Filename "globbing" with Catalina
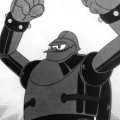
All,
I recently had a request about how to do filename globbing with Catalina. This is not supported by the DOSFS file system that Catalina uses (actually, it is not supported by any ANSI C compiler, since it is very OS-specific and not part of the ANSI C standard).
But it can be added easily enough.
I did a bit of hunting around the internet and created is a simple test program that demonstrates how to do it with Catalina. Unzip the attached file to a convenient place, and then compile the program with a command like the following (this command is for the C3):
The pattern matching algorithm (implemented in glob.c) supports DOS style wildcard characters like "?" and "*". The scanning function (implemented in storage.c) simply prints out any matches.
Here is an example of the output when I run the program on my Catalyst SD Card (on which I generally create a BIN subdirectory). The input I typed is shown in red (note <ENTER> means press the ENTER key!):
The listDir function could be modified to suit most applications that require globbing. The version provided needs some more work to be generally useful (e.g. it currently matches the raw FAT directory entry, which does not contain the "." between the name and extension, and also includes directories and not just files). Also, it should really be modified to use static variables so it can be called repeatedly to return all matching files one by one.
Ross.
I recently had a request about how to do filename globbing with Catalina. This is not supported by the DOSFS file system that Catalina uses (actually, it is not supported by any ANSI C compiler, since it is very OS-specific and not part of the ANSI C standard).
But it can be added easily enough.
I did a bit of hunting around the internet and created is a simple test program that demonstrates how to do it with Catalina. Unzip the attached file to a convenient place, and then compile the program with a command like the following (this command is for the C3):
catalina test.c storage.c glob.c -lcx -D SMALL -D C3 -D FLASH -D CACHED_1K -D PCWhen loaded and run, the test program asks you for a directory name, and a file name pattern to match, and scans the entries in the specified directory looking for filename matches.
The pattern matching algorithm (implemented in glob.c) supports DOS style wildcard characters like "?" and "*". The scanning function (implemented in storage.c) simply prints out any matches.
Here is an example of the output when I run the program on my Catalyst SD Card (on which I generally create a BIN subdirectory). The input I typed is shown in red (note <ENTER> means press the ENTER key!):
Press a key to start Mounting SD Card Volume label 'NO NAME ' Volume size 121 MB Filesystem: FAT16 Directory to search (null for root dir): [COLOR=red]<ENTER>[/COLOR] File name to match (wildcard expression):[COLOR=red]B*[/COLOR] Contents of directory '' entry: BIN Directory to search (null for root dir):BIN File name to match (wildcard expression):[COLOR=red]*[/COLOR] Contents of directory 'BIN' entry: . entry: .. entry: VI BIN entry: CATALYSTBIN entry: CP BIN entry: DBASIC BIN entry: LS BIN entry: LUA BIN entry: LUAC BIN entry: MKDIR BIN entry: MV BIN entry: RM BIN entry: RMDIR BIN entry: SST BIN Directory to search (null for root dir):[COLOR=red]BIN[/COLOR] File name to match (wildcard expression):[COLOR=red]??DIR*[/COLOR] Contents of directory 'BIN' entry: MKDIR BIN entry: RMDIR BINHere is the main function, to show how easy it is to use. For more detail, examine the files glob.c and storage.c:
/* * simple test of a file 'globbing' type function. * * NOTES: * * Seems to be case sensitive for file names, but not directory names! * * See glob.c for wildcard expression syntax. * * Filenames are simply 11 character strings - no attempt is made to insert * the "." before the extension (if any!) - this would need to be done * manually in the listDir function (which current just uses the raw * directory entry). * */ void main() { char dirname[256]; char pattern[256]; printf("Press a key to start\r\n"); k_wait(); printf("Mounting SD Card\r\n"); mountFatVolume(); while (1) { printf("Directory to search (null for root dir):"); gets(dirname); printf("\r\n"); printf("File name to match (wildcard expression):"); gets(pattern); printf("\r\n"); // list subdirectory 'dirname' entries that match 'pattern' listDir(dirname, pattern); } }The listDir function in storage.c (which does the work) uses the lower level DOSFS directory scanning functions rather than the higher level stdio functions (fopen etc). But once it finds a matching file name, you could simply return it and then open the file with that name using fopen.
The listDir function could be modified to suit most applications that require globbing. The version provided needs some more work to be generally useful (e.g. it currently matches the raw FAT directory entry, which does not contain the "." between the name and extension, and also includes directories and not just files). Also, it should really be modified to use static variables so it can be called repeatedly to return all matching files one by one.
Ross.