Trouble with floats in a cog
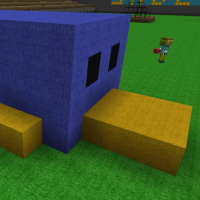
Can someone tell me what is wrong with my code here?
I have three variables. An int and two floats.
The int and one float increment in a cog.
The other float increments in the main program.
I expect to see all three increment on each run.
The int in the cog increments fine.
The float in the main program increments fine.
However, the float in the cog resets to zero periodically.
Why is this?
Here is a screenshot of the problem:
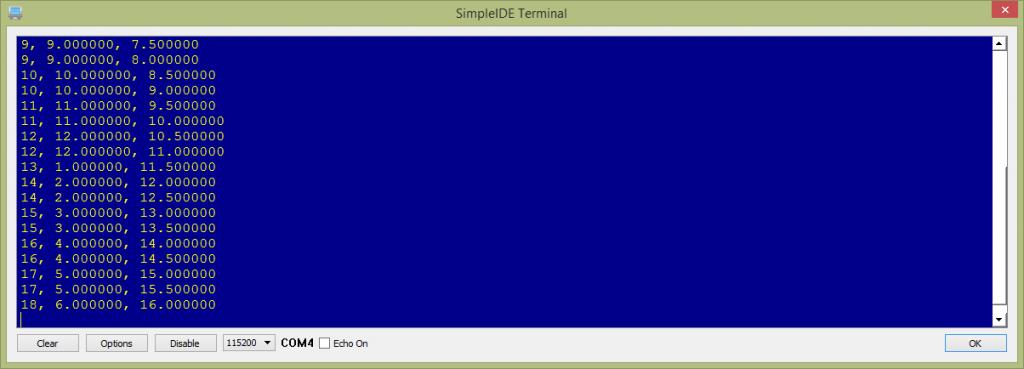
Notice how the globalFloat resets to 0 while the others keep incrementing. This happens repeatedly as the program runs.
P.S. Is this the right place to post this? It is C, not CPP, but I couldn't find another area that seemed more appropriate. :blank:
I have three variables. An int and two floats.
The int and one float increment in a cog.
The other float increments in the main program.
I expect to see all three increment on each run.
The int in the cog increments fine.
The float in the main program increments fine.
However, the float in the cog resets to zero periodically.
Why is this?
#include "simpletools.h" static volatile int globalInt; static volatile float globalFloat; void incrementNumbers(void *par); unsigned int stack[40 + 25]; int main() { float localFloat; // Initialize all variables to 0. globalInt = 0; globalFloat = 0.0; localFloat = 0.0; int incrementNumbersCog = cogstart(&incrementNumbers, NULL, stack, sizeof(stack)); while(1) { print("%d, %f, %f\n",globalInt,globalFloat,localFloat); localFloat = localFloat + 0.5; pause(100); } } void incrementNumbers(void *par) { while(1) { globalInt++; globalFloat = globalFloat + 1.0; pause(200); } }
Here is a screenshot of the problem:
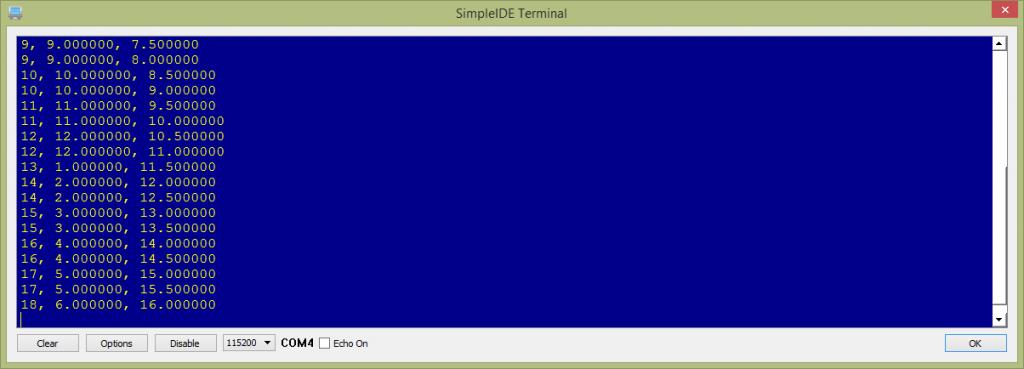
Notice how the globalFloat resets to 0 while the others keep incrementing. This happens repeatedly as the program runs.
P.S. Is this the right place to post this? It is C, not CPP, but I couldn't find another area that seemed more appropriate. :blank:
Comments
This happened with an older version of the compiler distributed with SimpleIDE.
What version do you have? Click Help -> About.
SimpleIDE 0.9.45 which I think is the latest available isn't it?
It is the one currently available here: http://learn.parallax.com/propeller-c-set-simpleide/windows
Thank you for the version information.
I recommend this:
1. Delete Documents\SimpleIDE entirely.
2. Download this https://propsideworkspace.googlecode.com/archive/2505f11eb63a3cb9bfd157fae4df7b86a6e0c96e.zip
3. Unzip the folder and rename it SimpleIDE
4. Move the new SimpleIDE to Documents
I have tested your program with the fpucog.c file included, and experience issues similar to what you have seen. The fpucog.c file was removed from the SimpleIDE workspace and libraries recently because of issues. Older SimpleIDE\Learn folders may still have the fpucog.c file in the library.
I have also tested your program with the recommended library and have not seen any problems.
Eric, .... I have not created an issue to track this yet. Sorry for neglecting it.
I was pulling my hair out last night when what I thought was a very simple idea turned into an unsolvable mess.
Now I can get back to writing more spaghetti code!
NOTE: In case someone else is reading this,It seems that I had to also replace the same set of files in C:\Program Files (x86)\SimpleIDE\Workspace in addition to Documents\SimpleIDE to affect the update.