Simpleide simpletools problem?
in Propeller 1
Up until recently I have been using libfdserial and libsimpletext without any problems. I am using a program that that uploads data via serial to my Raspberry Pi. On the SimpleIDE side the program runs as expected, but on the Raspberry Pi side,using Python, I have to use decode() on the incoming data. This is where the problem lies, ..."can't decode byte 0xea in position 0"... Not sure what is causing this problem? Any help will be appreciated.
Thanks
Ray
Comments
Doing some more investigation, using the P2 and implementing jm_fullduplexserial.spin2, the problem with the Python decode() went away. I am guessing that the Simpleide C version of FullDuplexSerial function has something that the decode() can not deal with. I wonder what would be the best way to get around this would be. Since Simpleide has gone quiet, as far as updating is concerned, I am not holding my breath.
If FlexC was able to do some WiFi, like handling and creating a WEB page, I would probably change over to FlexC, but I am not holding my breath on this one either. Everything seems to be so scattered and on usable.
Ray
Since I don't know what the program on P1 is doing it's hard to determine what is going on.
I looked through the code in fdserial and there is nothing in there that prevents it from sending 0xea.
The P2 does not work like the P1 so the code to send data is nothing like the P1.
To test this out I wrote this program:
On the receiving side I used XCTL to capture the serial data:
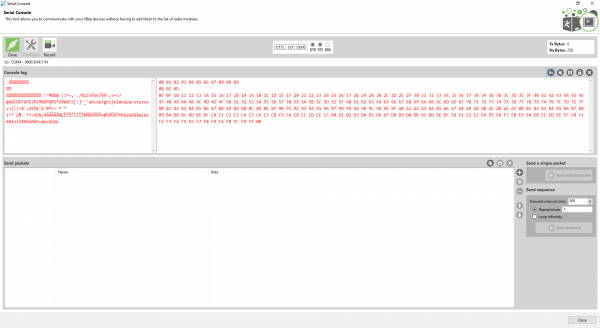
You can see all the data came through with no issues from P1 to my computer.
Sending binary data through serial is a bad idea.
Also, you don't need a full duplex driver to send data as the data can only be transmitted at the rate you are sending it at. You need a full duplex driver to receive data if the program will be off doing something else when the data arrives.
Mike
Below is the Simpleide program, code is kind of messy, but you can get an idea of what is going on. This program is also working a WEB page, when I look at the C program part, I try to think of how I can simplify it. I added a part where I am sending data to the P2, to see if the work around is working.
Ray
Below is the Python program that I am uploading the data to. It will get a lot more complicated than what it looks like right now.
Ray
Some time ago I tried to do serial to my RPi and had issues where sometimes random characters where being received.
I see that you are using a Wifi module and wondering if it might be simpler to send the serial data through the Wifi module over your network to the RPi.
MIke
I was also thinking along those lines at some point, but I could not figure out how to do it. I am not that well versed in javascript and Sockets. When I looked into doing javascript tasks, that really boggled my brain. Plus, I am not sure about the WiFi module and how big of a program it could hold. The other thing that I am now thinking about is, is there a limitation with the Simpleide WiFi function as it pertains to using javascript.
The other problem that started to occur, with the WiFi module is that something is changing the IP address of the WiFi module. Not sure if it is the TP-Link router or something else. I tried to see if I could lock in the IP address via the WiFi module setup, but I did not see how it could be done.
Ray
Below is the html program that I am using with the WiFi module. Even this is getting to be a complicated mess.
Ray
Did you try and run Putty on RPi to see what the data looks like coming from the P1?
Mike
Yes I ran Putty and serial port terminal and what I got was not what I was sending. Just got some weird characters. Now I am wondering if it is Python on the rpi that is the culprit. I do not have any way of putting a win 10 machine in place of the rpi. This is starting to get complicated just to find the fault.
Ray
Well, I broke out my Raspberry Pi 3 and hooked up a few wires to the serial interface and ran this program:
On the Pi side I used putty since that what I know and after setting the baud rate to 115200 got the following screen shot:
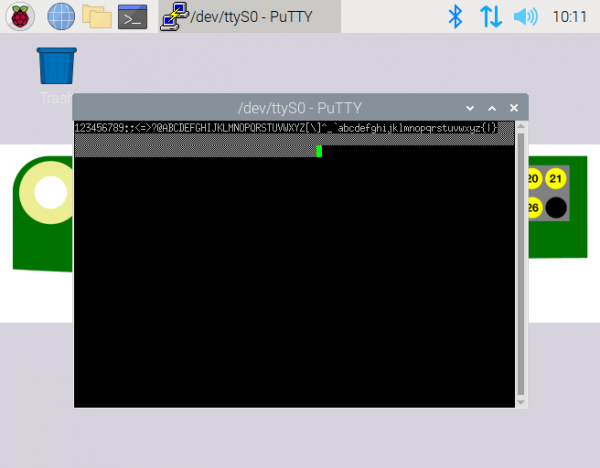
Serial seems to work just fine.
Also a about a year ago I wrote this program using Visual Studio:
That also worked as well.
Mike
Thanks Mike for looking into some of this stuff. I created a work around for the time being.
The Activity WX board is gathering some data and maintaining the WEB page. It is also uploading some data to the P2 Edge. This is using C.
The P2 Edge is uploading the data to the rpi 4. This is using FlexBasic.
The rpi 4 is developing the data that it has received. This is using Python.
So, is it possible that somewhere I will introduce some bugs. I am still thinking about involving the three micro.bit boards that I have, into this system. Not sure how that will be done. This is using using microPython. Still thinking about having a private network, that is not available to the internet. So, how do I simplify all of this.
Ray
Maybe you should turn things around.
From the RPi 4 you can make a connection to the WiFi board using telent and just send commands to the P1 which would then return the answers.
No serial would be needed to do that.
The RPi 4 should be able to do a web page or start a webserver that can process the requests.
This would be easy to test from the RPi4 using putty at first and then build an app from that.
Mike