TSL1401 and Arduino
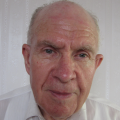
re Line-Scan Camera TSL1401-DB and DB to 12 pin Expander Board and Arduino Duemilanove Mother Board (http://arduino.cc/en/Main/ArduinoBoardDuemilanove).
I am trying to write Arduino C code to operate the TSL1401-DB in one-shot imaging.
Could an expert in TSL1401-DB programming review my timing in the function "getcamera" below? I would be grateful for your advice.
int syncPin = 12;
int clockPin = 11;
int dataPin = 2; // must be in range 0 to 5
int lightVal[128];
unsigned long utime;
int itime = 0;
int expose = 7390; // 8333uSec ie 1/120th second
void setup()
{
pinMode(clockPin, OUTPUT);
pinMode(syncPin, OUTPUT);
Serial.begin(9600);
}
void loop()
{
int b = Serial.read();
if (b == 's')
{
getCamera();
delay(2000);
}
}
void getCamera()
{
digitalWrite(clockPin, LOW);
pinMode(syncPin, OUTPUT);
digitalWrite(syncPin, HIGH);
digitalWrite(clockPin, HIGH);
digitalWrite(syncPin, LOW);
utime = micros();
digitalWrite(clockPin, LOW);
for (int j = 0; j < 128; j++)
{
digitalWrite(clockPin, HIGH);
digitalWrite(clockPin, LOW);
}
delayMicroseconds(expose);
digitalWrite(syncPin, HIGH);
digitalWrite(clockPin, HIGH);
digitalWrite(syncPin, LOW);
utime = micros() - utime;
digitalWrite(clockPin, LOW);
for (int j = 0; j < 128; j++)
{
delayMicroseconds(20);
lightVal[j] = analogRead(dataPin);
digitalWrite(clockPin, HIGH);
digitalWrite(clockPin, LOW);
}
delayMicroseconds(20);
for (int j = 0; j < 128; j++)
{
itoa(lightVal[j], 'S');
}
itime = (int)utime;
itoa(itime, 'S');
}
Regards,
Kevin
I am trying to write Arduino C code to operate the TSL1401-DB in one-shot imaging.
Could an expert in TSL1401-DB programming review my timing in the function "getcamera" below? I would be grateful for your advice.
int syncPin = 12;
int clockPin = 11;
int dataPin = 2; // must be in range 0 to 5
int lightVal[128];
unsigned long utime;
int itime = 0;
int expose = 7390; // 8333uSec ie 1/120th second
void setup()
{
pinMode(clockPin, OUTPUT);
pinMode(syncPin, OUTPUT);
Serial.begin(9600);
}
void loop()
{
int b = Serial.read();
if (b == 's')
{
getCamera();
delay(2000);
}
}
void getCamera()
{
digitalWrite(clockPin, LOW);
pinMode(syncPin, OUTPUT);
digitalWrite(syncPin, HIGH);
digitalWrite(clockPin, HIGH);
digitalWrite(syncPin, LOW);
utime = micros();
digitalWrite(clockPin, LOW);
for (int j = 0; j < 128; j++)
{
digitalWrite(clockPin, HIGH);
digitalWrite(clockPin, LOW);
}
delayMicroseconds(expose);
digitalWrite(syncPin, HIGH);
digitalWrite(clockPin, HIGH);
digitalWrite(syncPin, LOW);
utime = micros() - utime;
digitalWrite(clockPin, LOW);
for (int j = 0; j < 128; j++)
{
delayMicroseconds(20);
lightVal[j] = analogRead(dataPin);
digitalWrite(clockPin, HIGH);
digitalWrite(clockPin, LOW);
}
delayMicroseconds(20);
for (int j = 0; j < 128; j++)
{
itoa(lightVal[j], 'S');
}
itime = (int)utime;
itoa(itime, 'S');
}
Regards,
Kevin
Comments
I have written a code in c, but I have a true doubts how to connect sensor to my arduino.
I have written datasheet and there is no literal explanation how to connect exactply nodes as:
tr, sd, st, so
My question is where to find this? And I have some interesting code for you, but I have to check it so I won't paste it there yet.
Please help me and if the code works, i will give it to you.
thanks
Piter
Here is the breadboard -
There are 2 64 pixel arrays, so AO1 connects to AO2, and SO1 connects to SI2 to trigger the second array. I've simplified the code to only display the pixel values on a single line in the serial monitor. Once I have that working, I can move on to the more interesting stuff. I also made analog versions of getPix and dispPix so I can see something other than 0 or 1. So far with the digital input all I get are 0 with my Arduino Micro, or 1 with an Uno. With analog inputs, I get 95 from AO2. If I wire the sensor to only use the first 64 pixel array and take only AO1, I get 0.
I changed clockSI slightly, so SI goes low before the clock goes high. There was a footnote in the datasheet saying that was necessary. Also I left the clock high, because the first bit comes through with the first rising clock after SI. If I take the clock low during clockSI I will miss that first bit.
It didn't make any difference, I can't get this code to work any more than the original. I have two of these sensor, and neither of them worked. I know it's not the 1401, but I don't see anything different about its operation other than the need for the pulldown resistor.
I have the same issue with a TSL201R, which is 64 pixel array in one only, both doesn't work, I have 1 everywhere...
Have you found a solution ?