Autobaud on the propeller not using special characters but total packet length.
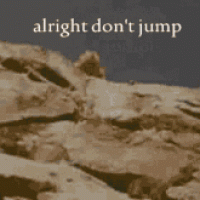
I am trying to code an autobaud routine using total packet length, but have run into basic problems with using CNT and the fact that it rolls over.
And perhaps there is something that won't work with using total packet length.
What my code is trying to do is use waitpeq to find the first start bit.
My serial transmission is no faster than 1 transmission per second, so I am using this information to determine the total packet start and stop times.
I measure the high to low times, and if the initial one is more than 1 second, i know its my start bit time.
I then mesure the high to low times again, and if its more than 1 second, I know that the last rise to high time was my stop bit. I then do some math to calculate the bit rate knowing I have 9 total bits which include the start and stop bit.
I know i may have some obvious errors in my code, but my fundamental error is using CNT to store the rise and fall times. I know there is a better way to still maintain very accurate timings, and properly deal with the CNT roll.
(which i am not doing and thus why my code is Smile)
And perhaps there is something that won't work with using total packet length.
What my code is trying to do is use waitpeq to find the first start bit.
My serial transmission is no faster than 1 transmission per second, so I am using this information to determine the total packet start and stop times.
I measure the high to low times, and if the initial one is more than 1 second, i know its my start bit time.
I then mesure the high to low times again, and if its more than 1 second, I know that the last rise to high time was my stop bit. I then do some math to calculate the bit rate knowing I have 9 total bits which include the start and stop bit.
I know i may have some obvious errors in my code, but my fundamental error is using CNT to store the rise and fall times. I know there is a better way to still maintain very accurate timings, and properly deal with the CNT roll.
(which i am not doing and thus why my code is Smile)
Pub Autobaud Repeat Repeat waitpeq(|< SerInput, |< SerInput, 0) 'wait for high riseatime := cnt waitpeq(0, |< SerInput, 0) 'wait for low (startbit) fallatime := cnt If fallatime - riseatime > 80000000 startbit := fallatime Quit Else riseatime := 0 fallatime := 0 Repeat waitpeq(|< SerInput, |< SerInput, 0) 'wait for high risebtime := cnt waitpeq(0, |< SerInput, 0) 'wait for low fallbtime := cnt If risebtime - fallbtime > 80000000 stopbit := risebtime Quit Else risebtime := 0 fallbtime := 0 frametime := stopbit - startbit bitrate := frametime / 9 abaudrate := bitrate * 80000000
Comments
That said, wouldn't a NEG counter based approach be easier?
Dude, you rawk, so i was just being a dummy with the details. I ALWAYS do this. I think its my trademark. lolz
I fixed it like you suggested and it works great.
THANKS!
(fair warning to others, the above corrected code is missing the first half(omitted due to no error?)
Not sure, I code by the seat of my pants most of the time. I learn as i go, so you may know better methods of autobaud, improving on it, etc, even doing it in assembly to allow much higher autobauding.
I am open to all suggestions.
38400 calculates to be 38406 sometimes and others 38443, and 38369.
The error range grows as baud rate grows.
If you need more accurate autobauding, do this in propasm.
These estimates are still very close, and you're safe to assume that the nearest standard baud rate to your estimate is what you've actually detected. It's not like there are that many of them.
I know this is a old horse ... however, I just had to 'fiddle' with it. I used timers to arrive at this solution. But it looks for a 'space-bar'. and I hard coded for a clock freq of 80MHz:
And this goes in its own cog with this:
I've tested it at all the Bauds shown and it works. Just had to throw that one in for fun.
... Tim