AS1106 Object
I am attempting to write an object for the AS1106 8 digit 7 segment driver. I think I got a good grasp on how it is all going to come together, but I am having a little (noob) problem on how to combine the register address and the data into one string for use with the SPI object from the Obex. Any suggestions?
Here is the SPI object I am using:
And the Data Sheet:
AS1106.pdf
Thank you to everyone.
>>~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~<<
>>(¯`·._.·(¯`·._.·(¯`·._.·(¯`·._. Schematics ._.·´¯)·._.·´¯)·._.·´¯)·._.·´¯)<<
>>~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~<<
┌────────┬───────┬────────┬───────┐
│ PIN │ SIG │ PIN │ SIG │
├────────┼───────┼────────┼───────┤ ┌──┐┌──┐┌──┐┌──┐┌──┐┌──┐┌──┐┌──┐
│ 1 │ DIN │ 13 │ CLK │ │8.├┤8.├┤8.├┤8.├┤8.├┤8.├┤8.├┤8.├──┐
├────────┼───────┼────────┼───────┤ └─┬┘└─┬┘└─┬┘└─┬┘└─┬┘└─┬┘└─┬┘└─┬┘ │
│ 2 │ DIG(0)│ 14 │ SEG(A)│ └───┻───┻───┻───┻───┻───┻───┻┐ │
├────────┼───────┼────────┼───────┤ │ │
│ 3 │ DIG(4)│ 15 │ SEG(F)│ VDD │ │
├────────┼───────┼────────┼───────┤  BUSS   BUSS
│ 4 │ GND │ 16 │ SEG(B)│ (R1*) │ │ │
├────────┼───────┼────────┼───────┤ ┌───────┫ │ │
│ 5 │ DIG(6)│ 17 │ SEG(G)│ │ ┌──────┻────────────────┐ │ │
├────────┼───────┼────────┼───────┤ │ │ DIG(0-7)┣──┘ │
│ 6 │ DIG(2)│ 18 │ Iset │ └──┫ISET(18) │ │
├────────┼───────┼────────┼───────┤ │ │ │
│ 7 │ DIG(3)│ 19 │ VDD │ SDAT───┫DIN(1) SEG(A-G)┣─────┘
├────────┼───────┼────────┼───────┤ │ SEG(DP)│
│ 8 │ DIG(7)│ 20 │ SEG(C)│ LD───┫LD/CSN(12) │
├────────┼───────┼────────┼───────┤ │ │
│ 9 │ GND │ 21 │ SEG(E)│ CLK───┫CLK(13) GND(4) GND(9)│
├────────┼───────┼────────┼───────┤ └─────────────┳──────┳──┘
│ 10 │ DIG(5)│ 22 │SEG(DP)│ │ │
├────────┼───────┼────────┼───────┤ └──────┫
│ 11 │ DIG(1 │ 23 │ SEG(D)│ │
├────────┼───────┼────────┼───────┤ 
│ 12 │ LD/CSN│ 24 │ DOUT │ GND
└────────┴───────┴────────┴───────┘
* R1 suggested values found on page 13. For 3.3 volt supply, reference table 19.
}}
CON
'' Clock settings
_CLKMODE = XTAL1 +PLL16X
_XINFREQ = 5_000_000
VAR
Byte Reg, Data, FDAT
Long Load, Clock, SerDat
OBJ
SPI : "SPI_ASM"
PUB init(LD, CLK, SDat)
'' init display
Load := LD
Clock := CLK
SerDat := SDat
dira[Load] := 1
outa[Load] := 0
SPI.start(15,0) '' Initialize SPI Engine with Clock Delay of 15 and Clock State of 1
PUB Digit(DigitNo)
'' Set AS1106 for number of digits connected to the driver IC. DigNo MUST be from 1 to 8,
'' since only one chip is supported at this time
Reg := DigitNo
Data := 0
Out(Reg, Data)
DigitNo := DigitNo - 1
Scan(DigitNo)
PUB Decode(mode)
{{ Full-featured Decoding modes. Find Binary value for the displays to be decoded, then
covert to DEC value for individual digit decoding options. 1 = decode, 0 = no decode
for each display. Mode 0-255 valid
┌────────┬────────┬────────────────────────────────────────────────────────────┐
│ Mode │ HEX │ Feature │
├────────┼────────┼────────────────────────────────────────────────────────────┤
│ 0 │ $00 │ No Decode for digits 7:0 │
├────────┼────────┼────────────────────────────────────────────────────────────┤
│ 1 │ $01 │ Code-B/HEX decode for digit 0. No Decode for Digits 7:1 │
├────────┼────────┼────────────────────────────────────────────────────────────┤
│ 15 │ $0F │ Code-B/HEX decode for digit 3:0. No Decode for Digits 7:4│
├────────┼────────┼────────────────────────────────────────────────────────────┤
│ 255 │ $FF │ Code-B/HEX decode for all digits │
└────────┴────────┴────────────────────────────────────────────────────────────┘ }}
Reg := ($09)
Data := Mode
Out(Reg, Data)
PUB Intense(int)
'' Digital adjustment of disply intensity. 0 - 15 is Valid)
Reg := ($0A)
Data := int
Out(Reg, Data)
PUB ShutDown(Mode)
{{ Fully-featured shut-down modes.
┌────────┬──────────────────────────────────────────────┐
│ Mode │ Features │
├────────┼──────────────────────────────────────────────┤
│ 0 │ Shut-Down. Reset feature register to default.│
├────────┼──────────────────────────────────────────────┤
│ 1 │ Shut-Down. Feature register unchanged. │
├────────┼──────────────────────────────────────────────┤
│ 2 │ Normal Operation. Feature register reset. │
├────────┼──────────────────────────────────────────────┤
│ 3 │ Normal Operation. Feature register unchanged.│
└────────┴──────────────────────────────────────────────┘}}
if Mode := 0
Data := ($00)
if Mode := 1
Data := ($80)
if Mode := 2
Data := ($01)
if Mode := 3
Data := ($81)
Reg := ($0C)
Out(Reg, Data)
PUB SelfTest
PUB Out(RegAddy, ModeData)
'' Output to AS1106 <===not completed===>
outa[Load] := 1
SPI.SHIFTOUT(SerDat, Clock, SPI#MSBFIRST , 16, RegAddy, ModeData) '' Request Configuration Write
{{
>>~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~<<
>>(¯`·._.·(¯`·._.·(¯`·._.·(¯`·._. Features ._.·´¯)·._.·´¯)·._.·´¯)·._.·´¯)<<
>>~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~<<
Upon init of your code, set all of the Funk variables to chosen value (1 or 0),
then you can update on the fly throughout your code.
┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐
│D15│D14│D13│D12│D11│D10│D09│D08│D07│D06│D05│D04│D03│D02│D01│D00│
├───┼───┼───┼───┼───┴───┴───┴───┼───┴───┴───┴───┴───┴───┴───┴───┤
│ X │ X │ X │ X │RegisterAddress│ Data │
└───┴───┴───┴───┴───────────────┴───────────────────────────────┘
Feature Bits (Data section):
D00 = Clock Enable
D01 = Register Reset
D02 = Decode Select
D03 = SPI Enable
D04 = Blink Enable
D05 = Blink Frequency
D06 = Sync
D07 = Blink Start
For more detailed explanation of feature set, please see page 12 of the data sheet.
}}
PUB Funk(clken, RegRes, DecodeSel, SPIen, Blinken, BlinkFreq, Sync, BlinkStart)
'' clken : Clock Settings 0 = Internal Oscillator *
'' 1 = CLK pin acts as clock
If clken := (%1)
FDat := FDat + 1
'' RegRes : Feature reset 0 = Normal Operation *
'' 1 = Reset control registers (except feature resister)
If regres := (%1)
FDat := FDat + 2
'' DecodeSel: Display Encoding 0 = Code B Decoding *
'' 1 = Enable HEX Decoding
If regres := (%1)
FDat := FDat + 4
'' SPIen: SPI interface 0 = Disable
'' 1 = Enable
If SPIen := (%1)
FDat := FDat + 8
'' BlinkEn: blink 0 = disable blinking
'' 1 = enable blinking
if blinken := (%1)
FDAT := FDAT + 16
'' BlinkFreq: Blink Frequency 0 = 1 sec per cycle (fast)
'' 1 = 2 sec per cycle (slow)
if BlinkFreq := (%1)
FDAT := FDAT + 32
'' sync: Syncs Blink with LD rise 0 = No sync
'' 1 = Sync'd
if sync := (%1)
FDAT := FDAT + 64
'' BlinkStart: How blinking starts 0 = Starts off
'' 1 = Starts on
if blinkstart := (%1)
FDAT := FDAT + 128
Reg := ($0E)
Data := FDat
Out(Reg, Data)
PRI Scan(Disp)
'' Scan Limit settings for digit number to be displayed. Should not be used for blanking
'' because of possible differences in brghtness.
Reg := ($0B)
Data := Disp
Out(Reg, Data)
PRI NoOp
'' Sends a NO-OP code to the device. This is used when only cascading multiple devices
'' together. See page 12 of the data sheet for more information. <===not completed===>
Here is the SPI object I am using:
{{
************************************************
* Propeller SPI Engine v1.2 *
* Author: Beau Schwabe *
* Copyright (c) 2009 Parallax *
* See end of file for terms of use. *
************************************************
Revision History:
V1.0 - original program
V1.1 - fixed problem with SHIFTOUT MSBFIRST option
- fixed argument allocation in the SPI Engines main loop
V1.2 - Added Clock delay option and fixed bug in SHIFTIN function
}}
CON
#0,MSBPRE,LSBPRE,MSBPOST,LSBPOST '' Used for SHIFTIN routines
''    
'' =0 =1 =2 =3
''
'' MSBPRE - Most Significant Bit first ; data is valid before the clock
'' LSBPRE - Least Significant Bit first ; data is valid before the clock
'' MSBPOST - Most Significant Bit first ; data is valid after the clock
'' LSBPOST - Least Significant Bit first ; data is valid after the clock
#4,LSBFIRST,MSBFIRST '' Used for SHIFTOUT routines
''  
'' =4 =5
''
'' LSBFIRST - Least Significant Bit first ; data is valid after the clock
'' MSBFIRST - Most Significant Bit first ; data is valid after the clock
#1,_SHIFTOUT,_SHIFTIN '' Used for operation Mode
''  
'' =1 =2
VAR
long cog, command
PUB SHIFTOUT(Dpin, Cpin, Mode, Bits, Value) ''If SHIFTOUT is called with 'Bits' set to Zero, then the COG will shut
''down. Another way to shut the COG down is to call 'stop' from Spin.
setcommand(_SHIFTOUT, @Dpin)
PUB SHIFTIN(Dpin, Cpin, Mode, Bits)|Value,Flag ''If SHIFTIN is called with 'Bits' set to Zero, then the COG will shut
''down. Another way to shut the COG down is to call 'stop' from Spin.
Flag := 1 ''Set Flag
setcommand(_SHIFTIN, @Dpin)
repeat until Flag == 0 ''Wait for Flag to clear ... data is ready
Result := Value
'------------------------------------------------------------------------------------------------------------------------------
PUB start(Delay,State) : okay
{{
Delay := 15 ''Clock delay
'' = 300ns + (N-1) * 50ns
'' Example1:
'' A Delay of 5 would be 500ns
'' 300ns + 4 * 50ns = 500ns
'' Example2:
'' A Delay of 15 would be 1us
'' 300ns + 14 * 50ns = 1000ns = 1us
State := 1 '' 0 - Start Clock LOW
'' 1 - Start Clock HIGH
}}
'' Start SPI Engine - starts a cog
'' returns false if no cog available
stop
ClockDelay := Delay
ClockState := State
okay := cog := cognew(@loop, @command) + 1
PUB stop
'' Stop SPI Engine - frees a cog
if cog
cogstop(cog~ - 1)
command~
PRI setcommand(cmd, argptr)
command := cmd << 16 + argptr ''write command and pointer
repeat while command ''wait for command to be cleared, signifying receipt
'################################################################################################################
DAT org
'
'' SPI Engine - main loop
'
loop rdlong t1,par wz ''wait for command
if_z jmp #loop
movd :arg,#arg0 ''get 5 arguments ; arg0 to arg4
mov t2,t1 '' │
mov t3,#5 ''───┘
:arg rdlong arg0,t2
add :arg,d0
add t2,#4
djnz t3,#:arg
mov address,t1 ''preserve address location for passing
''variables back to Spin language.
wrlong zero,par ''zero command to signify command received
ror t1,#16+2 ''lookup command address
add t1,#jumps
movs :table,t1
rol t1,#2
shl t1,#3
:table mov t2,0
shr t2,t1
and t2,#$FF
jmp t2 ''jump to command
jumps byte 0 ''0
byte SHIFTOUT_ ''1
byte SHIFTIN_ ''2
byte NotUsed_ ''3
NotUsed_ jmp #loop
'################################################################################################################
'tested OK
SHIFTOUT_ ''SHIFTOUT Entry
mov t4, arg3 wz '' Load number of data bits
if_z jmp #Done '' '0' number of Bits = Done
mov t1, #1 wz '' Configure DataPin
shl t1, arg0
muxz outa, t1 '' PreSet DataPin LOW
muxnz dira, t1 '' Set DataPin to an OUTPUT
mov t2, #1 wz '' Configure ClockPin
shl t2, arg1 '' Set Mask
test ClockState, #1 wc '' Determine Starting State
if_nc muxz outa, t2 '' PreSet ClockPin LOW
if_c muxnz outa, t2 '' PreSet ClockPin HIGH
muxnz dira, t2 '' Set ClockPin to an OUTPUT
sub _LSBFIRST, arg2 wz,nr '' Detect LSBFIRST mode for SHIFTOUT
if_z jmp #LSBFIRST_
sub _MSBFIRST, arg2 wz,nr '' Detect MSBFIRST mode for SHIFTOUT
if_z jmp #MSBFIRST_
jmp #loop '' Go wait for next command
'------------------------------------------------------------------------------------------------------------------------------
SHIFTIN_ ''SHIFTIN Entry
mov t4, arg3 wz '' Load number of data bits
if_z jmp #Done '' '0' number of Bits = Done
mov t1, #1 wz '' Configure DataPin
shl t1, arg0
muxz dira, t1 '' Set DataPin to an INPUT
mov t2, #1 wz '' Configure ClockPin
shl t2, arg1 '' Set Mask
test ClockState, #1 wc '' Determine Starting State
if_nc muxz outa, t2 '' PreSet ClockPin LOW
if_c muxnz outa, t2 '' PreSet ClockPin HIGH
muxnz dira, t2 '' Set ClockPin to an OUTPUT
sub _MSBPRE, arg2 wz,nr '' Detect MSBPRE mode for SHIFTIN
if_z jmp #MSBPRE_
sub _LSBPRE, arg2 wz,nr '' Detect LSBPRE mode for SHIFTIN
if_z jmp #LSBPRE_
sub _MSBPOST, arg2 wz,nr '' Detect MSBPOST mode for SHIFTIN
if_z jmp #MSBPOST_
sub _LSBPOST, arg2 wz,nr '' Detect LSBPOST mode for SHIFTIN
if_z jmp #LSBPOST_
jmp #loop '' Go wait for next command
'------------------------------------------------------------------------------------------------------------------------------
MSBPRE_ '' Receive Data MSBPRE
MSBPRE_Sin test t1, ina wc '' Read Data Bit into 'C' flag
rcl t3, #1 '' rotate "C" flag into return value
call #PreClock '' Send clock pulse
djnz t4, #MSBPRE_Sin '' Decrement t4 ; jump if not Zero
jmp #Update_SHIFTIN '' Pass received data to SHIFTIN receive variable
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
LSBPRE_ '' Receive Data LSBPRE
add t4, #1
LSBPRE_Sin test t1, ina wc '' Read Data Bit into 'C' flag
rcr t3, #1 '' rotate "C" flag into return value
call #PreClock '' Send clock pulse
djnz t4, #LSBPRE_Sin '' Decrement t4 ; jump if not Zero
mov t4, #32 '' For LSB shift data right 32 - #Bits when done
sub t4, arg3
shr t3, t4
jmp #Update_SHIFTIN '' Pass received data to SHIFTIN receive variable
'------------------------------------------------------------------------------------------------------------------------------
MSBPOST_ '' Receive Data MSBPOST
MSBPOST_Sin call #PostClock '' Send clock pulse
test t1, ina wc '' Read Data Bit into 'C' flag
rcl t3, #1 '' rotate "C" flag into return value
djnz t4, #MSBPOST_Sin '' Decrement t4 ; jump if not Zero
jmp #Update_SHIFTIN '' Pass received data to SHIFTIN receive variable
'------------------------------------------------------------------------------------------------------------------------------
LSBPOST_ '' Receive Data LSBPOST
add t4, #1
LSBPOST_Sin call #PostClock '' Send clock pulse
test t1, ina wc '' Read Data Bit into 'C' flag
rcr t3, #1 '' rotate "C" flag into return value
djnz t4, #LSBPOST_Sin '' Decrement t4 ; jump if not Zero
mov t4, #32 '' For LSB shift data right 32 - #Bits when done
sub t4, arg3
shr t3, t4
jmp #Update_SHIFTIN '' Pass received data to SHIFTIN receive variable
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
LSBFIRST_ '' Send Data LSBFIRST
mov t3, arg4 '' Load t3 with DataValue
LSB_Sout test t3, #1 wc '' Test LSB of DataValue
muxc outa, t1 '' Set DataBit HIGH or LOW
shr t3, #1 '' Prepare for next DataBit
call #PostClock '' Send clock pulse
djnz t4, #LSB_Sout '' Decrement t4 ; jump if not Zero
mov t3, #0 wz '' Force DataBit LOW
muxnz outa, t1
jmp #loop '' Go wait for next command
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
MSBFIRST_ '' Send Data MSBFIRST
mov t3, arg4 '' Load t3 with DataValue
mov t5, #%1 '' Create MSB mask ; load t5 with "1"
shl t5, arg3 '' Shift "1" N number of bits to the left.
shr t5, #1 '' Shifting the number of bits left actually puts
'' us one more place to the left than we want. To
'' compensate we'll shift one position right.
MSB_Sout test t3, t5 wc '' Test MSB of DataValue
muxc outa, t1 '' Set DataBit HIGH or LOW
shr t5, #1 '' Prepare for next DataBit
call #PostClock '' Send clock pulse
djnz t4, #MSB_Sout '' Decrement t4 ; jump if not Zero
mov t3, #0 wz '' Force DataBit LOW
muxnz outa, t1
jmp #loop '' Go wait for next command
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
Update_SHIFTIN
mov t1, address '' Write data back to Arg4
add t1, #16 '' Arg0 = #0 ; Arg1 = #4 ; Arg2 = #8 ; Arg3 = #12 ; Arg4 = #16
wrlong t3, t1
add t1, #4 '' Point t1 to Flag ... Arg4 + #4
wrlong zero, t1 '' Clear Flag ... indicates SHIFTIN data is ready
jmp #loop '' Go wait for next command
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
PreClock
mov t2, #0 nr '' Clock Pin
test t2, ina wz '' Read ClockPin
muxz outa, t2 '' Set ClockPin to opposite of read value
call #ClkDly
muxnz outa, t2 '' Restore ClockPin to original read value
call #ClkDly
PreClock_ret ret '' return
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
PostClock
mov t2, #0 nr '' Clock Pin
test t2, ina wz '' Read ClockPin
call #ClkDly
muxz outa, t2 '' Set ClockPin to opposite of read value
call #ClkDly
muxnz outa, t2 '' Restore ClockPin to original read value
PostClock_ret ret '' return
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
ClkDly
mov t6, ClockDelay
ClkPause djnz t6, #ClkPause
ClkDly_ret ret
'------------------------------------------------------------------------------------------------------------------------------
'tested OK
Done '' Shut COG down
mov t2, #0 '' Preset temp variable to Zero
mov t1, par '' Read the address of the first perimeter
add t1, #4 '' Add offset for the second perimeter ; The 'Flag' variable
wrlong t2, t1 '' Reset the 'Flag' variable to Zero
CogID t1 '' Read CogID
COGSTOP t1 '' Stop this Cog!
'------------------------------------------------------------------------------------------------------------------------------
{
########################### Assembly variables ###########################
}
zero long 0 ''constants
d0 long $200
_MSBPRE long $0 '' Applies to SHIFTIN
_LSBPRE long $1 '' Applies to SHIFTIN
_MSBPOST long $2 '' Applies to SHIFTIN
_LSBPOST long $3 '' Applies to SHIFTIN
_LSBFIRST long $4 '' Applies to SHIFTOUT
_MSBFIRST long $5 '' Applies to SHIFTOUT
ClockDelay long 0
ClockState long 0
''temp variables
t1 long 0 '' Used for DataPin mask and COG shutdown
t2 long 0 '' Used for CLockPin mask and COG shutdown
t3 long 0 '' Used to hold DataValue SHIFTIN/SHIFTOUT
t4 long 0 '' Used to hold # of Bits
t5 long 0 '' Used for temporary data mask
t6 long 0 '' Used for Clock Delay
address long 0 '' Used to hold return address of first Argument passed
arg0 long 0 ''arguments passed to/from high-level Spin
arg1 long 0
arg2 long 0
arg3 long 0
arg4 long 0
{{
┌──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┐
│ TERMS OF USE: MIT License │
├──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┤
│Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation │
│files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, │
│modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software│
│is furnished to do so, subject to the following conditions: │
│ │
│The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.│
│ │
│THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE │
│WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR │
│COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, │
│ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. │
└──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────┘
}}
And the Data Sheet:
AS1106.pdf
Thank you to everyone.
pdf
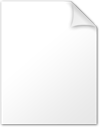
698K
Comments
No You are not an idiot!
I was going to give you a complete answer yesterday, But I was busy on other things,
1) Are you planning on using this device for (a) Matrix LED or (b) 7 Segemnt LED?
2) I use a simlar device the AS1108, Which is the same as the AS1106 that you are using except it only does 4 Segents ( or 32 LEDS)
3) I have complete code for the AS1108 for driving a 4 & 8 LED Matrix. I am not farmiliar with coding for a 7-digit Segment display but I am sure its not that dificult.
4) Another tip, This device I believe is exactly the same as MAX7219 LED Driver, there is plenty of info on the web about this, but the Austria mIcro systems chips are about half the price of MAXIM.
Firstly, You don't "Really" need any PASM code as the "Driver" , a SPIN driver will do nicely, You can use any of the SHIFT-OUT Objects/routines , but the only thing you need to manage is an addtional bit of code to toggle to LOAD Pin at the end.
This is my Shift Out Routine specifically made for the AS1108 Device..
PRI ShiftOut ( ByteValue ) DIRA [DataPin] := Output 'SET the DATA Pin to OUTPUT OUTA [DataPin] := Low 'TOGGLE the DATA Pin OFF DIRA [ClockPin] := Output 'SET the CLOCK Pin to OUTPUT OUTA [ClockPin] := Low 'TOGGLE the CLOCK Pin OFF DIRA [LoadPin] := Output 'SET the LOAD Pin to OUTPUT OUTA [LoadPin] := High 'TOGGLE the LOAD Pin On WAITCNT(1_500 + CNT) 'Wait OUTA [LoadPin] := Low 'SEt the LOAD Pin OFF REPEAT Bits 'Start REPEAT LOOP for vNoOfBits ie 16 for the AS1108 OUTA [DataPin] := ByteValue >> (Bits-1) 'SET the DATA pin to the first bit ByteValue := ByteValue << 1 'SHIFT the BYTEVALUE to the Next Bit OUTA [ClockPin] := High 'TOGGLE the CLOCK Pin ON OUTA [ClockPin] := Low 'TOGGLE the CLOCK Pin OFF WAITCNT(1_500 + CNT) 'WAIT for some time and REPEAT OUTA [LoadPin] := High 'SET the LOAD Pin On WAITCNT(1_500 + CNT) 'Wait OUTA [LoadPin] := Low 'SEt the LOAD Pin Off
You will need to "Set up" or "Init" the device first with a few calls but generally you just send a 16bit "word" to the device with the same shiftout routine. for example.
ShiftOut ( %0000_1100_1000_0001 ) 'SHUTDOWN MODE = NORMAL OPERATION ShiftOut ( %0000_1011_0000_0011 ) 'SCAN LIMIT -SET NONE ShiftOut ( %0000_0001_0000_0000 + LedRow1 )
A good tip is to create your own spin OBJECT and call it "AS1106.spin", and put all you code there, including the PRI SHIFTOUT method.
You will need in this object the following "Code" blocks ( for example)
CON Input = 0 , Output = 1, Low = 0, High = 1, Off = 0, On = 1, Bits = 16 VAR PUB Init PUB TestOn PUB TestOff PUB UpdateLeds PRI ShiftOut
If I get some time I can post my complete code, but as I said I only have this for driving individual leds in a 4 x 8 Matrix. keep in mind this device can be a little bit tricky.
Dave M
The initial idea was to have a counter that could go up into the millions for large-scale stress testing, so I was thinking 7 segments for starters, and maybe making some kind of crazy display for a project I have going at home re-building an arcade cabinet, using a matrix display for the marquis. But thisis once I get a better understanding, and the ground work laid for the controller, but it is not at all a requirement at this phase.
When shopping the distro's, I saw the 7219, and really liked it, other than the price. That is what prompted me to look for alternatives. Guess I did not dive into the spec sheet for the 7219 deep enough to see how similar they really are.
The SPI driver I took from the Obex as a quick way to try and get this thing to do what I want it to. I think I remember seeing the shift in/out routines in the BS2 object. I hear it is a bit slower to do it that way, but I guess looking in on it, it would not make too much of a difference to pull those out than it is using the SPI object. I have done a bit of work on the BS2, so maybe getting to something a little familiar would help hammer some concepts home.
Anyhow.. thank you for the info. I'll build up a circuit today if I have a chance to get out of a few meetings and get back to you.
In the init part you are setting dira and ina for the spi pins. Probably the spi object is already setting them, so it might be a problem.
Massimo
code samples right out of the department of redundancy department. Thanks much for the pointer, and I'll fix that up when I can hack back into it. (hopefully today)
Regarding my ShiftOut routine, I set the pin numbers in the INIT code block, When the SHIFTOUT is called it will set the direction and state at the time of use. The reason I do this is that I may have more that one use on a PCB for the SHIFT OUT, so the pins could be different each time. This means that the SHIFTOUT Routine is GENERIC, but this one of mine is made SPECIFICALLY for the AS1108 ( 6) devices. so I suggest to use these, Have a good look at my code comments, its all there!
Thanks
Dave M