Scara robot using servos and hardware store components (Video)
My latest project is a Scara robot which is a robot arm that works in a horizontal plane. It's anthropomorphic because it has a shoulder and elbow joint, but usually the end effector just moves up and down rather than the arm as a whole. This arrangement simplifies control over a revolute coodinate arm, but inverse kinematics are still helpful. In the general discussion forum I posted a FORTH program to do inverse kinematics using fixed point trig. My previous IK robot arms used C++ with floating point, but I want to use FORTH this time to allow interactive control like my FORTH powered drawbot.
I had planned to wait until I completed this project before writing it up, but I decided documenting as I go will help keep me motiviated. If anyone else is interested in building one feel free to ask questions. Any suggestions (particularly about the end effector) would be welcome too. Here's the bill of materials:
Again this is a much simplier build than a revolute arm. Here's what I've done so far:
1. Cut two 7" pieces of U channel and drill 4-40 size holes every 1/2" to allow for experimentation with mounting.
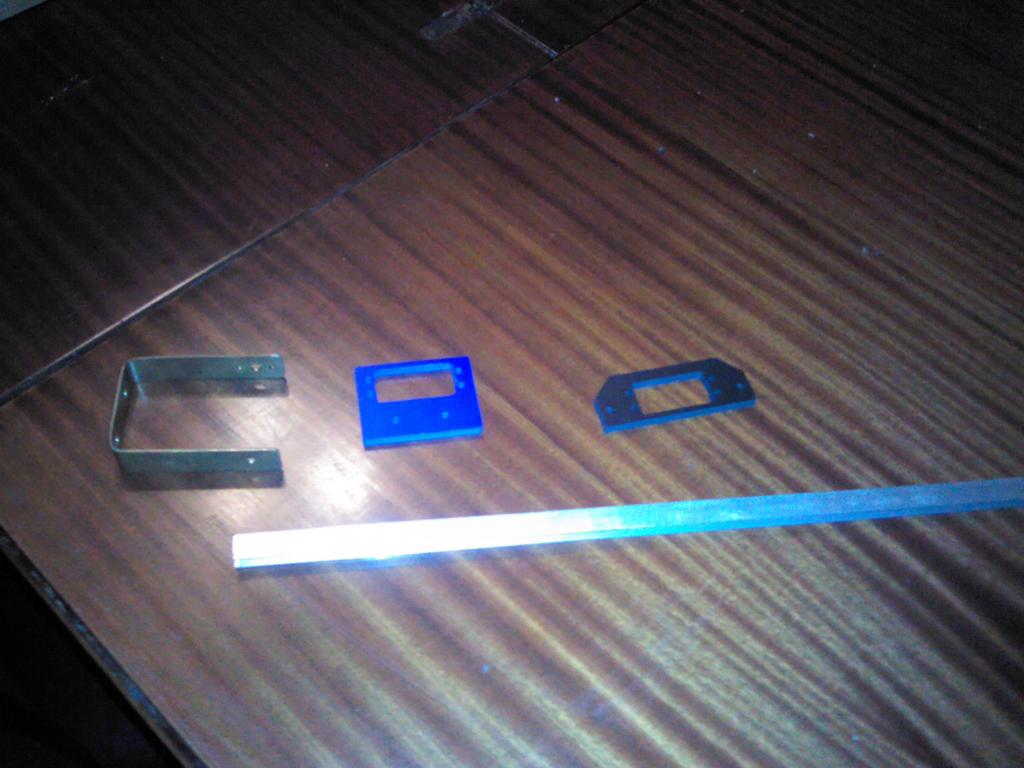
2. On the first piece of U channel mount two servos using servo brackets. Here's a picture:
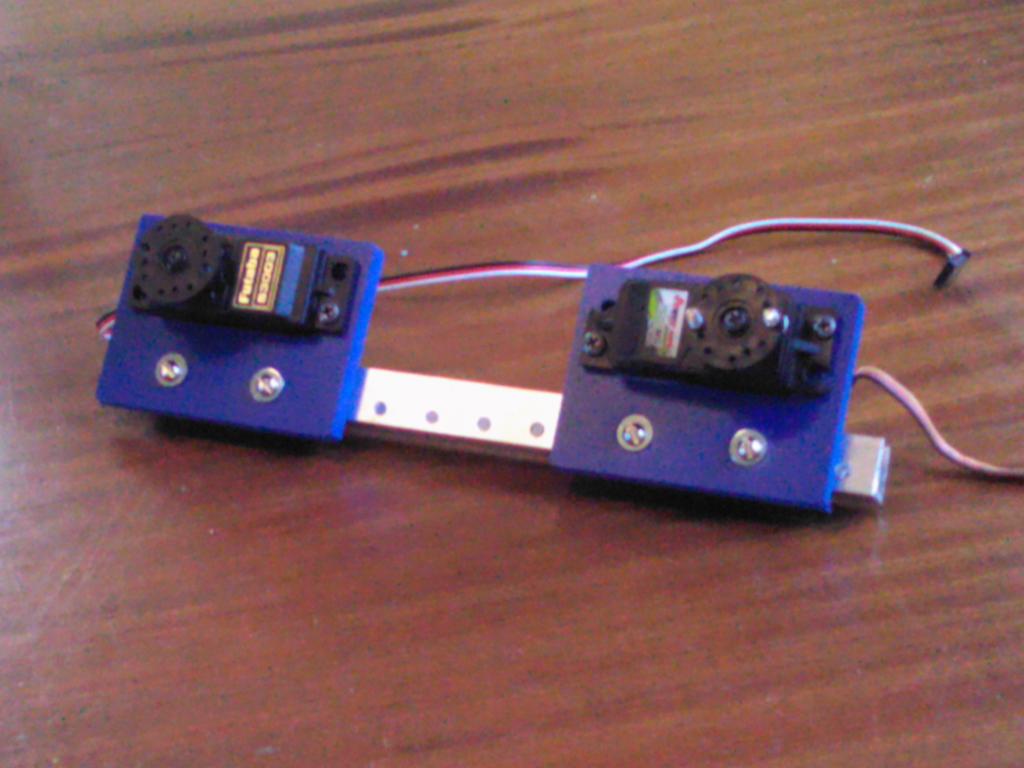
3. On the second piece of U channel enlarge a hole to allow access to a servo spline screw.
4. Make or buy a bracket to create a yoke for the shoulder joint. I make my own and glue a screw to the bottom of the servo to create the idler pivot. You can see the bracket in the first picture and the mounting below.
5. Screw the bracket to the wooden base, screw the servo horn to the bracket, and mount the servo bottom pivot. Use your strongest servo for this joint as it will be bearing the largest load.
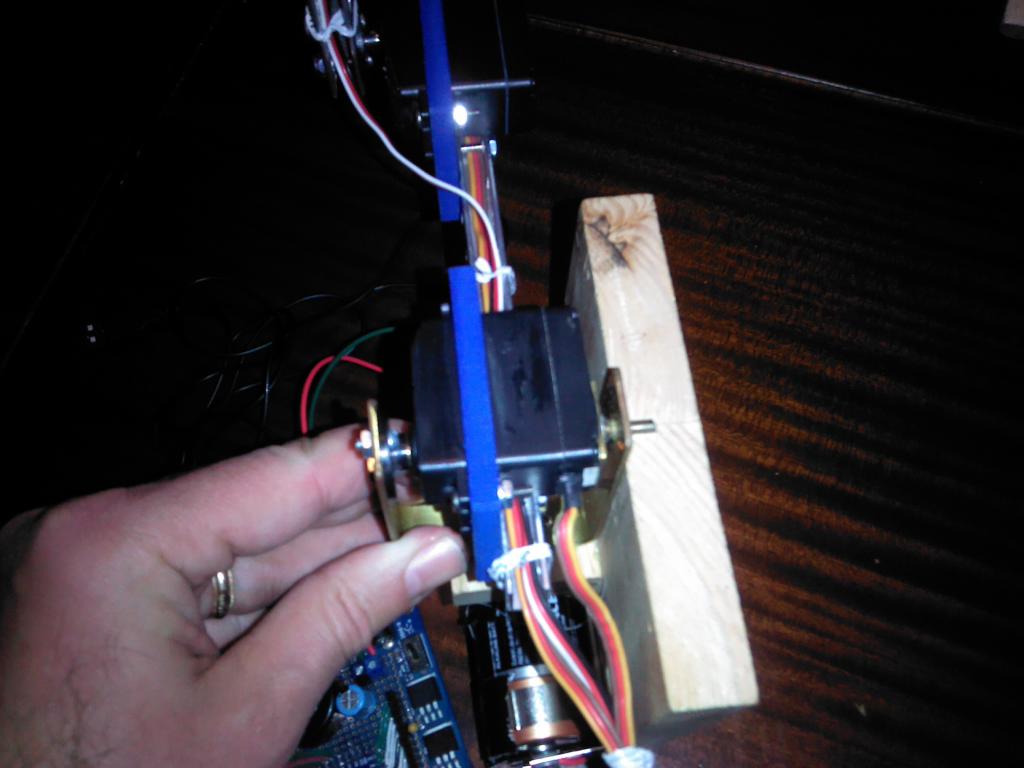
6. Screw the second piece of U channel to the second servo horn.
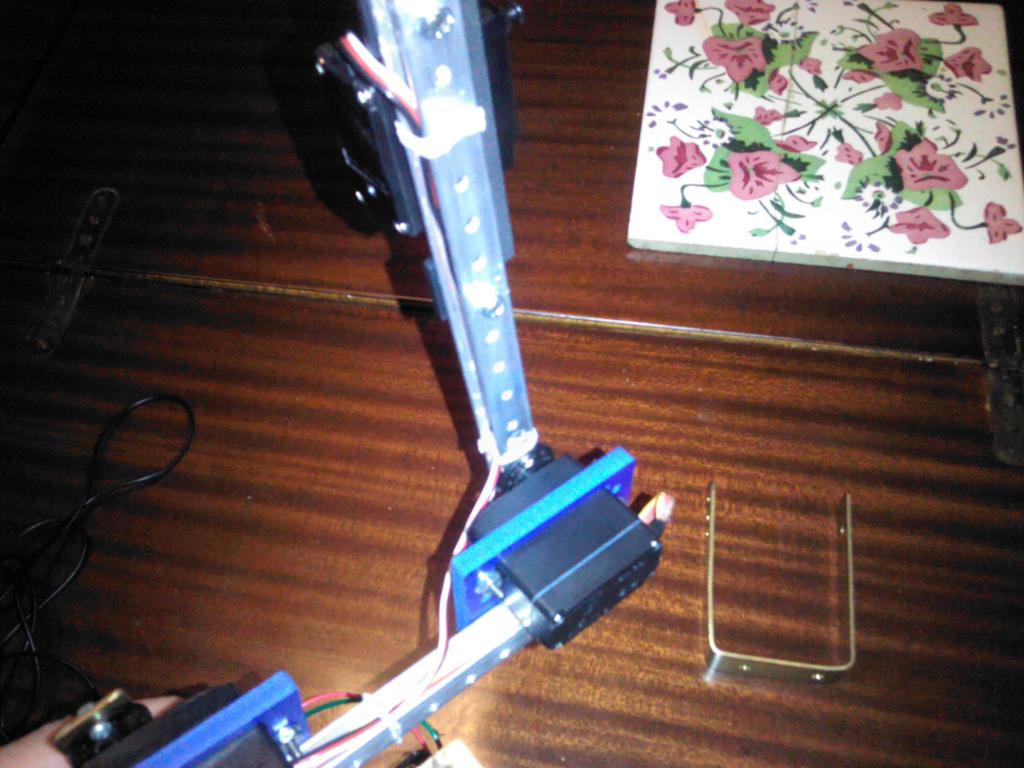
7. Mount the third servo at the end of the arm perpendicular to the other two. This servo will be used for the end effector lift mechanism. It will be best to use a lighter servo here to keep the mass lower.
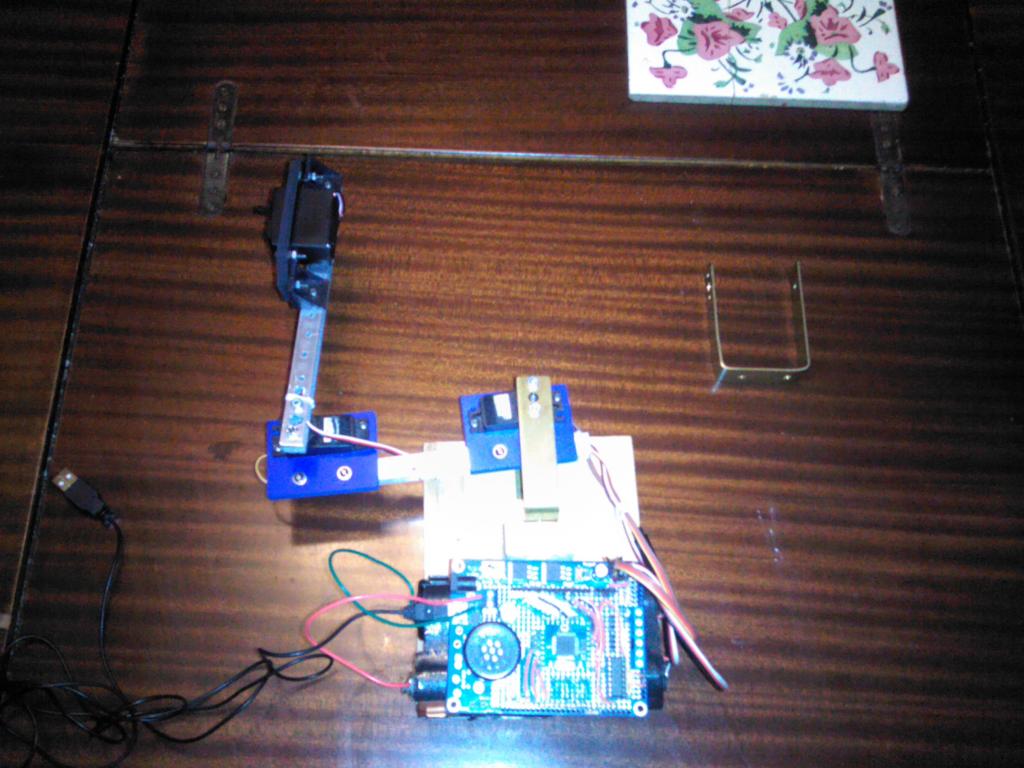
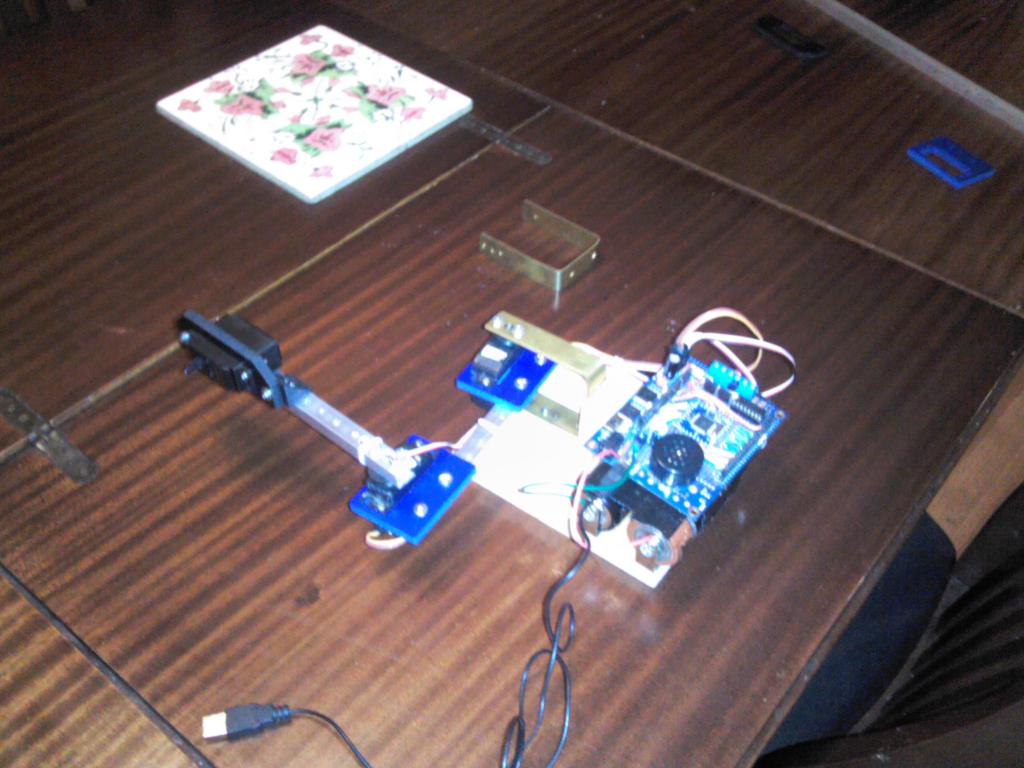
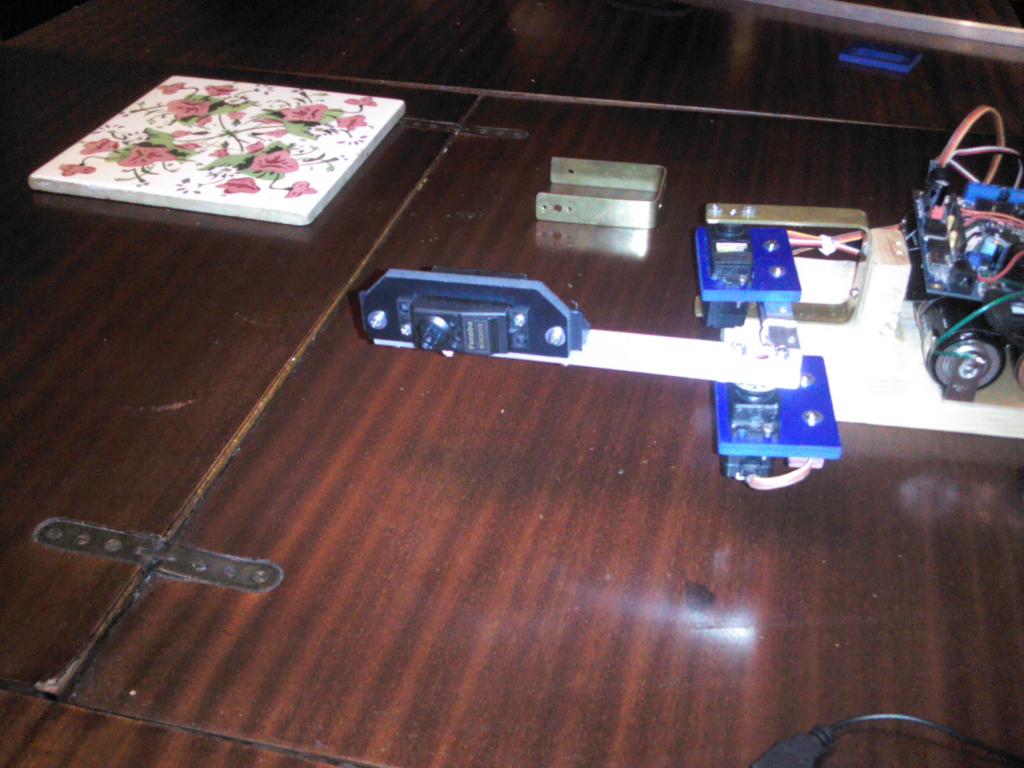
8. Mount the battery hold on the base and the control board above it. You'll need at least 3 servo headers, but the project board has four, so it will do nicely. Using D sized batteries allows the arm to fully extend without falling over as seen below.
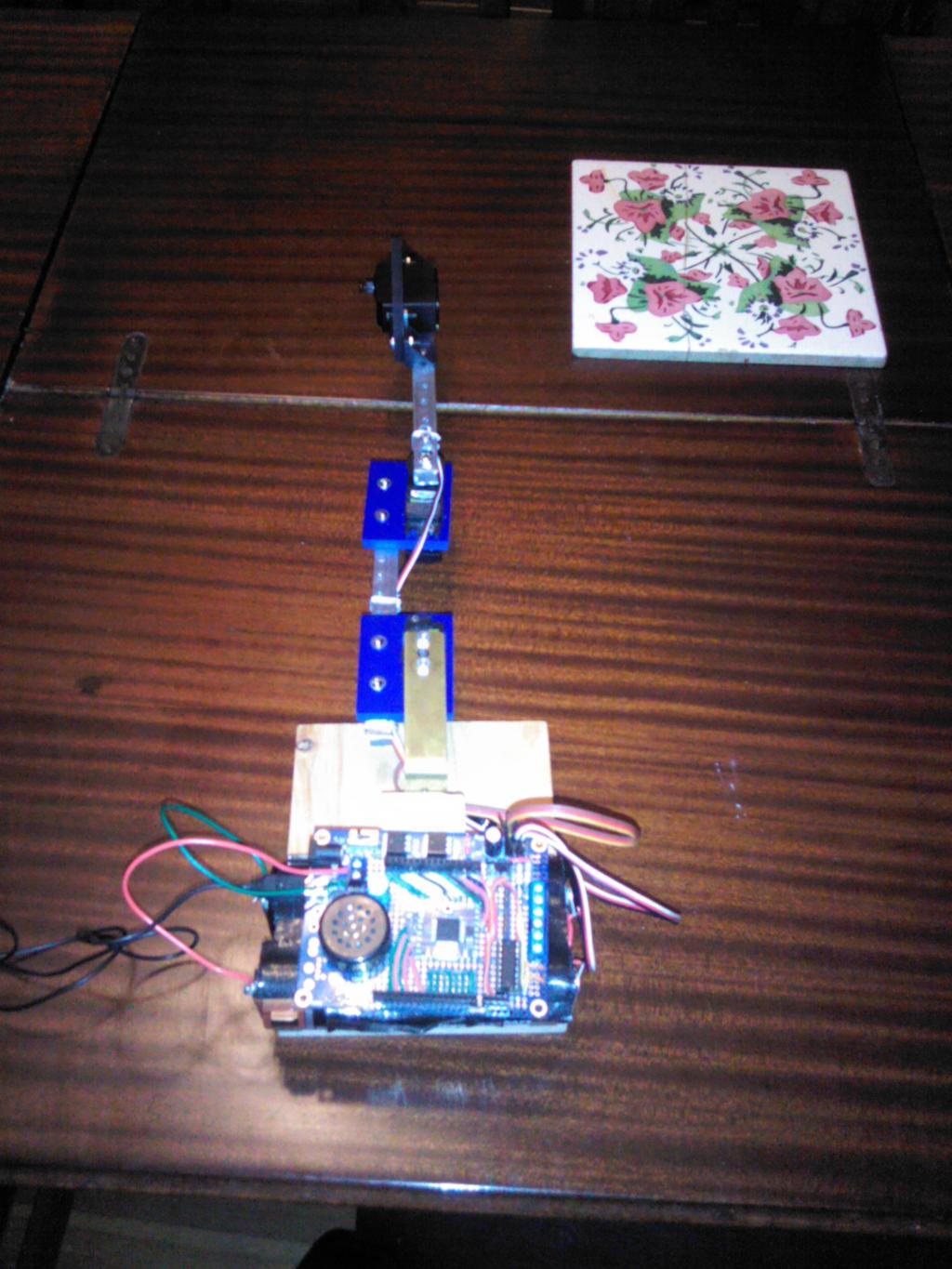
This forms the basic structure of the arm and I now plan to finish up the IK code. I already have some C++ and Spin IK code from prior projects, so if anyone is interested in building one of these but doesn't want to deal with FORTH I can post that too.
I had planned to wait until I completed this project before writing it up, but I decided documenting as I go will help keep me motiviated. If anyone else is interested in building one feel free to ask questions. Any suggestions (particularly about the end effector) would be welcome too. Here's the bill of materials:
3 standard sized servos and horns. I'm using Power HD metal gear servos. ($10-$19) 1/4 inch ID aluminum U channel ($6) three sets of servo brackets. I'm using sintra brackets from budget robotics ($2) 4-40 5/8 " bolts, nuts, and washers. ($3) A block of scrap wood to mount it on. Some metal to make a shoulder bracket ($2) Battery holder for 4 D batteries. This was free as it was salvage. I'm using D batteries to add weight to the base, and power it. A Propeller project board ($20).
Again this is a much simplier build than a revolute arm. Here's what I've done so far:
1. Cut two 7" pieces of U channel and drill 4-40 size holes every 1/2" to allow for experimentation with mounting.
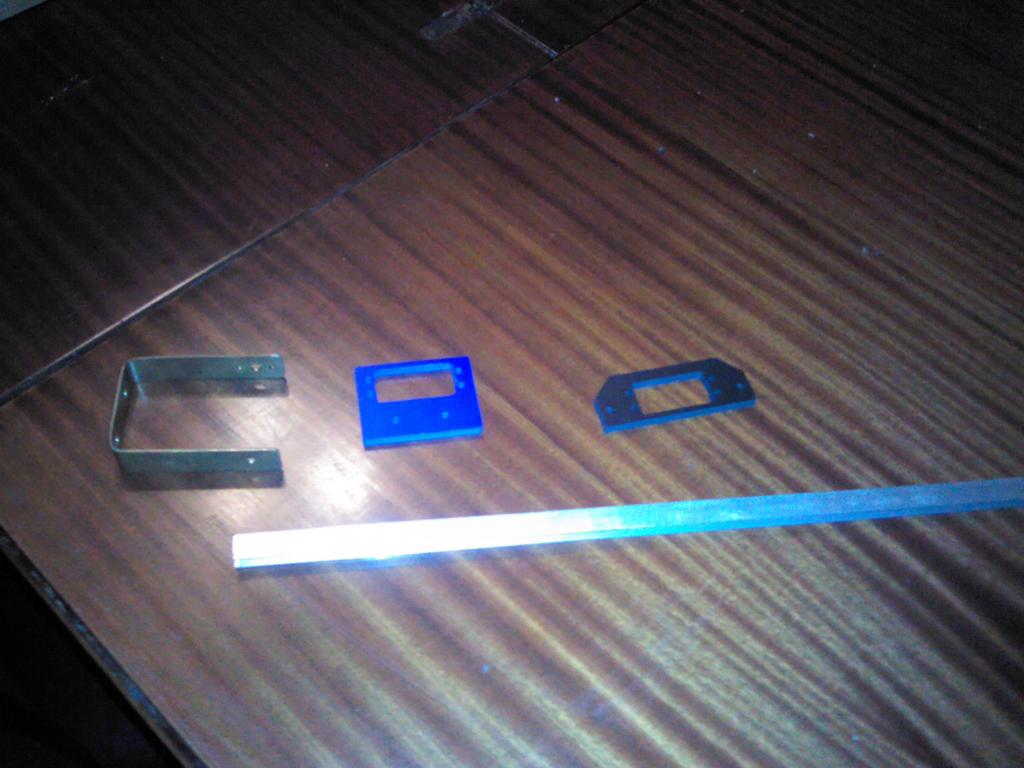
2. On the first piece of U channel mount two servos using servo brackets. Here's a picture:
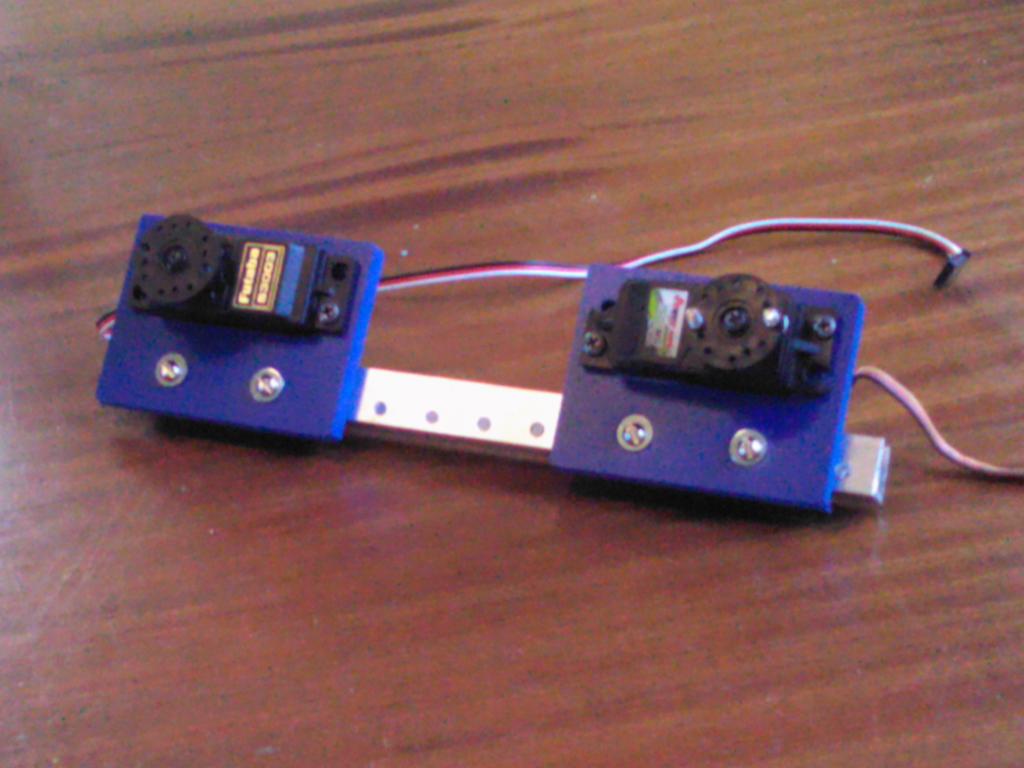
3. On the second piece of U channel enlarge a hole to allow access to a servo spline screw.
4. Make or buy a bracket to create a yoke for the shoulder joint. I make my own and glue a screw to the bottom of the servo to create the idler pivot. You can see the bracket in the first picture and the mounting below.
5. Screw the bracket to the wooden base, screw the servo horn to the bracket, and mount the servo bottom pivot. Use your strongest servo for this joint as it will be bearing the largest load.
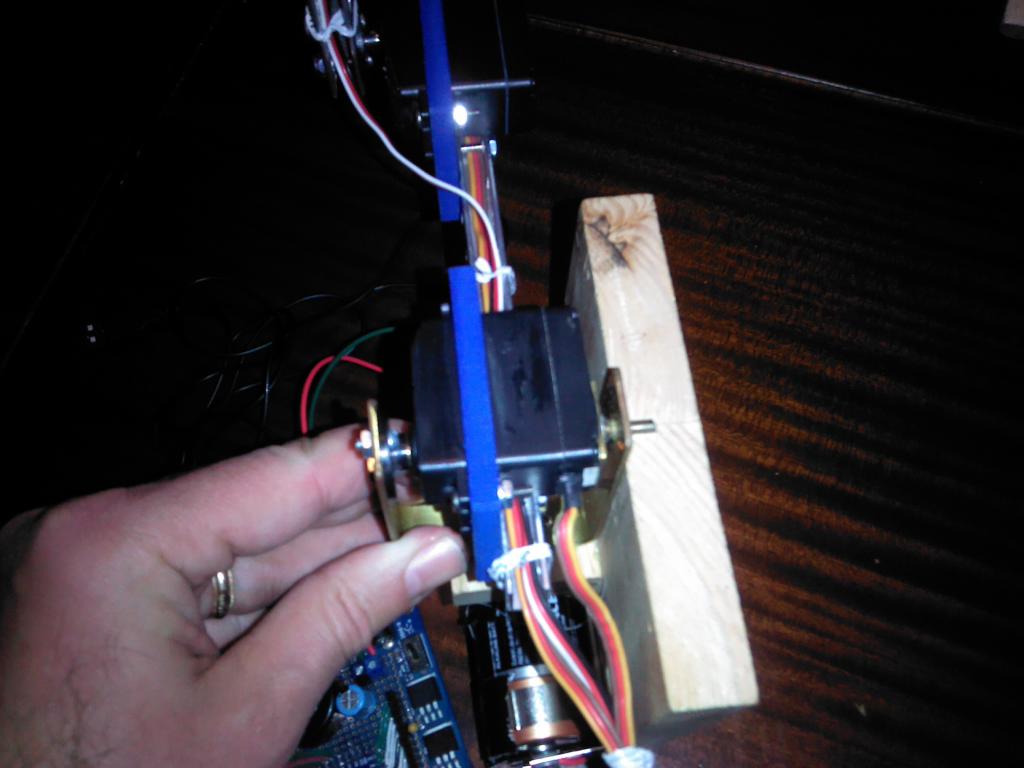
6. Screw the second piece of U channel to the second servo horn.
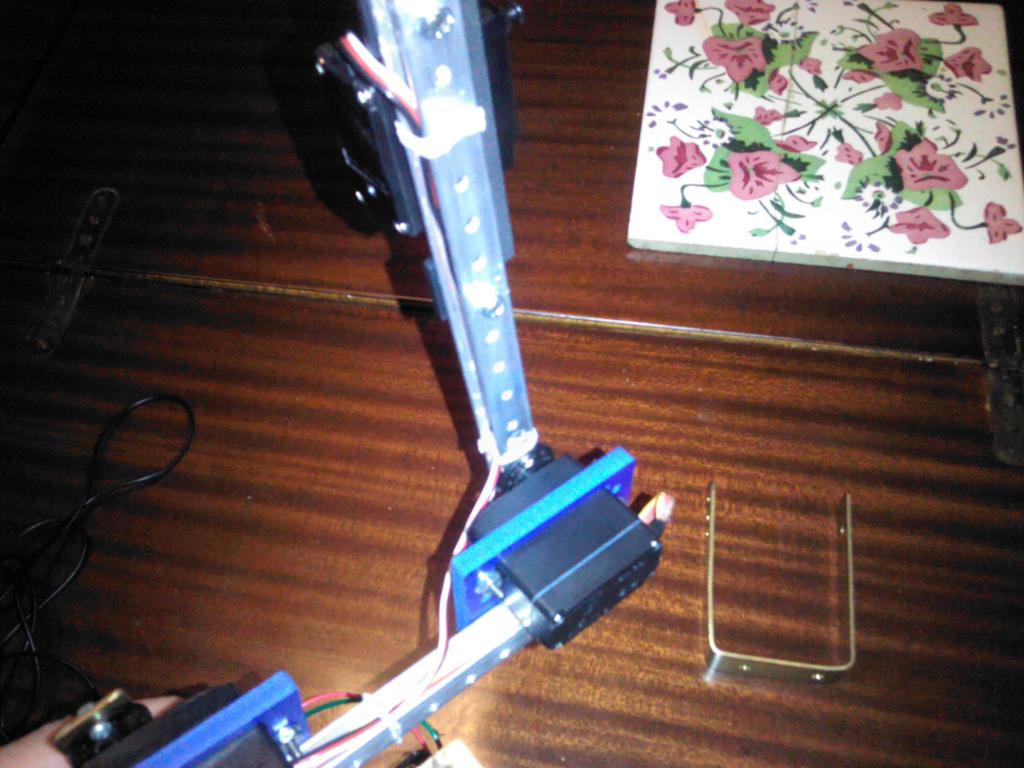
7. Mount the third servo at the end of the arm perpendicular to the other two. This servo will be used for the end effector lift mechanism. It will be best to use a lighter servo here to keep the mass lower.
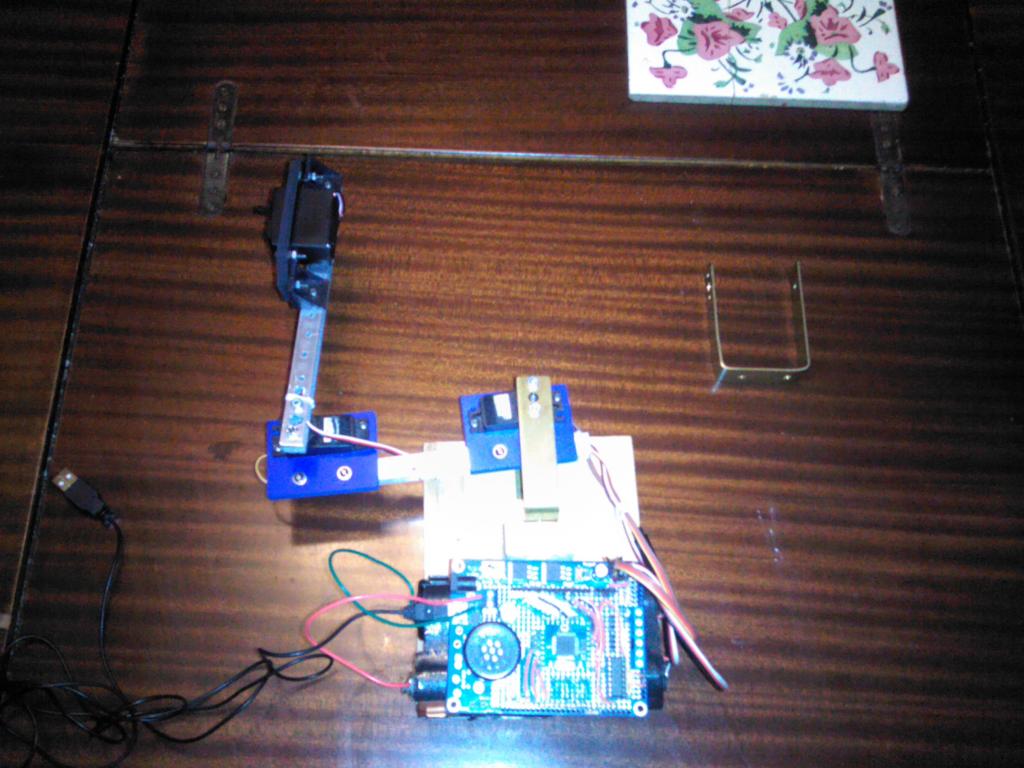
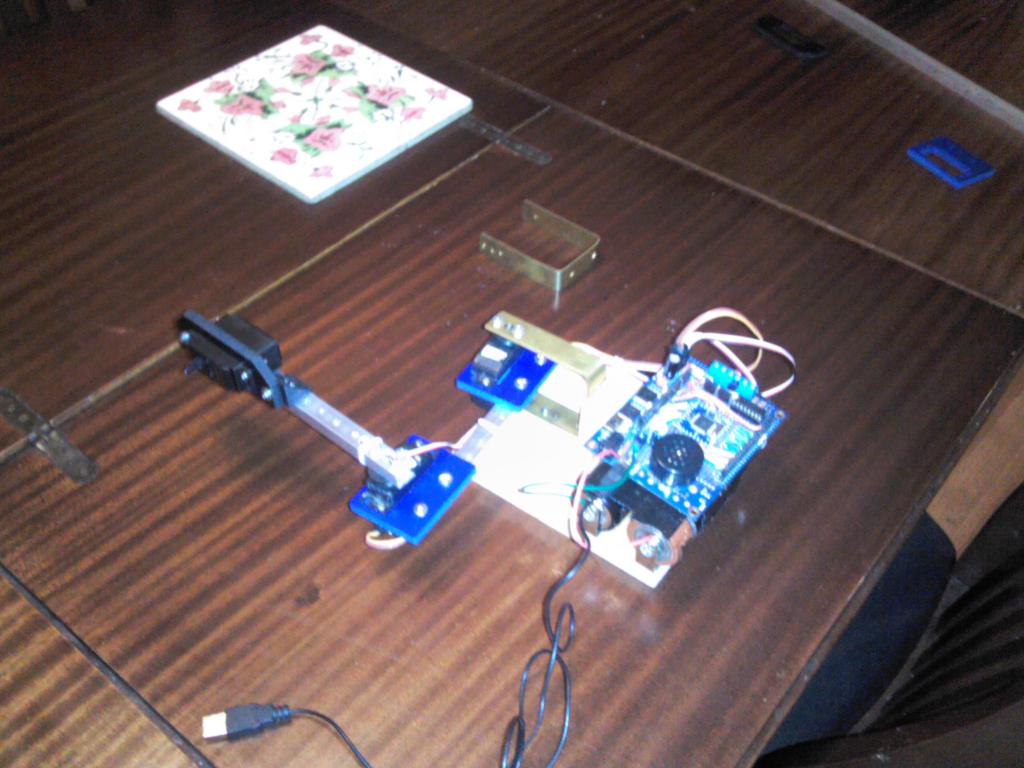
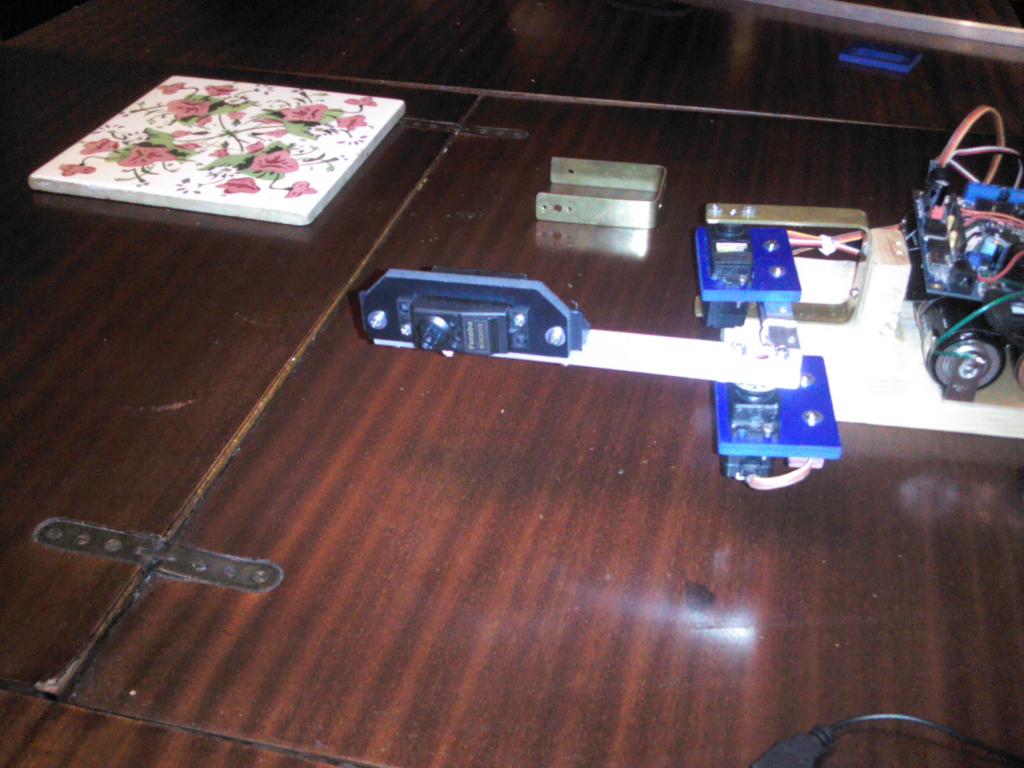
8. Mount the battery hold on the base and the control board above it. You'll need at least 3 servo headers, but the project board has four, so it will do nicely. Using D sized batteries allows the arm to fully extend without falling over as seen below.
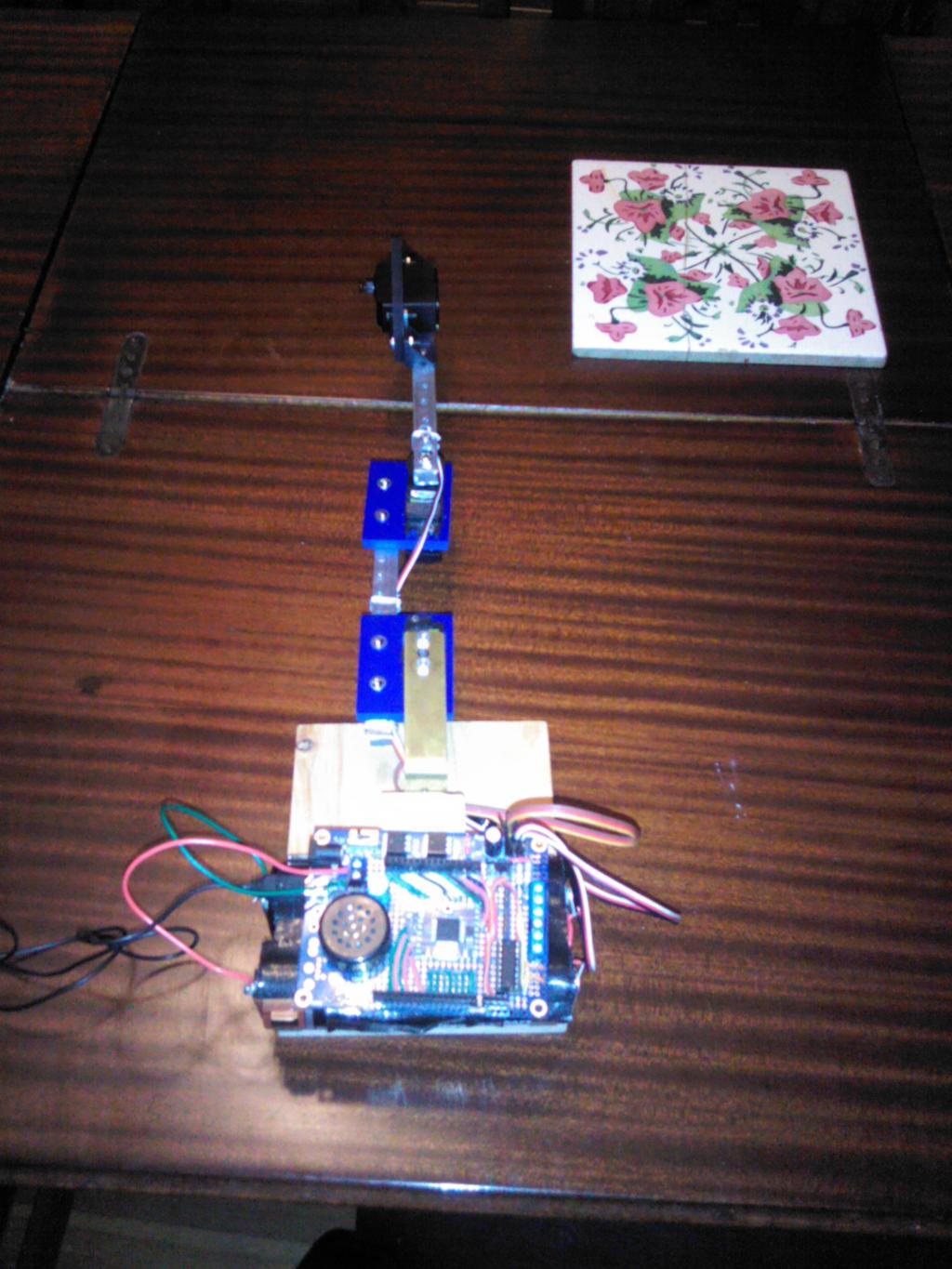
This forms the basic structure of the arm and I now plan to finish up the IK code. I already have some C++ and Spin IK code from prior projects, so if anyone is interested in building one of these but doesn't want to deal with FORTH I can post that too.
Comments
http://www.ebay.com/itm/Random-One-Tower-of-Hanoi-Wood-Puzzle-Toy-Great-Brain-Teaser-Game-Puzzles-NEW-/331011538090?pt=Pretend_Play_Preschool_US&hash=item4d11d33caa
For anyone who's curious, below is the Propforth IK code debugged up to the set_position method.
SinB/TanB=??? (see my 'Nerd Humor' post in General)
Here are some pictures of the completed end effector. The design turned out to be pretty simple after a number of more complicated failures. The rubber band seems to provide just enough give to prevent binding but still be effective.
Next is connecting the vacuum system to the suction cup and integration testing.