focused on the P1 for now. At the source level the two architectures seem not too different, although obviously there will be some tweaks required to take advantage of the P2. The biggest pain will probably be doing PASM -> binary translation for P2; we'll want that to process P2 DAT sections.
re:Spin to PASM converter
Cool! Your doing great work. For us that are not PASM experts all you need to do is look at the P2 doc's once and you will soon realize that a Spin to PASM converter will be very helpful on the P2
Chrome refuses to download the latest P2 package for me because it thinks there's a virus in it. I guess it's probably a false positive, since nobody else has noticed it, but I haven't had the time right now to figure out a work-around. I do plan to try to get it working, but right now I thought I'd get P1 PASM going first since I'm more familiar with it.
One thing that worked was to open it with Google Drive, which is connected to the download page somehow...
Then, I could download from Google Drive...
re:Chrome refuses to download the latest P2 package for me because it thinks there's a virus in it.
You can usually click on the icon for your antivirus program and disable it temporarily. For example on one system I have Avast and I can disable it for 10 minutes, 1 hour etc.
If chrome is still blocking it just use another web browser ( IE, Firefox etc)
What ever happened to the Spin to PASM converter?
I need that bad boy.
Are you reading the same thread I am ?
I can see this :
"right now I thought I'd get P1 PASM going first since I'm more familiar with it."
Does not look one bit like 'drifted of to P2-land' to me ?
Makes sense to do it for P1 first, as many eyes can scan P1 ASM very quickly.
You were very helpful.
All: I'll be posting a preview version of the converter in a new thread shortly
http://david.zemon.name:8111/project.html?projectId=Spin2Cpp&guest=1