What wrong with My HMC5883 on multiwii
I use this code
/*
An Arduino code example for interfacing with the HMC5883
by: Jordan McConnell
SparkFun Electronics
created on: 6/30/11
license: OSHW 1.0, http://freedomdefined.org/OSHW
Analog input 4 I2C SDA
Analog input 5 I2C SCL
*/
#include <Wire.h> //I2C Arduino Library
#define address 0x1E //0011110b, I2C 7bit address of HMC5883
void setup(){
//Initialize Serial and I2C communications
Serial.begin(9600);
Wire.begin();
//Put the HMC5883 IC into the correct operating mode
Wire.beginTransmission(address); //open communication with HMC5883
Wire.write(0x02); //select mode register
Wire.write(0x00); //continuous measurement mode
Wire.endTransmission();
}
void loop(){
int x,y,z; //triple axis data
//Tell the HMC5883 where to begin reading data
Wire.beginTransmission(address);
Wire.write(0x03); //select register 3, X MSB register
Wire.endTransmission();
//Read data from each axis, 2 registers per axis
Wire.requestFrom(address, 6);
if(6<=Wire.available()){
x = Wire.read()<<8; //X msb
x |= Wire.read(); //X lsb
z = Wire.read()<<8; //Z msb
z |= Wire.read(); //Z lsb
y = Wire.read()<<8; //Y msb
y |= Wire.read(); //Y lsb
}
//Print out values of each axis
Serial.print("x: ");
Serial.print(x);
Serial.print(" y: ");
Serial.print(y);
Serial.print(" z: ");
Serial.println(z);
delay(250);
}
But my result is""
/*
An Arduino code example for interfacing with the HMC5883
by: Jordan McConnell
SparkFun Electronics
created on: 6/30/11
license: OSHW 1.0, http://freedomdefined.org/OSHW
Analog input 4 I2C SDA
Analog input 5 I2C SCL
*/
#include <Wire.h> //I2C Arduino Library
#define address 0x1E //0011110b, I2C 7bit address of HMC5883
void setup(){
//Initialize Serial and I2C communications
Serial.begin(9600);
Wire.begin();
//Put the HMC5883 IC into the correct operating mode
Wire.beginTransmission(address); //open communication with HMC5883
Wire.write(0x02); //select mode register
Wire.write(0x00); //continuous measurement mode
Wire.endTransmission();
}
void loop(){
int x,y,z; //triple axis data
//Tell the HMC5883 where to begin reading data
Wire.beginTransmission(address);
Wire.write(0x03); //select register 3, X MSB register
Wire.endTransmission();
//Read data from each axis, 2 registers per axis
Wire.requestFrom(address, 6);
if(6<=Wire.available()){
x = Wire.read()<<8; //X msb
x |= Wire.read(); //X lsb
z = Wire.read()<<8; //Z msb
z |= Wire.read(); //Z lsb
y = Wire.read()<<8; //Y msb
y |= Wire.read(); //Y lsb
}
//Print out values of each axis
Serial.print("x: ");
Serial.print(x);
Serial.print(" y: ");
Serial.print(y);
Serial.print(" z: ");
Serial.println(z);
delay(250);
}
But my result is""
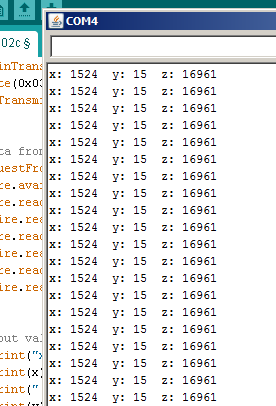
Comments
Were you expecting something different?
I don't see anything wrong with your HMC5883 based on what you wrote. The program is only displaying the raw values. You need to do some trig to calculate the heading.
As you can see, the forum software removes extra spaces from the posted code. To post code to the forum follow this tutorial.
When asking a question, it's a good idea to tell us what you were expecting to happen and not just what did happen.
It is my first time in this webboard
and now I use code below. I turn it around but results don't change . It give me 180 Deg all time
Then yes, you have a problem.
A diagram of how you've wired your sensor to your board would help as would a clear picture of your wiring.
A link to the exact board you are using would probably be helpful.
I2C lines need pull-up resistors, the Arduino library might be using the internal pull-ups but I'm not sure. Someone more familiar with the Arduino may be able to help if you provide the above information.
BTW, This is the Basic Stamp forum (not that I personally care much), I think the sensor forum would have probably been a better place to post your question (but don't do it now). If the moderators are inclined, they may move this thread.
While there are several people around here who use the Arduino, you might get better help on an Arduino forum. I have an Arduino but I haven't used it much.
I bet if you post the additional information (picture, link, etc.) someone around here will probably be able to help.
@darklizard
You might want to try some Arduino code from the learn site:
http://learn.parallax.com/KickStart/29133
Now I use that code,but results are
I use multiwii se v2.5 connect via USB Stlab ----> tx rx
>multiwii broad
thanks every one ^^
..............................Magnetic connect through aux_sda , aux scl on mpu6050. this is the code that I used
It works for me
thanks every one ++++