Driver code for 4D SGE (serial) mode displays
I recently purchased several of the 4.3" cap touch displays for a project. I thought I'd try my hand at writing a driver for the commands. It's far from finished but would like some critique from the crowd here before I get too far. In the zip file I also included a demo program I'm working with as I go along.
Here's my setup using a Quickstart board:
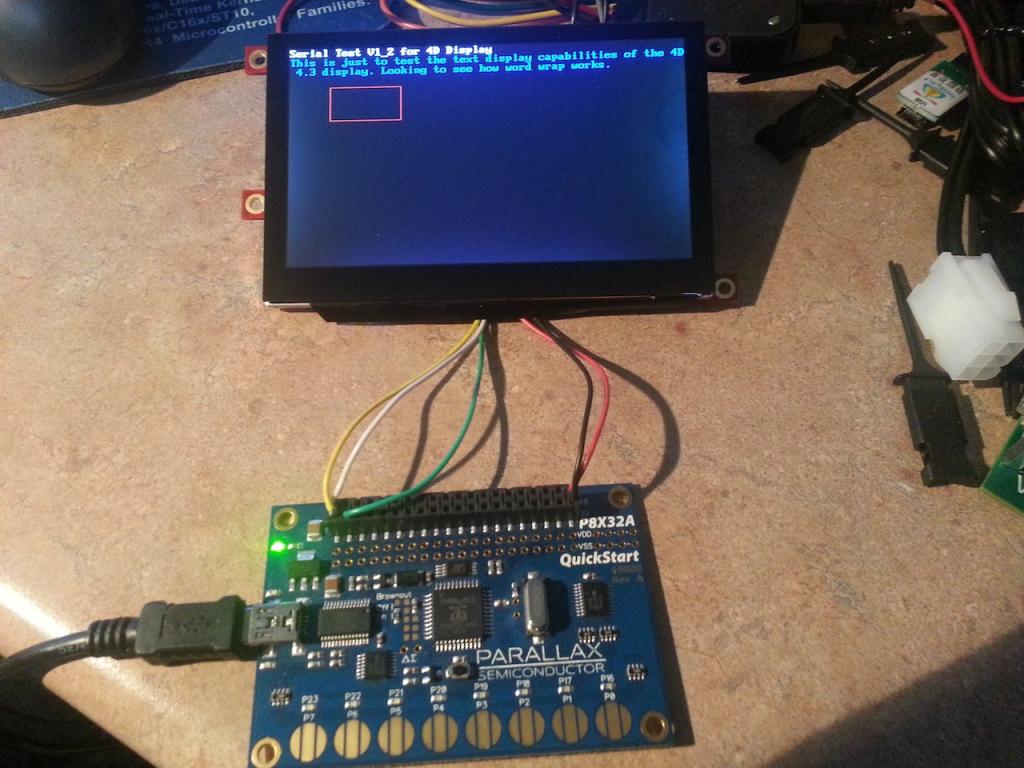
Edit: This will work with the new 3.2" displays that Parallax is now selling too.
Edit: Attached latest driver.
'' ================================================================================================= '' '' File....... 4D_SPE_Driver_V0_1.spin '' Purpose.... Serial commands for 4D Systems SPE '' Author..... Don Merchant '' Licensing.. MIT License - see end of file for terms of use. '' '' '' E-mail..... donmerch@yahoo.com '' Started.... FEB 15 2013 '' Updated.... '' '' ================================================================================================= con var obj ser : "FullDuplexSerial" pub Start(RX, TX, RST, Baud) {{ Start FullDuplexSerial driver }} ser.Start(RX, TX, 0, Baud) outa[RST]~~ dira[RST]~~ ' Set RST pin as output pub Move_Cursor(Line, Col) : result {{ The Move Cursor command moves the text cursor to a screen position set by line and column parameters in HEX. The line and column position is calculated, based on the size and scaling factor for the currently selected font. When text is outputted to screen it will be displayed from this position. The text position could also be set with Move Origin command if required to set the text position to an exact pixel location. Note that lines and columns start from 0, so line 0, column 0 is the top left corner of the display. Response: 0x06 ACK (byte) if successful Anything else implies mismatch between command and response. }} ser.tx($FF) ser.tx($E9) ser.tx(Line >> 8) ser.tx(Line & $FF) ser.tx(Col >> 8) ser.tx(Col & $FF) result := ser.rxtime(20) pub Put_Char(Char) : result {{ The Put Character command prints a single character to the display. Response: 0x06 ACK (byte) if successful Anything else implies mismatch between command and response. }} ser.tx($FF) ser.tx($E9) ser.tx(Char >> 8) ser.tx(Char & $FF) result := ser.rxtime(20) pub Put_String(Strng) : result | d, r1, r2 {{ The Put String command prints a string to the display. The argument can be a string constant or a pointer to a string. A string needs to be terminated with a NULL (00). Response: 0x06 ACK (byte), StringLength (word) if successful Anything else implies mismatch between command and response. }} ser.tx($00) ser.tx($18) ser.str(Strng) ser.tx($00) d := ser.rx 'time(200) if d == $06 r1 := d << 16 d := ser.rxtime(2) r2 := d << 8 r1 := r1 | r2 d := ser.rxtime(2) r1 := r1 | d result := r1 pub Text_FG_Color(Color) : result | d, r1, r2 {{ The Text Foreground Color command sets the text foreground color, and reports back the previous foreground color. Response: 0x06 ACK (byte) and Previous Text Foreground Color (word) if successful Anything else implies mismatch between command and response. }} ser.tx($FF) ser.tx($E7) ser.tx(Color >> 8) ser.tx(Color & $FF) d := ser.rx 'time(200) if d == $06 r1 := d << 16 d := ser.rxtime(2) r2 := d << 8 r1 := r1 | r2 d := ser.rxtime(2) r1 := r1 | d result := r1 pub Draw_Rect(x1, y1, x2, y2, color) : result {{ The Draw Rectangle command draws a rectangle from x1, y1 to x2, y2 using the specified color. The line may be tessellated with the Line Pattern command. Response: 0x06: ACK (byte) if successful Anything else implies mismatch between command and response. }} ser.tx($FF) ser.tx($C5) ser.tx(x1 >> 8) ser.tx(x1 & $FF) ser.tx(y1 >> 8) ser.tx(y1 & $FF) ser.tx(x2 >> 8) ser.tx(x2 & $FF) ser.tx(y2 >> 8) ser.tx(y2 & $FF) ser.tx(color >> 8) ser.tx(color & $FF) result := ser.rxtime(20) pub Clear_Scrn : result {{ The Clear Screen command clears the screen using the current background colour. This command brings some of the settings back to default; such as * Transparency turned OFF * Outline colour set to BLACK * Opacity set to OPAQUE * Pen set to OUTLINE * Line patterns set to OFF * Right text margin set to full width * Text magnifications set to 1 * All origins set to 0:0 The alternative to maintain settings and clear screen is to draw a filled rectangle with the required background colour. Response: 0x06: ACK (byte) if successful Anything else implies mismatch between command and response. }} ser.tx($FF) ser.tx($CD) result := ser.rxtime(40) dat {{ Colors }} RED word $F800 GREEN word $0400 LIME word $07E0 WHITE word $FFFF BLACK word $0000 BLUE word $001F {{ ┌──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┐ │ TERMS OF USE: MIT License │ ├──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┤ │Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation │ │files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, │ │modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software│ │is furnished to do so, subject to the following conditions: │ │ │ │The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.│ │ │ │THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE │ │WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR │ │COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, │ │ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. │ └──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┘ }}
Here's my setup using a Quickstart board:
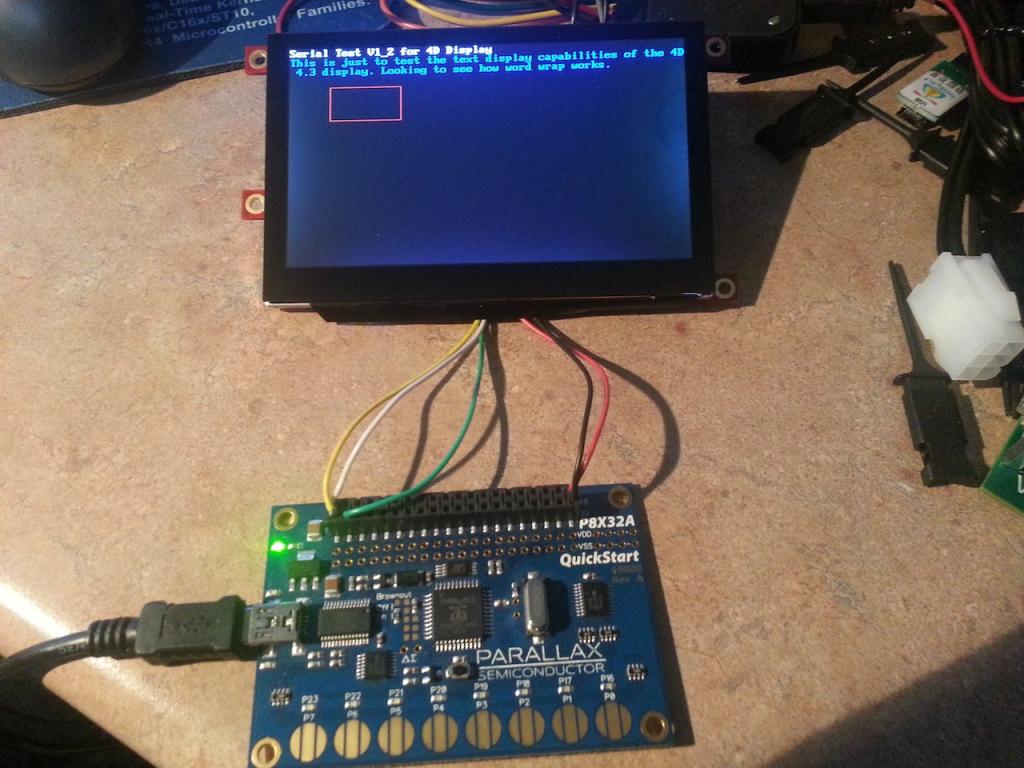
Edit: This will work with the new 3.2" displays that Parallax is now selling too.
Edit: Attached latest driver.
Comments
I'm using the uLCD-43PCT with PMMC Ver 3.3 and SGE Ver 1.0 @ 115_200 Baud.
I've added some more functions to the driver and will continue to as time goes on. So far this display has been pretty good to work with.