Print directly from the BS2
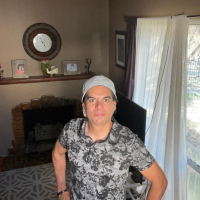
I will love to be able to use the Sparkfun COM-10438 thermal printer with the BS2 Stamp!
http://www.sparkfun.com/products/10438
It has been used with arduino but I don't know how to use C+ or whatever is that language. CAN ANYBODY OUT THERE TRANSLATE TO BASIC? I will reward!
Code:
' Sparkfun Thermal Printer Test (COM-10438)
#include
NewSoftSerial Thermal(2, 3); // printer RX to digital 2, printer TX to digital 3
int heatTime = 80;
int heatInterval = 255;
char printDensity = 15;
char printBreakTime = 15;
void setup()
{
Serial.begin(57600); // used for debugging via serial monitor
Thermal.begin(19200); // used for writing to the printer
initPrinter();
}
void initPrinter()
{
//Modify the print speed and heat
Thermal.print(27, BYTE);
Thermal.print(55, BYTE);
Thermal.print(7, BYTE); //Default 64 dots = 8*('7'+1)
Thermal.print(heatTime, BYTE); //Default 80 or 800us
Thermal.print(heatInterval, BYTE); //Default 2 or 20us
//Modify the print density and timeout
Thermal.print(18, BYTE);
Thermal.print(35, BYTE);
int printSetting = (printDensity<<4) | printBreakTime;
Thermal.print(printSetting, BYTE); //Combination of printDensity and printBreakTime
Serial.println();
Serial.println("Printer ready");
}
void loop()
{
Thermal.println("HELLOW WORLD!");
Thermal.print(10, BYTE); // Sends the LF to the printer, advances the paper
Thermal.print(" Millis = ");
Thermal.println(millis());
Thermal.print(10, BYTE);
Thermal.print(10, BYTE);
do { } while (1>0);
}
http://www.sparkfun.com/products/10438
It has been used with arduino but I don't know how to use C+ or whatever is that language. CAN ANYBODY OUT THERE TRANSLATE TO BASIC? I will reward!

Code:
' Sparkfun Thermal Printer Test (COM-10438)
#include
NewSoftSerial Thermal(2, 3); // printer RX to digital 2, printer TX to digital 3
int heatTime = 80;
int heatInterval = 255;
char printDensity = 15;
char printBreakTime = 15;
void setup()
{
Serial.begin(57600); // used for debugging via serial monitor
Thermal.begin(19200); // used for writing to the printer
initPrinter();
}
void initPrinter()
{
//Modify the print speed and heat
Thermal.print(27, BYTE);
Thermal.print(55, BYTE);
Thermal.print(7, BYTE); //Default 64 dots = 8*('7'+1)
Thermal.print(heatTime, BYTE); //Default 80 or 800us
Thermal.print(heatInterval, BYTE); //Default 2 or 20us
//Modify the print density and timeout
Thermal.print(18, BYTE);
Thermal.print(35, BYTE);
int printSetting = (printDensity<<4) | printBreakTime;
Thermal.print(printSetting, BYTE); //Combination of printDensity and printBreakTime
Serial.println();
Serial.println("Printer ready");
}
void loop()
{
Thermal.println("HELLOW WORLD!");
Thermal.print(10, BYTE); // Sends the LF to the printer, advances the paper
Thermal.print(" Millis = ");
Thermal.println(millis());
Thermal.print(10, BYTE);
Thermal.print(10, BYTE);
do { } while (1>0);
}
Comments
Duane
printerPin PIN 3
heatTime CON 80
heatInterval CON 255
printDensity CON 15
printBreakTime CON 15
To initialize the printer:
SEROUT printerPin, 32, [27, 55, 7, heatTime, heatInterval, 18, 35, (printDensity<<4) | printBreakTime ]
An example of an output:
SEROUT printerPin, 32, ["HELLO WORLD!", 10 ]
Note that the BS2 is not intended to work at 19200 Baud which is the normal Baud for this printer. It probably will work, but don't be surprised if there are occasional timing problems at this Baud. It's not at all clear how to change the printer to slower Bauds. The documentation from SparkFun doesn't mention it.
Be sure to look at the "What's a Microcontroller?" tutorial and the "Basic Stamp Syntax and Reference Manual", both downloadable from Parallax's Download webpage. The Stamp Manual shows how to use the SEROUT statement.
I've had one of these printers for a couple of months. Today is the first time I powered it up.
I wrote a small program for the Propeller to use the printer. I added the initialization bytes as Mike shows.
At first the printer wasn't printing well but I noticed I had the low current setting on my power supply. These little printers can suck a lot of juice. I wouldn't power it from your BS board directly (I don't know how much current it can supply). At 6.3V the max current was 1.054A when the printer was printing.
These a fun little printers. I'm glad you asked about it.
I couldn't find how to change the baud either.
Duane
Edit: The current at 5V was 0.994A. The current at 9V was 1.220A. The print quality was worse at 5V compared with 6.3V. The print quality a 6.3V and 9V looked the same to me. I used the settings in the program Luis posted.
http://www.parallax.com/Store/Microcontrollers/BASICStampModules/tabid/134/CategoryID/9/List/0/SortField/0/Level/a/ProductID/392/Default.aspx
But a Propeller still would be cheaper.
You said you couldn't find how to change the baud but works anyways?
QUOTE=Duane Degn;1034455]Luis,
I've had one of these printers for a couple of months. Today is the first time I powered it up.
I wrote a small program for the Propeller to use the printer. I added the initialization bytes as Mike shows.
At first the printer wasn't printing well but I noticed I had the low current setting on my power supply. These little printers can suck a lot of juice. I wouldn't power it from your BS board directly (I don't know how much current it can supply). At 6.3V the max current was 1.054A when the printer was printing.
These a fun little printers. I'm glad you asked about it.
I couldn't find how to change the baud either.
Duane
Edit: The current at 5V was 0.994A. The current at 9V was 1.220A. The print quality was worse at 5V compared with 6.3V. The print quality a 6.3V and 9V looked the same to me. I used the settings in the program Luis posted.[/QUOTE]
Here's the Propeller code I wrote.
After its "Hello World", it takes whatever is entered through the terminal window and sends it to the printer. Any information sent from the printer is displayed in the terminal window.
I haven't seen any information coming from the printer yet.
I found it interesting that the printer uses a 3.3V TTL interface. I thought it would be 5V.
Duane
Dave
I have been trying to make this printer work for weeks, it's been hit and miss.....
it will work for me if I use a 9V battery, but not if pluged in to the wall. is that crazy?