Simple example of two way communication with the XBee and Propeller
Hey all,
I had a customer walk in with his project in hand. He was having some trouble getting bi-directional communication between two XBee connected Propeller boards. Once I double checked his wiring and XBee settings, I wrote this little example program to simply demonstrate the concept of client->server->client communication.
The basic run down of what these two programs do...
Host Server gets loaded with the server code.
Remote Client gets loaded with the client code.
Client transmits a prompt for validation to the server.
Server validates the received prompt. Server then transmits a validated reply to the Client.
Client waits for received response from server. If received response is valid, LED turns on. If recieved response is invalid, LED turns off.

--Real simple demonstration. I thought it might be useful for some of you who want to get started with the XBee modules.
I had a customer walk in with his project in hand. He was having some trouble getting bi-directional communication between two XBee connected Propeller boards. Once I double checked his wiring and XBee settings, I wrote this little example program to simply demonstrate the concept of client->server->client communication.
The basic run down of what these two programs do...
Host Server gets loaded with the server code.
Remote Client gets loaded with the client code.
Client transmits a prompt for validation to the server.
Server validates the received prompt. Server then transmits a validated reply to the Client.
Client waits for received response from server. If received response is valid, LED turns on. If recieved response is invalid, LED turns off.
--Real simple demonstration. I thought it might be useful for some of you who want to get started with the XBee modules.
spin
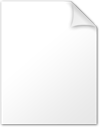
967B
Comments
Neat. If this is to serve as general demo code, maybe add a comment on the BAUD_RATE setting, that if using Series 1 XBees and wanting high speed, to set BAUD_RATE to 111_111 and not the nominal 115_200?
Is there a known problem with the XBee where it needs to communicate at 111_111 baud? I haven't heard of that before.
As far as I have used them, you can totally communicate at 115_200 baud. The XBee just has to be configured to expect communication at that speed...
Bearing in mind that we're only talking about the series 1 XBees here, the issue is that the internal clock runs at 16MHz. That gets divided by a configurable integer value and then by 16 to drive the UART, so you can get any baud rate that's a factor of 1MHz. If you want 115,200 baud the nearest divisor is 9, which actually delivers 111,111 baud. The difference is enough to make communication unreliable in many cases. Series 2 XBees have a faster clock, and don't suffer from the problem.
For more detail, you might like to check out my XBee series 1 cookbook (and other resources - FAQ and API packet check program) at http://www.jsjf.demon.co.uk/xbee/xbee.html . The cookbook has an appendix on baud rates. It also has the stub of a second appendix on the issue, but that's a work in progress and currently on hold.
i am trying to use the demo code on 2 demo boards with 2 xbees thansk for the exmaple ou have been the most help so far. but i am haveing some issues i have the dmeo boards so i changed the pin for the led to my demo boards led "20" but the code does not seem to work. also i changed the dout and din ping to 1,2
In your client code you have LED_PIN set to 19 (not 20 as you said) and when you try to toggle the LED you're driving pin 16. Would that account for it?
i made the chages to use the LED_PIN varable but still nothing lights up on the demo board.
Have you verified that your XBees are configured to communicate with each other? That was one of the biggest stumbling blocks I ran into when learning how to use XBees.
Some of the settings to verify are the PAN ID, the Channel, and if Destination High and Destination Low are set. If you got XBee 802.14.5 adapters from Parallax, they come from Digi International preprogrammed with defaults to set the XBees to just broadcast messages - meaning that any XBee with the default values will receive a message sent by any other XBee with the default values. If you picked up the ZB (Series 2) modules, then you will have to do some pre-configuration to get them talking nicely with each other.
If you're still stuck, would you like to post again with a few extra details?
1. You can use X-CTU to save a .PRO file containing (most of) the XBee settings. Do that for each of the XBees and attach the files to the post (eg host.pro, client.pro). I have a nifty little program that can analyse these things if you're using the Series 1 model.
On the other hand, if you're using series 2, I may have to bow out at this point.
2. Attach also your current SPIN code for client and host.
My default settings are:
PAN ID: 3332
Channel: C
Destination High and Destination Low: leave those blank or clear them.
Also, disable AES Encryption if its on, but you would have had to set that up.
Any luck with it?
Can these codes be modified in any way to interface the parallax propeller with MICAz motes...?