VGA - Demo: Beauty of fractals
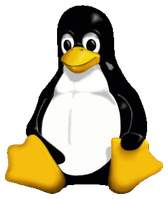
in Propeller 2
This demo is for vga, connect the A/V Board to pin 48.
Alternating 2 known fractals are calculated and drawn in real time.
Have fun!
Reinhard
Alternating 2 known fractals are calculated and drawn in real time.
Have fun!
Reinhard
zip
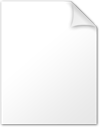
273K
Comments
In monochrome you could go higher resolutions too. 1024x768 or up to 1920x1080 might look even more detailed...
I think it would be good to get a graphics library working soon, ideally one that works with both HUB based and external memory frame buffers with HyperRAM which is now imminent too. It would be handy if it was done in SPIN2 and then could work for both FastSPIN and official PNUT based SPIN2.
One benefit of using it in Fastspin would be the graphics library itself could grow to be quite substantial with heaps of useful drawing functions but it would only actually take up image space if the routines were called. Without dead code removal, using this library in Chip's SPIN2 version could mean it unfortunately grows rather large, unless @cgracey plans to ultimately support dead code removal. If he doesn't want that then it might then need to be broken into different smaller modules somehow, which can get a bit more tedious to manage and doesn't buy as much code savings unless every method is its own object.
I do a few cosmetic changes in sierpinsky.c
Thank you for the modified version with high resolution, I named it fractals.c
I hope to find time soon to do more experiments with higher resolution.
best regards
Reinhard
By the way, all examples are derived from BASIC programs (goto
From the books
Fractals for the Classroom Part1 & 2 (1992) by Peitgen,Jürgens and Saupe
It would be nice to make the images fill the entire screen space too. I guess the code was probably designed for lower resolution initially but scaling it up could look nicer.
You could have just compiled the BASIC programs with fastspin