joystick driven BOEBot using Xbee in spin
I had built a propeller BOEBot from the tutorialhttp://learn.parallax.com/node/162. I then went through the getting started with XBee RF modules text http://www.parallax.com/product/32450 including chapter 7 that uses an mx2125 accelerometer on a board to navigate the robot by tilting the board. When I saw a recent Projects forum post by Vale T. that used a 2 axis joystick to navigate an activity bot with XBee I was wondering If I could do the same with my BOEBot. His code was in c with which I am not familiar. So I thought I would try writing spin code that would accomplish my purpose. The two codes at the end of this post is my attempt. It worked for my BOEBot and should work for an activity BOT also. I mounted the joystick (parallax 27800) on an activity board but it should work on any board. If you follow the steps below it should work.
1. Build the joystick on an activity board or BOE as shown at: http://learn.parallax.com/KickStart/27800 and download the propeller demo code there also. Put an XBee on the board containing the joystick being careful to orient it correctly. A small diagram next to the XBEee socket on the board will show how it goes into the socket. Propeller board I/O pin 6 should be connected to XBee pin DI and propeller board I/O pin 7 should connect to XBee pin DO. See image below.
[img][/img]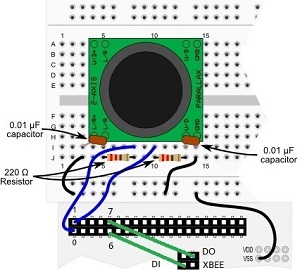
2. Run the joystick spin demo and check out the raw RC time values displayed on PST. I ran the demo at: http:// learn.parallax.com/KickStart/27800 which gave me the raw RC time data. It was 0 to 230 with 114 as the value with joystick centered. To get both x axis and y axis readings to agree at these values I played around with the 220 ohm resistors and ending up replacing one with a 100 ohm and the other with a 260 ohm. With these values in mind I defined the cases where the wheels were set to zero (BOE-Bot) as having raw RC values (joystick) between 100 and 130. I set the max wheel values to 64 rather than 100 so the steering in turns was smoother and no “wheelies” happened between forward - backward changes. Make appropriate adjustments to your raw data as needed.
3. Build the BOE-Bot and download the code at: [url] http://learn.parallax.com/node/277[/url] the BOEBot tutorial.
4. Insert the XBEE module in the socket on the BOE-Bot, making sure it is oriented correctly ( not pointing the same way as on an activity board). Download XBEE_Object.spin and see diagram and code at: http://learn.parallax.com/node/362XBee tutorial .
5.Copy the two code files that follow in this post, paste them into a blank propeller editor window , and save them as spin files in the same folder. That folder must also contain all the files in the OBJ section of both: FullDuplexSerial.spin, RCTime.spin (both in propeller tools), PropellerBoardOfEducation.spin, PropellerBoeBotServoDrive.spin, Timing .spin (these three downloaded from the BOEBot tutorial), and XBee_object.spin ( from XBee tutorial)
Here is spin code for navigating the propeller BOEBot with a 2 axis joy stick.
This is the spin code for the joy stick board.
This is the spin code for the propeller BOEBot
1. Build the joystick on an activity board or BOE as shown at: http://learn.parallax.com/KickStart/27800 and download the propeller demo code there also. Put an XBee on the board containing the joystick being careful to orient it correctly. A small diagram next to the XBEee socket on the board will show how it goes into the socket. Propeller board I/O pin 6 should be connected to XBee pin DI and propeller board I/O pin 7 should connect to XBee pin DO. See image below.
[img][/img]
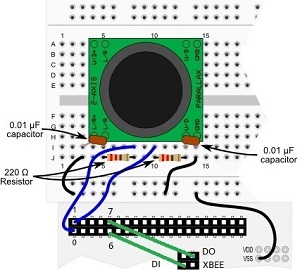
2. Run the joystick spin demo and check out the raw RC time values displayed on PST. I ran the demo at: http:// learn.parallax.com/KickStart/27800 which gave me the raw RC time data. It was 0 to 230 with 114 as the value with joystick centered. To get both x axis and y axis readings to agree at these values I played around with the 220 ohm resistors and ending up replacing one with a 100 ohm and the other with a 260 ohm. With these values in mind I defined the cases where the wheels were set to zero (BOE-Bot) as having raw RC values (joystick) between 100 and 130. I set the max wheel values to 64 rather than 100 so the steering in turns was smoother and no “wheelies” happened between forward - backward changes. Make appropriate adjustments to your raw data as needed.
3. Build the BOE-Bot and download the code at: [url] http://learn.parallax.com/node/277[/url] the BOEBot tutorial.
4. Insert the XBEE module in the socket on the BOE-Bot, making sure it is oriented correctly ( not pointing the same way as on an activity board). Download XBEE_Object.spin and see diagram and code at: http://learn.parallax.com/node/362XBee tutorial .
5.Copy the two code files that follow in this post, paste them into a blank propeller editor window , and save them as spin files in the same folder. That folder must also contain all the files in the OBJ section of both: FullDuplexSerial.spin, RCTime.spin (both in propeller tools), PropellerBoardOfEducation.spin, PropellerBoeBotServoDrive.spin, Timing .spin (these three downloaded from the BOEBot tutorial), and XBee_object.spin ( from XBee tutorial)
Here is spin code for navigating the propeller BOEBot with a 2 axis joy stick.
This is the spin code for the joy stick board.
{{ Reads 2 axis joystick, sends drive information to bot at address 1.}} CON _clkmode = xtal1 + pll16x _xinfreq = 5_000_000 ' Set pins and Baud rate for XBee comms XB_Rx = 7 ' XBee Dout XB_Tx = 6 ' XBee Din XB_Baud = 9600 MY_Addr = 0 DL_Addr = 1 ' bot address 'Constants used by joystick Object Xout_pin = 0 'Propeller pin joystick X Yout_pin = 1 'Propeller pin joystick Y VAR long drive, UD, LR byte Stack[100] OBJ pst : "FullDuplexSerial" XB : "XBee_Object" rc : "RCTime" PUB Start ' Configure XBee XB.start(XB_Rx, XB_Tx, 0, XB_Baud) ' Initialize comms for XBee XB.AT_Init ' Fast AT updates XB.AT_ConfigVal(string("ATMY"), MY_Addr) XB.AT_ConfigVal(string("ATDL"), DL_Addr) cognew(sendcontrol,@Stack) ' start cog to accept incoming data Pub SendControl drive :=5 repeat rc.rctime(0, 1, @UD) 'RC time joystick x axis rc.rctime(1, 1, @LR) 'RC time joystick y axis IF UD < 100 drive := 1 ' 1 is forward IF UD > 130 drive := 2 '2 is backward IF LR > 130 and UD < 100 drive := 3 '3 is left going forward IF LR < 100 and UD <100 drive := 4 '4 is right going forward IF UD >100 AND UD <130 AND LR > 100 AND LR <130 drive := 5 '5 is stop IF LR > 130 and UD > 130 drive := 6 '6 is left going backward IF LR < 100 and UD > 130 drive := 7 '7 is right going backwards XB.DEC(drive) ' send drive value to BOEBot XB.tx(13)
This is the spin code for the propeller BOEBot
{{ Accept control information from node 0 joystick to drive propeller BOEbot.}} CON _clkmode = xtal1 + pll16x _xinfreq = 5_000_000 ' I/O and Baud rate for XBee comms XB_Rx = 6 ' XBee Dout XB_Tx = 7 ' XBee Din XB_Baud = 9600 ' XBee addresses DL_Addr = $ffff ' Send data to this address (both controller) MY_Addr = 1 ' This units address ' Servo outputs Left = 14 Right = 15 VAR long drive OBJ XB : "XBee_Object" system : "Propeller Board of Education" servo : "PropBOE-Bot Servo Drive" time : "Timing" PUB Start ' Initialize XBee Comms and stop wheels XB.Start(XB_Rx, XB_Tx ,0, XB_Baud) servo.wheels(0,0) ' Enable XBee for fast configation changes & ' set MY and DL (destinaton) address. XB.AT_Init XB.AT_ConfigVal(string("ATMY"), MY_Addr) XB.AT_ConfigVal(string("ATDL"), DL_Addr) repeat XB.RXFlush 'clear data drive := XB.RXDec 'accept drive values from node 0 case drive 1: 'forward servo.wheels(64, 64) 2: 'reverse servo.wheels(-64, -64) 3: 'right turn going forward servo.wheels(64, 0) 4: 'left turn going forward servo.wheels(0, 64) 5: 'stop servo.wheels(0,0) 6: 'right turn going backwards servo.wheels(-64,0) 7: 'left turn going backwards servo.wheels(0,-64)
Comments
Hello. I am trying to find OBJECT "Propeller Board of Education" and OBJECT "Propeller-Bot servo Drive" I click on the http// and page not found. Is there another way to find the OBJECT. I have tryed the Parallax OBJECT exchange and I can not find anything. Thank you. 22 jan 2025(wen).
Nice project Patrick.
Other useful references for Xbee are;
Parallax 122-32450 Xbee tutorial
and our very own JonnyMac; Nuts and Volts Nov2017.
Hi!
Some of those old downloads can be found here:
https://www.parallax.com/download/?search=boe-bot
And the lessons here:
https://web.archive.org/web/20170602014631/http://learn.parallax.com/tutorials/robotics-propeller-boe-bot
As for the kickstarts...
https://learn.parallax.com/public_downloads/microcontroller-kickstarts/
https://learn.parallax.com/public_downloads/spin-tutorials-and-projects/