Select Case problem
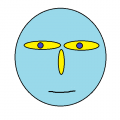
in BASIC Stamp
Does anyone know how to use Debug/Select/Case commands using words and sentences from keyboard ? Appreciate the
help.
help.
Comments
left 108*2+ 101*3+ 102*5+ 116*7 =1841
right 114*2+ 105*3+ 103*5+ 104*7+ 116*11 =3062
up 117*2+ 112*3 =570
down 100*2+ 111*3+ 119*5+ 110*7 =1898
By the "chinese remainder theorem", the values computed will be unique. Subsequently when you input chars from the keyboard after the prompt, sum up with the same math until you get a CR, then look for a numerical match.
LOOKUP x,[2,3,5,7,9,11,13],y
sum = Dbyte * y + sum
For a small set of key words it could work by simply adding up the ascii codes, if it turns out that the sums are unique anyway.
sum = Dbyte + sum
Edit: Tracy, I sent you a PM regarding this thread. EndEdit
The method or one like it does works though for a small set of words, just so long as the words map to unique numerical values.
Sometimes you don't see the forest for the trees.