Difficulties with C++ Hello World
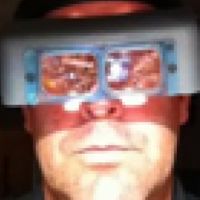
For reasons I can't seem recall right now I decided to try compiling a C++ program. I have the p2test build and I am compiling with SimpleIDE. I get a scope error that I don't understand.
The error is:
Sorry for such noob problem but I can't figure it out.
Paul
/** * @file hello-cpp.cpp * This is the main hello-cpp program start point. */ #include <iostream> #include "propeller.h" /** * Main program function. */ int main(void) { cout << "Hello, World!"; return 0; }
The error is:
Project Directory: /Users/doc/code/hello-cpp/ propeller-elf-c++ -o a.out -Os -mlmm -I . -fno-exceptions -fno-rtti hello-cpp.cpp hello-cpp.cpp: In function 'int main()': hello-cpp.cpp:14:5: error: 'cout' was not declared in this scope hello-cpp.cpp:14:5: note: suggested alternative: /opt/parallax/lib/gcc/propeller-elf/4.6.1/../../../../propeller-elf/include/c++/4.6.1/iostream:62:18: note: 'std::cout' Done. Build Failed! Click error or warning messages above to debug.
Sorry for such noob problem but I can't figure it out.
Paul
Comments
C++ has several great advantages over C but i'm too tired to talk about them LOL.
For reference, I've found that programs with a format specifier in the printf rarely take less than 15KB in CMM -Os mode.
Of course then it becomes a C program although I used the c++ compile option.
It was my intention to go through some tutorials on the web to familiarize myself with c++ but now I'm having second thoughts.
Paul
I was talking with one of my old professors last night. We both agreed that learning C syntax before learning C++ is a good path.
Learning C enables easily understanding C#, Java, PHP, and others. The only problem with learning C before C++ (or other object oriented languages) is in having to make the leap of understanding object methodologies in C++. Spin has some object methodologies, but not some of the more useful ideas like extending classes, class abstraction, and function overloads.
Use of the Standard Template Library doesn't define what is or isn't a C++ program.
C++ is mostly backward compatible with C, and in the case of things like printf(), fully compatible. I've written a LOT of C++ code, and rarely use the STL, because it's a bloated pig and it's very hard to debug. :-)
I use lots of class objects, inheritance, overloading, and all the other things C++ is good at that aren't available in C.
If you're already familiar with C, and structures, C++ classes will be relatively easy to grasp.