Hello, World :)
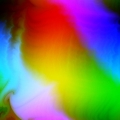
In microcontroller world, "Hello" means blinking some leds. So I started to blink:
maybe in some strange way, but it blinks
And I discovered that I cannot pass more than 16-bits delay value via coginit. Where is this value truncated?
[FONT=courier new]con delay=40000 pub start coginit (1,@proc,delay) dat[/FONT] [FONT=courier new]proc mov 489,#3 shl 489,#16 mov dira,489 loop3 mov 490,par shl 490,#4 mov 489,#1 shl 489,#16 mov outa,489 loop djnz 490,#loop mov 489,#1 shl 489,#17 mov outa,489 mov 490,par shl 490,#4 loop2 djnz 490,#loop2 jmp #loop3[/FONT]
maybe in some strange way, but it blinks

Comments
Good start. No idea what is going on there but I would suggest that you declare some longs in your cog rather than use numerical addresses.
Put them at the end of your code.
then you can use them in your code like
or (Note the lack of a # in that, everyone always makes mistakes with # or not.)
For completeness an ORG at the begining of PASM is a good idea and a FIT at the end.
Oh yeah, normally one would use cognew instead of coginit. It's not often you actually need to start a specific cog and if you are mixing and matching code from here and there it avoids any conflicts over which object uses which cog.
When you pass a literal, you can only pass 9 bits, refer to asm manual. If you need to use a larger value, you need to set it up as a var at compile time.
Delay. Long. 32bitvalue
Jim
and it works, giving a led on pin p16 blinking with sawform way. But why is frequency about 0.3 Hz, when I supposed 1.8 Hz?
Counting:frqb=$80000000 => 40MHz
frqb=1 =>40000000/$80000000 => 0.0186Hz
frqb=100 =>1.86 Hz
Where am I wrong with this counting and this code?
Now it works OK. Time to change pin from 16 to 11 and try some beeps
Edit - changed pin to 11 and freq to 53*440. Now it beeps
First attempt to access vga, successful. Will try to write a nostalgic vga driver, with borders.
Now it displays only background color, 640x400 2-colors window on 960x600 screen (my monitor is 1920x1200, so I made 1/2 of these)
Good progress here. Don't slow down!
This code gives too much border; I updated this to get 640x400 screen on 720x480 border. It looks much better now, and clock is 30.625 MHz (pll/4, $1880_0000 as frqa_val). And it gives more time between waitvids.
Now I am struggling with basics of Spin, to add a frame buffer and character definitions. (is there any Spin tutorial somewhere?)
Expected: white screen (buffer filled with $FF)
Got: vertical color bars.
Edit:found error: add buffer_addr,4; should be #4
Still get color vertical bars
Edit: test from fsrw 2.6 works (see code section). FemtoBasic cannot mount this card. It is 4GB, FAT32, SDHC card.
Edit 2: just read about FemtoBasic doesn't support SDHC. Need to use smaller card. Or try to add this http://obex.parallax.com/objects/619/ driver...