Demo: function pointer
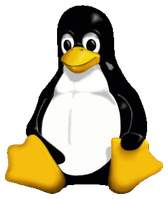
Hi,
one of C's strenght are function pointers.
Here a small demo for propgcc.
propeller-elf-gcc -o numdiff.elf -mlmm -Os -Wall main.c -lm
best regards,
Reinhard
one of C's strenght are function pointers.
Here a small demo for propgcc.
#include <stdio.h> #include <stdlib.h> // this is a new type, a pointer to a function typedef double (*function_pointer)(double x); /** * Differentiate any given Function at point x. * @param f: function to differentiate, thats a pointer to a function * @param x: double the argument where to differentiate * @return: double with the value of f'(x) */ // note there is one differentiate function !!! // we can call it, with any argument from type double ((*f)(double),double) double differentiate(double (*f)(double), double x) { double h = 0.0001; return (f(x+h)-f(x-h))/(2*h); } double xsquare(double x) // user defined function { return x*x; } double cube(double x) // user defined function { return x*x*x; } int main(int argc, char *argv[]) { double x; function_pointer fcp; // we make a variable from type function_pointer printf("differentiate the cube\n"); fcp = cube; // point to the function cube for(x = 0;x < 1; x+=0.1) { printf("x=%f x*x*x=%f, 3*x*x=%f, f'= %f\n", x, fcp(x), 3*x*x, differentiate(fcp,x)); } printf("\ndifferentiate the square\n"); fcp = xsquare; //point to the function xsquare for(x = 0;x < 1; x+=0.1) { printf("x=%f x*x=%f, 2*x=%f, f'= %f\n", x, fcp(x), 2*x, differentiate(fcp,x)); } return EXIT_SUCCESS; }
propeller-elf-gcc -o numdiff.elf -mlmm -Os -Wall main.c -lm
best regards,
Reinhard