Inter-Process communication
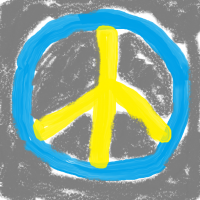
I like to present a method I now use for some time to synchronize processes, running in parallel on different cogs.
One of the reasons is, that calling a method may need a lot of parameters to pass and that is sometimes uncomfortable.
The idea behind that is: start a cog by loading a spin or asm method and have the method initialized, then running idle. Sending a command to the method triggers an action, which may be running ones or persistant.
Communication runs via a process control block, like PCB_IEnc for an incremental encoder.
First I declare a constant NmbIEncPar, which is the number of parameters, passed to the process. Then, there is a table of NmbIEncPar pointers to the actual variables in global memories.
Then there is an enumeration of the process commands:
Now, as the main routine starts, the process has to be initialized:
Now the incremental encoder is running and the position will be in IEncPos.
As a special feature: it may happen, that the resolution of the encoder is not fixed. If unknown at the start (hardware could be plugged in), then the default value of resolution is set to 0. If this is true, then the incremental encoder first has to pass the index signal twice running into the same direction, so resolution is known, stored to IEncRes and the programm run continues.
If the resolution is known, than the initial position is set to a value more than twice the resolution. When the encoder reaches index, the position is set to the correct value.
To be continued
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away
One of the reasons is, that calling a method may need a lot of parameters to pass and that is sometimes uncomfortable.
The idea behind that is: start a cog by loading a spin or asm method and have the method initialized, then running idle. Sending a command to the method triggers an action, which may be running ones or persistant.
Communication runs via a process control block, like PCB_IEnc for an incremental encoder.
CON NmbIEncPar = 12 'Number of parameters, handed over to the incremental encoder process Dat PCB_IEnc long @IEncCmd, @IEncPos, @IEncOfs, @IEncRes, @IEncVTim, @IEncVCnt, @IEncTiSl, @IEncSens long @IEncTiBa, @ScVal053, @ScVal054, @ScVal055
First I declare a constant NmbIEncPar, which is the number of parameters, passed to the process. Then, there is a table of NmbIEncPar pointers to the actual variables in global memories.
Then there is an enumeration of the process commands:
'CommandSet of incremental encoder process #1, Idle, Run, FreeRun, DetReso, LoadPar, Sweep
Now, as the main routine starts, the process has to be initialized:
IEncCmd := NmbIEncPar ' Tell the process how many pointers to initialize i := IEnc.start(@PCB_IEnc,@@0)-1 ' Start incremental encoder process ifIEncRes ==0 ActState := CmdInvok (@IEncCmd, DetReso, i) Returns if resolution of encoder detected, stays in 'FreeRun' else ActState := CmdInvok (@IEncCmd, Run, 255) 'Run the incremental encoder in triggered mode (to be explained)
Now the incremental encoder is running and the position will be in IEncPos.
As a special feature: it may happen, that the resolution of the encoder is not fixed. If unknown at the start (hardware could be plugged in), then the default value of resolution is set to 0. If this is true, then the incremental encoder first has to pass the index signal twice running into the same direction, so resolution is known, stored to IEncRes and the programm run continues.
If the resolution is known, than the initial position is set to a value more than twice the resolution. When the encoder reaches index, the position is set to the correct value.
To be continued
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away

Comments
This code is nearly independ from starting a Spin or an ASM process.
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away
And I was so proud to have a piece of self modifying code!
Thank you for this hint. By the way: this asm module realizes an quadrature encoder with speed measurement. Will come in parts after I return from holidays
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away
more to follow
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
cmapspublic3.ihmc.us:80/servlet/SBReadResourceServlet?rid=1181572927203_421963583_5511&partName=htmltext
Hello Rest Of The World
Hello Debris
Install a propeller and blow them away
This post came up on my recient post about memory so I thought I would give it a read. The problem I am having is that the snippets are confusing me. Is it possible you could post or PM me a file that shows the complete process from the top method thru to the assembly obj. I think I am seeing a pointer to a series of pointers that is being fed to the new cog.
RS_Jim