How do I write text strings to SD card?
I have this part of my program that stores hex data from 2 serial channels into a buffer and then to an sd card. The buffers are a 514 word buffer :
I then use pread to read out the data from the sd card (512 bytes) and store it into a word buffer then run the data from the word buffer through this code to get my print out on PST:
It produces an output like this:
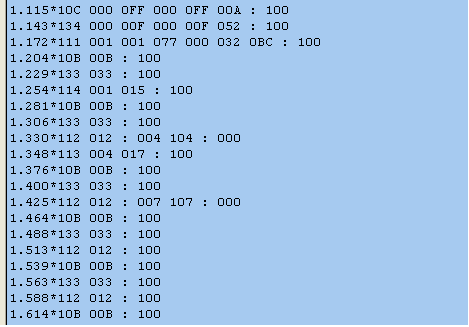
which is what I want. My question is how do I convert this data and store it on the SD card as a text file so that it can be read out in the same format as what is shown on PST?
repeat repeat d := \ser1.rx_check ' d = serial channel 1 if d <> -1 ' Look for data if c == 0 ' If RTC hasn't been reset... rtc.reset c := 1 ' Set so RTC won't be reset again if mrk == 0 ' If time stamp wasn't logged... if d <> $0000 ' Don't write time stamp to Master ACK's buf1[i] := (rtc.seconds | $B000) ' Add seconds with $B000 mask i++ buf1[i] := (rtc.millis | $A000) ' Add milliseconds with $A000 mask i++ mrk := 1 ' Time stamp is only logged once at beginning of Master command buf1[i] := (d | $8000) ' Write data from d to buffer and add $8000 mask i++ repeat d := \ser1.rx_check if(d == -1) ' If no data then flush ser1.rx_flush quit s := \ser2.rx_check ' s = serial channel 2 if s <> -1 ' If data present... buf1[i] := (s | $4000) ' Write data to buffer with $4000 mask i++ mrk := 0 ' Reset time stamp flag if i => 254 ' Watch index i i := 0 ' Reset i if true quit ' Quit loop to write buffer to sd card sd.pwrite(@buf1[0], 512) ' Write 512 bytes to sd card from buffer
I then use pread to read out the data from the sd card (512 bytes) and store it into a word buffer then run the data from the word buffer through this code to get my print out on PST:
repeat i from 0 to 255 ' Repeat for 256 word buffer d := (block[i] & $F000) ' Mask for data logger flags case d $4000: ' Serial 2 data s1 := 1 ' Set Serial 2 flag if m == 1 ' If data was preceeded by Serial 1 data... term.tx(58) ' Print colon.. term.tx(32) ' and space m := 0 ' Reset Serial 1 flag so colon doesn't print again term.hex(block[i], 3) ' Print Serial 2 data if block[i] == $4100 ' If Serial 2 data was an ACK.. if s2 == 0 ' and no other Serial data was received.. term.tx(13) ' then CR for a new line else s2 := 1 ' If not an ACK m := 0 term.tx(32) ' then print space $8000: ' Serial 1 data if block[i] == $8000 ' Look for Serial 1 ACK if m == 0 if s1 == 1 ' Look to see if this was a response to Serial 2 term.tx(58) ' If so print a colon.. term.tx(32) ' then a space... term.hex(block[i], 3) ' then the Serial 1 ACK.. term.tx(13) ' then a CR for new line s1 := 0 ' Reset Serial 1 flag else term.hex(block[i], 3) ' If $8000 was not an ACK then just print it.. term.tx(32) ' and a space else if (block[i] & $0F00) == $0100 ' Look for no response from Serial 2... if m == 1 term.str(string("** NO RESP **", 13)) term.hex(block[i], 3) ' Print Serial 1 data term.tx(32) ' and a space s2 := 0 ' Reset s2 flag m := 1 ' Set flag to look for Slave response $A000: term.decn((block[i] & $0FFF), 3) ' Display milliseconds term.tx(42) ' Print asterisk $B000: if (rtc.seconds) > 0 ' Don't print seconds unless > 0 term.dec(block[i] & $0FFF) ' Display seconds term.tx(46) ' Print period
It produces an output like this:
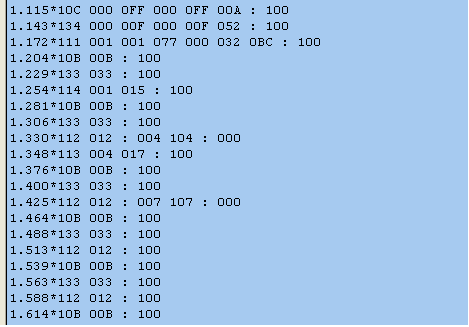
which is what I want. My question is how do I convert this data and store it on the SD card as a text file so that it can be read out in the same format as what is shown on PST?
Comments
If you look at the internals of those methods, you see that at some point they call the "tx" method. That's where it has converted the next digit, and is ready to output it. When you copy the method into your program, you'll have to modify it so that it builds a string in memory instead of tx'ing it. Or, if you don't mind closely coupling it with the FSRW code, you can simply replace tx with a pputc option.
Here is how I would structure the function:
I don't know if this is in your scope, but there are example of doing this in the tutorials section of the propforth download archive.
You don't have to learn forth to get the jist of whats going on. It might provide some perspective on ways to 'git 'er done'.