Looking for ideas for identifying the start of a serial input into Prop
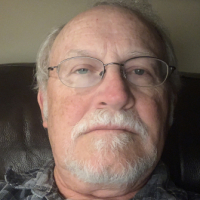
Before I start coding I want to come up with a process for downloading serial data into a prop so I don't end up going down too many blind alleys.
What I have is a serial source (a seperate PIC processor performing 10-bit ADC). I will be sending 3 seperate ADC result sets (numbers ranging from 0 to 1023) at 1 second increments. I am trying to figure out is the best way to transfer the data and have the prop be able to properly identify which one of the 3 sets of data it is recieving and then store the value in the appropriate global value. I have one cog exclusively devoted to recieving the data into the variables where the other cogs can read the value as needed. I wrote some spin code for recieving and storing a single value but need to come up with some ideas for recognizing multiple items. I thought I'd ask if anyone else has run across an elegant·solution in their travels?
Since I am only concerned with the ADC numbers, I thought to first send a character like 'a' followed by comma, first number, comma, second number, comma, third number. (ex.: a,40,523, 1023). Then I could use the 'a' to key as the leadin in spin to determine where my location in the serial stream. That should be fairly easy to code at the PIC and in spin.
If anyone has a better idea I would love to hear it! (examples are nice too if you can point me to existing code!)
Bob Sweeney
What I have is a serial source (a seperate PIC processor performing 10-bit ADC). I will be sending 3 seperate ADC result sets (numbers ranging from 0 to 1023) at 1 second increments. I am trying to figure out is the best way to transfer the data and have the prop be able to properly identify which one of the 3 sets of data it is recieving and then store the value in the appropriate global value. I have one cog exclusively devoted to recieving the data into the variables where the other cogs can read the value as needed. I wrote some spin code for recieving and storing a single value but need to come up with some ideas for recognizing multiple items. I thought I'd ask if anyone else has run across an elegant·solution in their travels?
Since I am only concerned with the ADC numbers, I thought to first send a character like 'a' followed by comma, first number, comma, second number, comma, third number. (ex.: a,40,523, 1023). Then I could use the 'a' to key as the leadin in spin to determine where my location in the serial stream. That should be fairly easy to code at the PIC and in spin.
If anyone has a better idea I would love to hear it! (examples are nice too if you can point me to existing code!)
Bob Sweeney
Comments
-Phil
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
'Just a few PropSTICK Kit bare PCBs left!
Andy
Post Edited (TChapman) : 12/14/2008 4:52:50 AM GMT
Bob Sweeney
( my code is buried away somewhere but I'll dig it up if someone wants to try it )
( you should be able to do the same thing with the standard RS-232 function as well... if one "Master" chip controls who can talk when )
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
" Anything worth doing... is worth overdoing. "
··············································· ( R.A.H. )
····································
Of all the ideas presented here (including my own), I like Ariba's the most.
-Phil
▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔▔
'Just a few PropSTICK Kit bare PCBs left!