help me check my code, from keypad to lcd
' {$STAMP BS2} ' {$PBASIC 2.5} ' ========== Program Description ========== ' This program demonstrates using a Hitachi-compatible Parallel LCD Display ' to interface with a 4 x 4 keypad, outputing it to the LCD display ' This code works with the BS2, and is in the language of pbasic. ' ========== I/O Definitions ========== 'RW gnd ' R/W Pin For LCD E PIN 0 ' Enable Pin For LCD RS PIN 1 ' LCD Register Select LDR1 PIN 2 LDR2 PIN 3 TX1 PIN 4 TX2 PIN 5 SEL_KP PIN 6 SEL_LCD PIN 7 KP_IN1 PIN 8 KP_IN2 PIN 9 KP_IN3 PIN 10 KP_IN4 PIN 11 KP_LCD1 PIN 12 KP_LCD2 PIN 13 KP_LCD3 PIN 14 KP_LCD4 PIN 15 ' ========== Variables ========== char VAR Byte ' Character To Send To LCD inst VAR char ' Induction To Send To LCD index VAR Word ' Character Pointer temp VAR Byte ' Temp Variable cnt VAR Byte db VAR Bit ' Debounce bit for use by keyScan. press VAR Bit ' Flag to indicate keypress. key VAR Nib ' Key number 0-15. keychar VAR CHAR row VAR Nib ' Counter used in scanning keys. cols VAR INC ' Input states of pins P8-P11. ' ========== EEPROM Data ========== DATA "Mobile Number" ' Message To Send To LCD ' ========== Initialization ========== Initialize: OUTS = %0000000000000000 ' Set All Output Low DIRS = %1111000011110011 ' Set I/O Direction GOSUB Init_Lcd ' Initialize The LCD Display ' ========== Program Code ========== Main: FOR temp = 0 TO 31 ' 32 = 2 x 16 Characters IF temp = 15 THEN ' Check For End Of Line GOSUB Next_Line ' Jump To Next Line ENDIF READ temp, char ' Read Next Character From EEPROM GOSUB Send_Text ' Send Character To LCD Display NEXT HIGH SEL_LCD 'select LCD PAUSE 100 LOW SEL_KP PAUSE 100 LOW SEL_LCD 'select LCD PAUSE 100 HIGH SEL_KP PAUSE 100 LOW SEL_LCD 'select LCD PAUSE 100 HIGH RS PAUSE 100 OUTD = %0011 ' Send High Nibble PULSOUT E,1 PAUSE 10 OUTD = %0011 ' Send Low Nibble PULSOUT E,1 HIGH SEL_LCD 'select LCD PAUSE 100 keypad1: GOSUB keyScan IF press = 0 THEN keypad1 DEBUG "key pressed = ", HEX key,CR ' LOW SEL_LCD 'select LCD ' PAUSE 100 ' LOW RS ' Inst = %00000001 ' Reset The LCD ' GOSUB Send_Inst 'DEBUG "lcd cleared" 'HIGH RS 'keychar=keychar+$30 'keychar.LOWNIB = key GOSUB Send_Num PAUSE 50 press = 0 FOR cnt = 1 TO 7 keypad2: GOSUB keyScan HIGH SEL_KP PAUSE 100 IF press = 0 THEN keypad2 DEBUG "key pressed = ",HEX key,CR 'keychar = key+$30 LOW SEL_LCD 'select LCD PAUSE 100 HIGH RS 'keychar.HIGHNIB = %0011 'keychar.LOWNIB = key GOSUB Send_Num PAUSE 50 press = 0 NEXT GOTO Main END '========== Subroutines ========== Init_Lcd: LOW SEL_LCD 'select LCD PAUSE 100 OUTH = %00000001 ' Reset The LCD PULSOUT E,1 PAUSE 10 OUTH = %00101000 ' Set To 4-bit Operation PULSOUT E,1 Inst = %00001110 ' Turn On Cursor GOSUB Send_Inst Inst = %00000110 ' Set Auto-Increment GOSUB Send_Inst Inst = %00000001 ' Clears LCD GOSUB Send_Inst HIGH SEL_LCD 'select LCD PAUSE 100 RETURN Send_Inst: LOW SEL_LCD 'select LCD PAUSE 100 LOW RS ' Set Instruction Mode OUTD = Inst.HIGHNIB ' Send High Nibble PULSOUT E,1 OUTD = Inst.LOWNIB ' Send Low Nibble PULSOUT E,1 HIGH RS ' Set LCD Back To Text Mode HIGH SEL_LCD 'select LCD PAUSE 100 RETURN Send_Text: LOW SEL_LCD 'select LCD PAUSE 100 OUTD = Char.HIGHNIB ' Send High Nibble PULSOUT E,1 PAUSE 10 OUTD = char.LOWNIB ' Send Low Nibble PULSOUT E,1 HIGH SEL_LCD 'select LCD PAUSE 100 RETURN Next_Line: Inst = 128+64 ' Move Cursor To Line 2 GOSUB Send_Inst RETURN Send_Num: LOW SEL_LCD 'select LCD PAUSE 100 OUTD = $3 ' Send High Nibble PULSOUT E,1 PAUSE 10 OUTD = keychar.LOWNIB ' Send Low Nibble PULSOUT E,1 HIGH SEL_LCD 'select LCD PAUSE 100 RETURN keyScan: LOW SEL_KP PAUSE 100 FOR row = 12 TO 15 ' Scan rows one at a time. LOW row ' Output a 0 on current row. 'OUTH = row 'PAUSE 5 key = ~cols ' Get the inverted state of column bits. key = NCD key ' Convert to bit # + 1 with NCD. IF key <> 0 THEN HIGH SEL_KP PAUSE 100 LOW SEL_LCD 'select LCD PAUSE 100 HIGH RS PAUSE 100 OUTD = %0000 ' Send High Nibble PULSOUT E,1 PAUSE 10 OUTD = %0000 ' Send Low Nibble PULSOUT E,1 PAUSE 10 HIGH SEL_LCD 'select LCD PAUSE 100 LOW SEL_KP PAUSE 100 IF db = 1 THEN done ' Already responded to this press, so done. db = 1: press = 1 ' Set debounce and keypress flags. key = (key-1)+(row*4) ' Add column (0-3) to row x 4 (0,4,8,12). ' Key now contains 0-15, mapped to this arrangement: ' 0 1 2 3 ' 4 5 6 7 ' 8 9 10 11 ' 12 13 14 15 ' A lookup table is translates this to match the actual ' markings on the key caps. LOOKUP key,[noparse][[/noparse]1,2,3,10,4,5,6,11,7,8,9,12,14,0,15,13],key done: INPUT row ' Disconnect output on row. HIGH SEL_KP PAUSE 100 RETURN ENDIF ' push ' No high on cols? No key pressed. INPUT row ' Disconnect output on row. NEXT ' Try the next row. db = 0 ' Reset the debounce bit. HIGH SEL_KP PAUSE 100 RETURN ' Return to program. ' push: ' IF db = 1 THEN done ' Already responded to this press, so done. ' db = 1: press = 1 ' Set debounce and keypress flags. ' key = (key-1)+(row*4) ' Add column (0-3) to row x 4 (0,4,8,12). ' Key now contains 0-15, mapped to this arrangement: ' 0 1 2 3 ' 4 5 6 7 ' 8 9 10 11 ' 12 13 14 15 ' A lookup table is translates this to match the actual ' markings on the key caps. ' LOOKUP key,[noparse][[/noparse]1,2,3,10,4,5,6,11,7,8,9,12,14,0,15,13],key ' done: ' INPUT row ' Disconnect output on row. 'HIGH SEL_KP 'PAUSE 100 ' RETURN ' Return to program.
it cant seems to work ):
im using a SN54LS244J, its attached
pdf
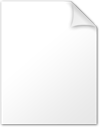
198K