Lissajous Curve Generator
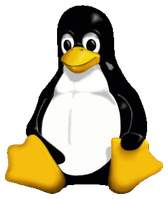
;-)
For everyone who has to work hard in an electronics laboratory and for whom the boss constantly looks over his shoulder.
Load the program onto the P2 and your Osci looks very busy.
For everyone who has to work hard in an electronics laboratory and for whom the boss constantly looks over his shoulder.
Load the program onto the P2 and your Osci looks very busy.
/* * Lissajou Curve on 2 Channel osci * connect probes with P0 and P2 -- 100mV/Div -- AC * set osci mode to XY */ #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <propeller.h> #define P2_TARGET_MHZ 160 #include "sys/p2es_clock.h" #define L_PIN 0 #define R_PIN 2 #define SYSTEM_CLOCK (_CLOCKFREQ) #define SAMPLE_RATE 16000 #define DAC_MODE (3 << 1) // DAC 16-bit dither, PWM #define DIR_MODE 1 // Output enabled, overrides DIR #define INIT_8BIT_DAC_VALUE 128 #define ANALOG_OUT_MODE 0x17 // 75 ohm, 2.0V DAC mode #define SMARTPIN_DAC_MODE (ANALOG_OUT_MODE << 16) | (INIT_8BIT_DAC_VALUE << 8) | (DIR_MODE << 6) | DAC_MODE #define SAMPLE_PERIOD SYSTEM_CLOCK / SAMPLE_RATE // CPU cycles between sample updates //////////////////////////////////////////////////////////////////////// void audio_init() { __asm { wrpin ##SMARTPIN_DAC_MODE, #L_PIN // Config smartpin DAC mode on "left" pin wrpin ##SMARTPIN_DAC_MODE, #R_PIN // Config smartpin DAC mode on "right" pin wxpin ##SAMPLE_PERIOD, #L_PIN // Set sample period for left audio channel wxpin ##SAMPLE_PERIOD, #R_PIN // Set sample period for right audio channel dirh #L_PIN // Enable smartpin DAC mode on left pin dirh #R_PIN // Enable smartpin DAC mode on right pin setse1 #(1 << 6) | L_PIN // Event triggered every new sample period (when "left in pin rises") }; } //////////////////////////////////////////////////////////////////////// void main () { int phi = 0; int s1,s2; unsigned int alpha,tmp; int frq = 800 * 268435; clkset(_SETFREQ, _CLOCKFREQ); audio_init(); while(1) { alpha += 0x5000; if (alpha > 0x7FFFFFFF) tmp = phi - alpha; else tmp = phi + alpha; if (getcnt() > 0x7FFF) //curve changed every 10 sec tmp *= 2; __asm { qrotate ##$1000,phi getqy s1 qrotate ##$1000,tmp getqy s2 xor s1, ##$8000 xor s2, ##$8000 wypin s1, #L_PIN wypin s2, #R_PIN waitse1 add phi,frq }; }//end while }//end main
Comments
1.) I find the math behind very interest.
2.) It's a test case for switch/case and getchar statements.
3.) demonstrate the communication between cog and hub in C.
run with:
Is this second piece of code Propeller GCC or Catalina? I've obviously been napping. Had no idea C was so well along on the P2.
sorry I forget this info.
This is fastspin on the command line. Easy to use ;-)