Help with Programing code for BS2 using PPI 8255 for controlling LCD
' {$STAMP BS2}
' {$PBASIC 2.5}
' ======= PINs =======
E_LCD PIN 1
Reset_8255 PIN 2
A1_8255 PIN 3
A0_8255 PIN 4
WR_8255 PIN 5
RD_8255 PIN 6
CS_8255 PIN 7
DB0_8255 PIN 8
DB1_8255 PIN 9
DB2_8255 PIN 10
DB3_8255 PIN 11
DB4_8255 PIN 12
DB5_8255 PIN 13
DB6_8255 PIN 14
DB7_8255 PIN 15
' ======= Initialization =======
OUTS = $00 ' Set Outputs Low
DIRS = $FF ' Set I/O Direction
GOSUB Init_8255 'Initialize PPI 8255
GOSUB LCD_Init ' Initialize The LCD Display
' ======= Main Code ========
Main:
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %01000001 'Set databus for function set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDdata
PAUSE 100
GOTO Main
'======= Subroutines =======
Init_8255: 'Initialize 8255 to All Outputs at Active Low state
OUTL = $FF 'Set all the control lines (P0-P7) High (Inactive)
PAUSE 50 'Settling time for a few ms (50 ms)
LOW Reset_8255 '8255 is Reset (Active)
HIGH WR_8255 'Set Write control High (Inactive)
LOW RD_8255 'Set Read control Low (Active)
HIGH A1_8255 'Set Address control A1 & A0 High to access the control word register
HIGH A0_8255
OUTH = $81 'Set databus (P8-P15) to $81 for MODE 0 - Port A & B ports as outputs and C as half half
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 50 'Pulse Write control Low (Active) for 100 us (duration not critical)
HIGH CS_8255 'Set Chip Select High (Inactive) - All outputs are set to Active Low
RETURN
LCD_Init: 'Set up 8255 Port A for Output
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00111000 'Set databus for function set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
GOSUB writeLCDcmd
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00001000 'Set databus for display off
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00000001 'Set databus for clear display
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00000110 'Set databus for entry mode set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00001110 'Set databus for turn display and cursor on
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
RETURN
writeLCDcmd:
LOW A1_8255 'Set address control A1 & A0 to set the 8255 Port B for Output
HIGH A0_8255 'take note of the reverse from the orignal code
OUTH = %00000000 'Set databus for pattern2
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
PAUSE 50
PULSOUT E_LCD,1
PAUSE 100
OUTH = %01100000 'Set databus for back high
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
RETURN
writeLCDdata:
LOW A1_8255 'Set address control A1 & A0 to set the 8255 Port B for Output
HIGH A0_8255 'take note of the reverse from the orignal code
OUTH = %00100000 'Set databus for informatio displayed to LCD
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
PAUSE 50
PULSOUT E_LCD,1
PAUSE 100
OUTH = %01100000 'Set databus for back high
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
RETURN
sorry im pretty new to this BS2
im using BS2 to control the 8255 to output to the LCD
but it seems to not work.
i have attached the 8255 datasheet.
can any one please help me?
' {$PBASIC 2.5}
' ======= PINs =======
E_LCD PIN 1
Reset_8255 PIN 2
A1_8255 PIN 3
A0_8255 PIN 4
WR_8255 PIN 5
RD_8255 PIN 6
CS_8255 PIN 7
DB0_8255 PIN 8
DB1_8255 PIN 9
DB2_8255 PIN 10
DB3_8255 PIN 11
DB4_8255 PIN 12
DB5_8255 PIN 13
DB6_8255 PIN 14
DB7_8255 PIN 15
' ======= Initialization =======
OUTS = $00 ' Set Outputs Low
DIRS = $FF ' Set I/O Direction
GOSUB Init_8255 'Initialize PPI 8255
GOSUB LCD_Init ' Initialize The LCD Display
' ======= Main Code ========
Main:
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %01000001 'Set databus for function set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDdata
PAUSE 100
GOTO Main
'======= Subroutines =======
Init_8255: 'Initialize 8255 to All Outputs at Active Low state
OUTL = $FF 'Set all the control lines (P0-P7) High (Inactive)
PAUSE 50 'Settling time for a few ms (50 ms)
LOW Reset_8255 '8255 is Reset (Active)
HIGH WR_8255 'Set Write control High (Inactive)
LOW RD_8255 'Set Read control Low (Active)
HIGH A1_8255 'Set Address control A1 & A0 High to access the control word register
HIGH A0_8255
OUTH = $81 'Set databus (P8-P15) to $81 for MODE 0 - Port A & B ports as outputs and C as half half
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 50 'Pulse Write control Low (Active) for 100 us (duration not critical)
HIGH CS_8255 'Set Chip Select High (Inactive) - All outputs are set to Active Low
RETURN
LCD_Init: 'Set up 8255 Port A for Output
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00111000 'Set databus for function set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
GOSUB writeLCDcmd
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00001000 'Set databus for display off
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00000001 'Set databus for clear display
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00000110 'Set databus for entry mode set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00001110 'Set databus for turn display and cursor on
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
RETURN
writeLCDcmd:
LOW A1_8255 'Set address control A1 & A0 to set the 8255 Port B for Output
HIGH A0_8255 'take note of the reverse from the orignal code
OUTH = %00000000 'Set databus for pattern2
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
PAUSE 50
PULSOUT E_LCD,1
PAUSE 100
OUTH = %01100000 'Set databus for back high
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
RETURN
writeLCDdata:
LOW A1_8255 'Set address control A1 & A0 to set the 8255 Port B for Output
HIGH A0_8255 'take note of the reverse from the orignal code
OUTH = %00100000 'Set databus for informatio displayed to LCD
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
PAUSE 50
PULSOUT E_LCD,1
PAUSE 100
OUTH = %01100000 'Set databus for back high
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255
RETURN
sorry im pretty new to this BS2
im using BS2 to control the 8255 to output to the LCD
but it seems to not work.
i have attached the 8255 datasheet.
can any one please help me?
pdf
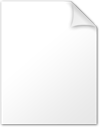
367K
Comments
The 8255 was meant as a parallel port expander and will use a lot of Stamp pins. Is there something different about your LCD that a serial LCD and 1 Stamp pin couldn't do?
Cheers,
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
Tom Sisk
http://www.siskconsult.com
·
this is my LCD (: can some one please help?
2. Why are you using the 8255? Planning to control more I/O pins that the stamp has?
3. If your LCD is Hitachi compatible it will do internal reset on power up and you should be able to adjust the contrast to see the LCD matrix without running any programs.
4. You can save 4 I/O pins running 4- bit operatios.There are sample codes on this site.
5. I do not see any LCD initialization, I may have missed it, in your code. You need to do that to set LCD operation - data bus width, number of lines, solid or blinking characters etc. (See Hitachi HD4470 spec)
3. i will be using a keypad to output to the LCD
4. i will be needing it as a 8bit
5. my code for initialization
LCD_Init: 'Set up 8255 Port A for Output
LOW A1_8255 'Set address control A1 & A0 Low to set the 8255 Port A for Output
LOW A0_8255
OUTH = %00111000 'Set databus for function set
HIGH RD_8255 'Set Read control high (inactive)
LOW WR_8255 'Set Write control Low (active)
LOW CS_8255 'Set Chip Select Low (Active)
PULSOUT WR_8255, 5 'Pulse Write control Low (Active) for 10 us (port changes state)
HIGH CS_8255 'Set Chip Select High (Inactive) - Disable the 8255 databus
GOSUB writeLCDcmd
GOSUB writeLCDcmd
GOSUB writeLCDcmd
Can you see any matrix characters on you LCD when you just apply power?
Just noticed that you are setting all the data to port A of 8255 but writing to LCD using port B.
You should add some DEBUG's to·your code. Aslo some of·the 8255 code could be in subroutines to make you code more readible.
Post Edited (vaclav_sal) : 9/23/2009 5:07:47 AM GMT
the port A will be used as the Databus of the LCD and the Port B as control bus of port B
this is my design =/
im pretty lousy with programming, so help would be greatly appreciated ! (: